Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial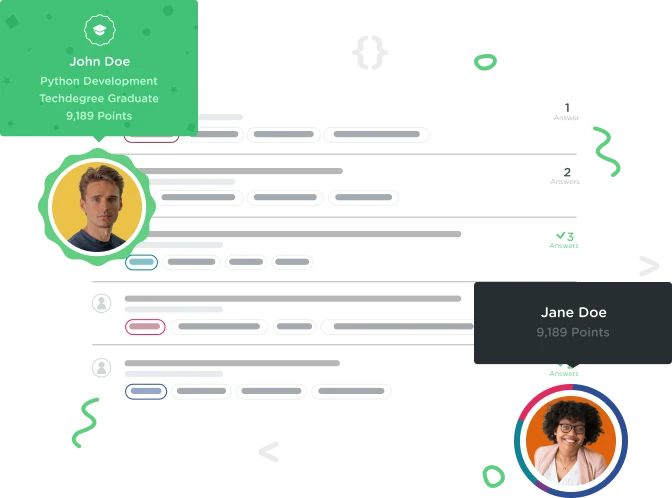

Francois Martel
24,694 PointsJava Objects-Part 2 of Validation Challenge
I had no issue with the first part of the Validation Challenge (make sure the fieldName starts with 'm'). However, it's the second part (make sure the fieldName is in CamelCase) that has me stumped. I've tried breaking up the string into substrings so that I can convert the second letter in fieldName to uppercase using the "toUpperCase()" method. Then I concatenate all the strings. The code compiles, but it does not pass the test. I understand that I am probably overcomplicating this, but I do not know why the challenge is not passing, regardless of the inelegance of my solution.
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
// These things should be verified:
// 1. Member fields must start with an 'm'
// 2. The second letter in the field name must be uppercased to ensure camel-casing
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
if (fieldName.charAt(0) != 'm') {
throw new IllegalArgumentException("Please start the field with the letter 'm'");
}
if (fieldName != fieldName.charAt(0) + fieldName.substring(1, 2).toUpperCase() + fieldName.substring(2)) {
throw new IllegalArgumentException("Please write it in CamelCase");
}
return fieldName;
}
}
1 Answer
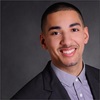
Mario Blokland
19,750 PointsHi Francois Martel,
you actually don't need the substring method since you are only checking the second character of the fieldName which index is 1 (even though you could use the substring method if you wanted too though). What I would do is something like:
// Pseudocode
if (fieldName.charAt(Index) is between 'a' and 'z' or
fieldName.charAt(Index) is not a letter) {
then throw a new exception
}
There are almost always more solutions for a problem but I think this should give you a good idea and if you implement this hint as real java code you should be good to go.
Francois Martel
24,694 PointsFrancois Martel
24,694 PointsHi Mario,
Thanks for the help. You were right that I didn't need to use the substring method since I was only checking the second character. I also didn't realize at first that I needed to test that the second character is indeed a letter, in addition to it being a capital letter. I also realized that I mistakenly was trying to call methods on char, when I should have been calling them on the "Character" class.
I managed to solve this challenge once I considered the above points. Thanks again for your help, it certainly did make a difference.
Mario Blokland
19,750 PointsMario Blokland
19,750 PointsGreat that you could manage to pass this exercise and that I could help you. Keep up the good work! :D