Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial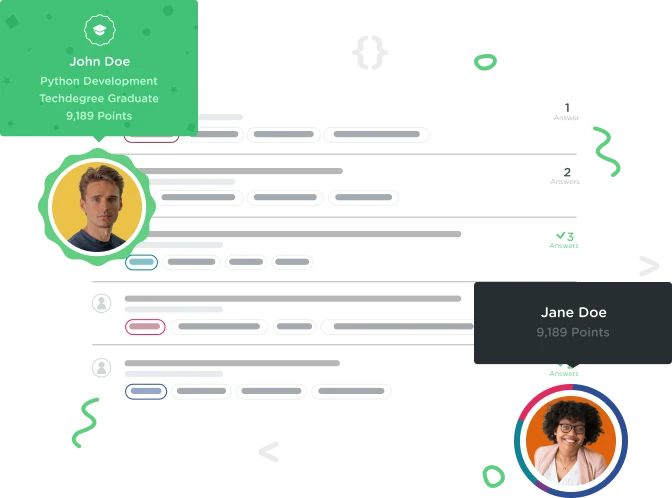

Scott Kim
518 PointsJagged Array Challenge - hard to tell what I am doing...please help!
I keep getting errors regarding = inn lines 12 and 14, but no clues. Can anyone please help?
namespace Treehouse.CodeChallenges
{
public static class MathHelpers
{
public static int[][] BuildMultiplicationTable(int maxFactor)
{
int arraySize = maxFactor + 1;
int[][] BuildMultiplicationTable = new int[arraySize][];
BuildMultiplicationTable[arraySize] = new int[arraySize];
for (int i=0, i < maxFactor; i++)
{
for (int j=0, j < maxFactor; j++)
{
table[i][j] = i * j;
}
}
return table;
}
}
}
4 Answers

jb30
44,807 PointsYou have a comma instead of a semicolon in for (int i=0, i < maxFactor; i++)
and for (int j=0, j < maxFactor; j++)
. You also have not declared table
.

Scott Kim
518 PointsThanks so much JB! I tried again, but the same error I got...any clue may I ask? Thanks a bunch!
==
namespace Treehouse.CodeChallenges { public static class MathHelpers { public static int[][] BuildMultiplicationTable(int maxFactor) { int[][] BuildMultiplicationTable = new int[maxFactor][];
BuildMultiplicationTable[maxFactor] = new int[maxFactor];
for (int i=0; i <= maxFactor; i++)
{
for (int j=0; j <= maxFactor; j++)
{
BuildMultiplicationTable[i][j] = i * j;
}
}
return BuildMultiplicationTable;
}
}
}

jb30
44,807 PointsIn the line int[][] BuildMultiplicationTable = new int[maxFactor][];
, the number of arrays in the first dimension of BuildMultiplicationTable
is set to maxFactor
. Based on the challenge example output, the challenge is expecting there to be maxFactor + 1
arrays in the first dimension of BuildMultiplicationTable
. Try changing it back to int[][] BuildMultiplicationTable = new int[maxFactor + 1][];
If BuildMultiplicationTable
has at least maxFactor + 1
elements in its first dimension, then the line BuildMultiplicationTable[maxFactor] = new int[maxFactor];
sets the length of an array at BuildMultiplicationTable[maxFactor]
. It does not set the length of an array at any other index of BuildMultiplicationTable
. For example, if maxFactor
is not 0
, the length of an array at BuildMultiplicationTable[0]
has not been defined, so any index j
for BuildMultiplicationTable[0][j]
will be outside the bounds of the array BuildMultiplicationTable[0]
.
You could use a for loop to define each of the arrays in the array BuildMultiplicationTable
by
for (int i = 0; i <= maxFactor; i++)
{
BuildMultiplicationTable[i] = new int[maxFactor + 1];
}
which defines the length of an inner array at each element of the first dimensional array in BuildMultiplicationTable
.

Scott Kim
518 PointsAaaaaah, now I get it!! (And it worked out!) THANK YOU SO VERY MUCH JB!!!

Scott Kim
518 PointsFor anyone curious about how this ended with JB's help.
==
namespace Treehouse.CodeChallenges { public static class MathHelpers { public static int[][] BuildMultiplicationTable(int maxFactor) { int[][] BuildMultiplicationTable = new int[maxFactor + 1][]; for (int i=0; i <= maxFactor; i++) { BuildMultiplicationTable[i] = new int[maxFactor+ 1 ];
for (int j=0; j <= maxFactor; j++)
{
BuildMultiplicationTable[i][j] = i*j;
}
}
return BuildMultiplicationTable;
}
}
}
Scott Kim
518 PointsScott Kim
518 PointsAha - thanks! My update as follows, but it says..."Bummer: Index was outside the bounds of the array."
namespace Treehouse.CodeChallenges { public static class MathHelpers { public static int[][] BuildMultiplicationTable(int maxFactor) { int arraySize = maxFactor + 1; int[][] BuildMultiplicationTable = new int[arraySize][];
}
jb30
44,807 Pointsjb30
44,807 PointsIn the line
BuildMultiplicationTable[arraySize] = new int[arraySize];
,arraySize
is outside the bounds of the array because the indices start at0
and go toarraySize-1
. If you usedmaxFactor
as the index instead, then it would only set the dimension of the last inner array. To set the dimension of each of the inner arrays, you could instead define the size of each inner array inside the outer for loop by setting the index toi
.In both the for loops, you might want to change from the form
i < maxFactor
toi <= maxFactor
ori < arraySize
so thatmaxFactor
is included in the calculations.