Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial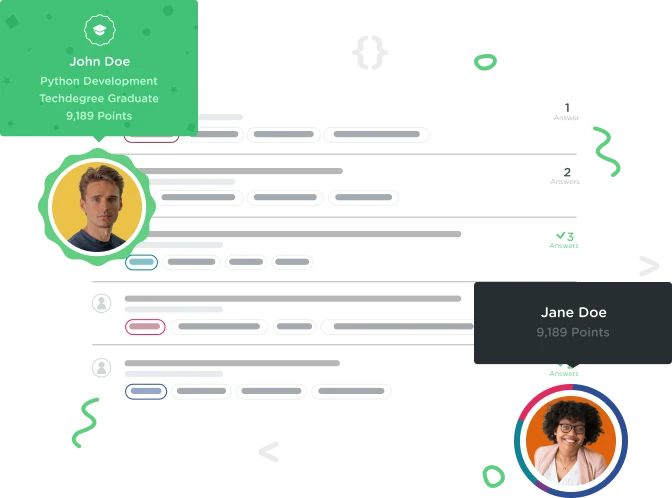

Chad Whiting
Full Stack JavaScript Techdegree Student 4,652 Pointsjavascript function returning multiple values
Doing the Random Quote Generator and I have gotten the function to return 2 values the quote and the source but when it comes to any other values even though I type it exactly like the others it doesn't show up when clicking the button. Here's the code:
const quotes = [
{quete: '1w', by: 'me1', citation: 'c1', year:'y1'},
{quete: '2w', by: 'me2', citation: 'c2', year: 'y2'},
{quete: '3w', by: 'me3', citation: 'c3', year: 'y3'},
{quete: '4w', by: 'me4', citation: 'c4', year: '1986'},
{quete: '5w', by: 'me5', citation: 'c5', year: 'y4'}
]
/***
* `getRandomQuote` function
***/
function getRandomQuote() {
const rando = Math.floor(Math.random() * quotes.length);
let q = quotes[rando].quete;
let b = quotes[rando].by;
let c = quotes[rando].citation;
let y = quotes[rando].year;
return [q, b, c, y];
}
/***
* `printQuote` function
***/
function printQuote() {
let rnd = getRandomQuote();
let q = rnd[0];
let b = rnd[1];
let c = rnd[2];
let y = rnd[3];
document.querySelector(".quote").innerHTML = `${q}`;
document.querySelector(".source").innerHTML = `${b}`;
document.querySelector(".citation").innerHTML = `${c}`;
document.querySelector(".year").innerHTML = `${y}`;
}
/***
* click event listener for the print quote button
* DO NOT CHANGE THE CODE BELOW!!
***/
document.getElementById('load-quote').addEventListener("click", printQuote, false);
so basically it shows the first 2 but then the next 2 are not even in the inspect element after pressing the button
2 Answers
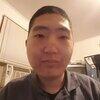
Joseph Yhu
PHP Development Techdegree Graduate 48,637 PointsI believe you can't use innerHTML
in an inline element, e.g. <span>
if you are also using innerHTML
in its parent element, e.g. <p>
; try using insertAdjacentHTML instead. I've tested it, and it works.
Of course, you can also change the two <span>
elements to <p>
elements as well, making sure to move the last </p>
tag to the right place.
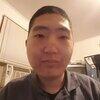
Joseph Yhu
PHP Development Techdegree Graduate 48,637 PointsCan I see your index.html file? I tested it with this simple index file:
<!DOCTYPE html>
<html>
<head>
<title>test</title>
</head>
<body>
<p class="quote"></p>
<p class="source"></p>
<p class="citation"></p>
<p class="year"></p>
<input type="submit" id="load-quote" value="Click">
<script src="test.js"></script>
</body>
</html>
and it displayed everything.

Chad Whiting
Full Stack JavaScript Techdegree Student 4,652 Points<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Random Quotes</title>
<link rel="stylesheet" href="css/normalize.css">
<link rel="stylesheet" href="css/styles.css">
</head>
<body>
<header>
<h1>Random Quotes</h1>
<button id="load-quote" class="load-quote">Show another quote</button>
</header>
<div class="container">
<div id="quote-box" class="quote-box">
<p class="quote">Every great developer you know got there by solving problems they were unqualified to solve until they actually did it.</p>
<p class="source">Patrick McKenzie<span class="citation">Twitter</span><span class="year">2016</span></p>
</div>
</div>
<script src="js/script.js"></script>
</body>
</html>
Chad Whiting
Full Stack JavaScript Techdegree Student 4,652 PointsChad Whiting
Full Stack JavaScript Techdegree Student 4,652 PointsThanks was able to get the insertAdjacentHTML to work. Was trying to keep the index.html file the same cause that was what the course gave to me to try and finish with. But also don't really get why i needed to use something that was taught in the course yet just to finish 8\