Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial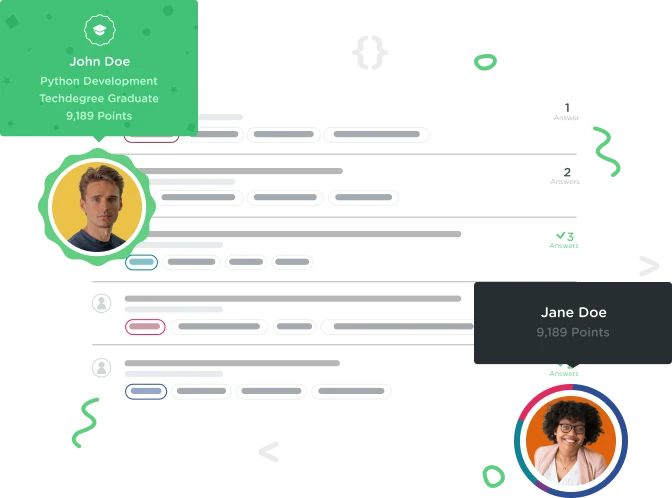

Cathrine Horneland
360 PointsI've got a compiler error but I can't see it.
I've for hours now but can't figure it out.. I thought if I made I somehow put response = console.readLine ("do you know do while loops) inn before the do while loop so I can access it in the rightResponse variable it would work. but it did not.. not the way I did it annyway.. plz help. Ps I would love an explination on how :)
// I have initialized a java.io.Console for you. It is in a variable named console.String response;
String response;
boolean badResponse;
do {
response = console.readLine("Do you understand do while loops? ");
badResponse = (response.equalsIgnoreCase("no"));
if (badResponse) {
console.printf("Sorry, wrong answer!");
}
} while(badResponse);
boolean rightResponse;
rightResponse = (responseEqualsIgnoreCase("Yes"));
if (rightResponse) {
console.printf("Because you said "Yes", you passed the test!");
}
3 Answers
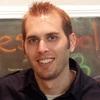
Rick Buffington
8,146 PointsThe key to this one is simplicity. I will walk through each task to help you understand what I mean by that. You do have some syntax errors in your code, however your logic is mostly correct. You just have to do it pretty much exactly how they want you to! :) Here is what I mean:
The tasks are as follows:
- Prompt the user with the question "Do you understand do while loops?" Store the result in a new String variable named response.
- Now continually prompt the user in a do while loop. The loop should continue running as long as the response is No. Don't forget to declare response outside of the do while loop.
- Finally, using console.printf print out a formatted string that says "Because you said <response>, you passed the test!"
For TASK 1, let's break it down into 2 parts - 1) Prompt the user, 2) store that value in a variable called response. For this to work, we actually need to work backwards. So start with part 2 and initialize a string variable named response:
String response = "";
For part 1, we now need to assign response equal to console input:
String response = "";
response = console.readLine("Do you understand while loops?");
This should complete task 1.
For TASK 2, we need to create the loop. We know from the instructions it needs to be a do/while loop. The criteria to keep it running is as long as the response variable is = No. From your code it is apparent you are totally familiar with how to construct it, so I won't go into detail about that. However, we need to keep re-prompting the user, so we need to move our console.readLine assignment INTO the loop (but keep the response declaration above the loop):
do {
response = console.readLine("Do you understand while loops?"); // <- Moved into loop to re-prompt
} while (response.equalsIgnoreCase("No"));
This should complete task 2.
For TASK 3, they want you to print out a formatted string using console.printf. So AFTER your user has exited the loop by typing ANYTHING other than "No", they want you to print that out to the console. console.printf uses special characters to include variables within output without having to do string concatenation. So to do that, you want to use the following OUTSIDE of the do/while loop:
console.printf("Because you said %s, you passed the test!", response);
Adding this line to task 3 should complete your exercise. The %s tells printf to interpolate your response variable INSIDE the text that it prints to the console. Below is the final product:
// I have initialized a java.io.Console for you. It is in a variable named console.
String response = "";
do {
response = console.readLine("Do you understand loops? ");
} while(response.equalsIgnoreCase("No"));
console.printf("Because you said %s, you passed the test!", response);
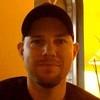
Jeremy Hill
29,567 PointsIt seems as if you are making your code more complicated than it needs to be. Don't worry I did the same thing when I first started out. It should look something like this:
// I have initialized a java.io.Console for you. It is in a variable named console.
String response = "";
do{
response = console.readLine("Do you understand do while loops?");
}while(response.equalsIgnoreCase("No"));
console.printf("Because you said %s, you passed the test!", response);

Cathrine Horneland
360 PointsThank you :)
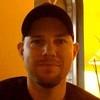
Jeremy Hill
29,567 PointsYou're welcome. Yes I agree, it is nice when you are having to reach out for help and get possitive feedback.

Onyekachukwu Bosah
414 PointsString response; do { response = console.readLine("Do you understand while loops?"); // <- Moved into loop to re-prompt } while (response.equalsIgnoreCase("No"));
Cathrine Horneland
360 PointsCathrine Horneland
360 PointsHi
And thank you so much. I see now that I might have missinterpited the text also. That said I would not have thought it could be done this way. as you said I'm a beginner :) Thanks again for the response, I have to say it really makes me happy to see people act in this manor online. I'm used to rude people.. this forum is awesome :D
Rick Buffington
8,146 PointsRick Buffington
8,146 PointsYou are very welcome. Treehouse is awesome! :)