Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial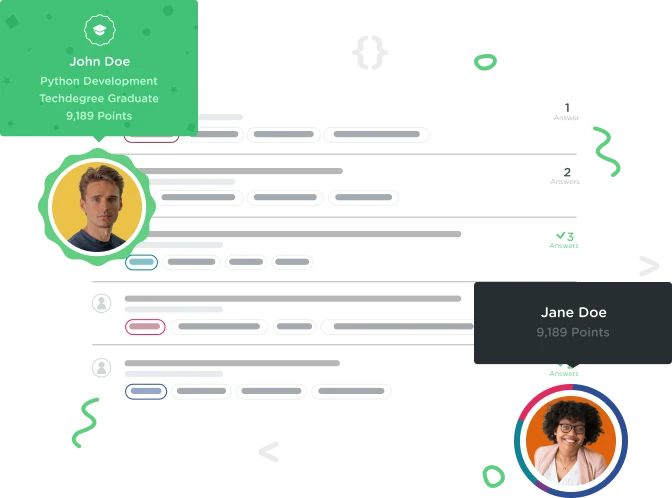

zeinakassem
306 PointsJava Basics challenge Task 2 of 3
prompt the user in a do while loop. The loop should continue running as long as the response is No. Don't forget to declare response outside of the do while loop.

zeinakassem
306 PointsString response ;
Boolean responseIsNo ;
do{
response=console.readLine("do you understand do while loop ?") ;
responseIsNo=(response.equalsIgnoreCase("No") ;
if (responseIsNo) {
console.printf("do you understand do while loop ?");
}
}while(responseIsNo);
4 Answers
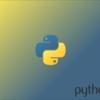
Kevin Faust
15,353 PointsHey Zeinakassem,
What we first do is create a variable string called response. We are going to ask the user if they understand "do while loops" and then we will store their response in the variable we created. Do you remember how to do this? We use the console.readLine() method!
String response = console.readLine("Do you understand do while loops?");
The next part is actually quite tricky for a beginner.
Here we want to use a do-while loop. While our response variable has a "no" value, then we keep asking them if they understand until they write yes.
String response;
do {
response = console.readLine("Do you understand do while loops?");
} while (response=="No");
What we do is first create our response string outside our loop as it says that in the instructions: Don't forget to declare response outside of the do while loop.. Then inside our do loop, we take their input and store it in the response variable. If it equals no, then we keep looping.
Were you able how each line of code worked? I hope you picked up a thing or two from reading this
Happy coding and best of luck!,
Kevin

zeinakassem
306 PointsI understood part 1 but in part 2why we used == instead of .equals "No" is a string so we should use .equals
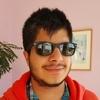
faraz
Courses Plus Student 21,474 PointsHey zeinakassem! You are right, .equals() is better for string comparison. Check out this Stack Overflow post and this video to learn more.
The code below using .equals() instead of == works perfectly for this challenge, I think you're having another issue. If the issue persists after trying the code below, please reply here so I can better assist you. Good luck!
// I have initialized a java.io.Console for you. It is in a variable named console.
String response;
do {
response = console.readLine("Do you understand do while loops?");
} while (response.equals("No"));
console.printf("Because you said %s, you passed the test!", response);
Christopher Augg
21,223 PointsNice explanation! The only thing I would suggest is to use equalsIgnoreCase. The code as written would not consider a user typing in "no", "nO", or "NO" and break out of the loop.
String response;
do {
response = console.readLine("Do you understand do while loops?");
}while(response.equalsIgnoreCase("No"));
console.printf("Because you said %s, you passed the test!", response);
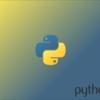
Kevin Faust
15,353 PointsHey Zeinakassem,
Both == and .equals() are the same thing in our case. Generally if we are just comparing two primitives like comparing String with String or int with int, we can just use == as it is quicker. We simply want to see if the two things are exactly the same. However using .equals() is usually used when dealing with objects we create. You haven't learned this yet but we can "override" what the equals() method does meaning that we can choose what two things need in common for them to be similar. You'll learn this later on but just remember that although we could use .equals(), we wouldn't because we are just comparing two primitives.
Does that make sense?
Kevin

zeinakassem
306 PointsI wrote it like your answer but also compiler error
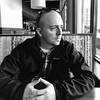
Unsubscribed User
14,189 PointsI just put in the code again and it passed for me. I'll go line by line... Line 1: String response; Line 2: do { Line 3: console.readLine("Do you understand do while loops?"); Line 4: } while (response == "No");
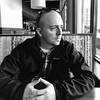
Unsubscribed User
14,189 PointsI went ahead and went back to that challenge. Your answer should look like this:
String response;
do {
response = console.readLine("Do you understand do while loops?");
} while (response == "No");
Essentially, you're declaring your String variable outside of your loop to begin with, letting your program know you'll be storing a String in variable response. The Do While loop will run through first, prompting the user to answer "Do you understand do while loops?" If the answer happens to be "Yes", the loop will still check the condition (while (response == "No")). Seeing that the condition is false, the loop will exit. However, if the answer is "No", the loop condition will be true, causing the user to be prompted again. The only way to exit the loop is for the condition to be false (and the user answers "Yes"). Hope this helps!

zeinakassem
306 Pointscompiler error wrong answer :(
Unsubscribed User
14,189 PointsUnsubscribed User
14,189 PointsCould you post your code please?