Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial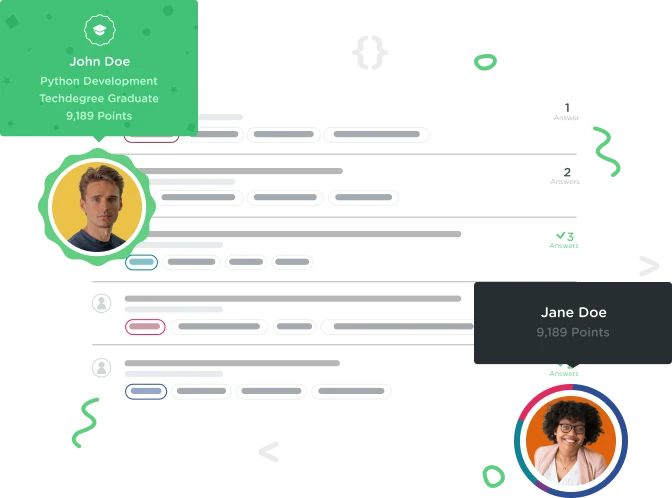

Weston Ross
832 PointsI've declared boolean outside of the scope. How do I apply it to more than one answer, or to a true and a false answer?
Provided that I have followed directions accurately (unlikely, this is my first time coding), I am attempting to solve this problem. I made the boolean re print out the given question if the answer to the question was "no". I could see how you could use an else statement if it was any answer other than "no", but how do you add more than one boolean if the answer to the question was "yes". Thank you for your help.
// I have initialized a java.io.Console for you. It is in a variable named console.
String response;
boolean wrongAnswer;
boolean rightAnswer;
do {
response = console.readLine("Do you understand do while loops? ");
wrongAnswer = (response.equalsIgnoreCase("no"));
if (wrongAnswer){
console.printf("do you understand do while loops? ");
}
rightAnswer = (response.equalsIgnorCase("yes"));
if (rightAnswer){
console.printf("Because you said yes, you passed the test!");
}
}while (wrongAnswer);
2 Answers
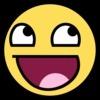
Michael Hess
24,512 PointsHi Weston,
This is one way to complete this challenge:
//Declare a String variable named response, then initialize it using double quotes
String response ="";
// The do while loop keeps asking the same question until "yes" is entered
//So we want to keep repeating when "no" is typed
// Exit the loop when "yes" is entered
do{
response = console.readLine("Do you understand do while loops?"); // "yes" or "no" is stored in the response variable
// when someone types "no" the loop repeats
// when someone types "yes" the loop is exited
}while(response.equalsIgnoreCase("no"));
//After the loop exits, the following line prints to the console
console.printf("Because you said %s, you passed the test!",response); //task 3
** if, else if, else statements, and booleans aren't needed to complete this challenge ** This exercise is focusing on the do-while loop.
Hope this helps! If this isn't making sense we're here to help! Ask any lingering questions you may have!
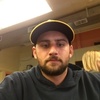
Miles Rakestraw
204 PointsIf I am understanding your question correctly, you would need to add an "else if".
So your code would look something like this:
public static void main(String [] args){
Console console = System.console();
String response;
boolean wrongAnswer;
boolean rightAnswer;
do {
response = console.readLine("Do you understand do while loops? ");
wrongAnswer = (response.equalsIgnoreCase("no"));
rightAnswer = (response.equalsIgnoreCase("yes"));
//this is your code to check if "wrongAnswer" is true.
if (wrongAnswer){
System.out.printf("do you understand do while loops? ");
}
//this is also your code to check if "rightAnswer" is true.
else if(rightAnswer){
System.out.printf("Because you said yes, you passed the test!");
}
//this else would handle any input that was not "yes" or "no"
// i.e. an input of "dog"
else {
System.out.printf("Not an answer");
}
//here you would have to change your conditional to encompass any answer that is not "rightAnswer"
}while (!rightAnswer);
}
Sorry Markdown didn't display the code chuck right.
I added some comments into the code segment briefly explaining what each piece does. I hope this answers your question. If any of this doesn't make sense I'll try my best to explain in more detail.