Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial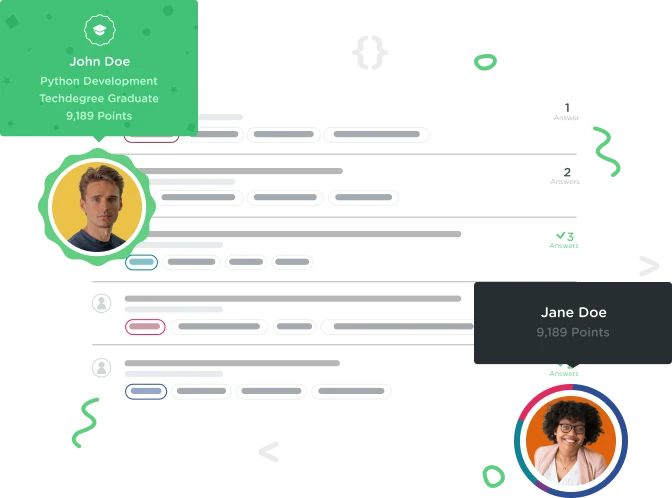
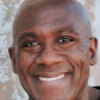
Jonathan Tucker
Ubora | Web Development Techdegree Student 16,987 PointsJS quandary: Treating strings as numbers with conditional statements
Hello Treehouse Community! As part of a coding challenge I created a JS program in which we treated strings as numbers with conditional statements. I successfully built the original intent of the program which was to collect two number between 10 and 25. If the user doesn't put any number, then the If-Then stops the program and tells the user to try again. I noticed, however, that it was accepting values outside of the 10-25 parameter.
I need help creating the exception preventing the user from proceeding with putting a number less than 10 or greater than 25. I can't work out the logic in my head right now. Any advice is greatly appreciated.
Here's my code:
//Display an alert asking user to play
alert("Would you like to play a game? Click OK.");
alert("Please provide a low and high number between 10 and 25. Click OK to begin.");
// Collect input from a user
const inputLow = prompt("Please provide a low number");
const inputHigh = prompt("Please please provide a high number");
// Convert the input to a number
const lowNumber = parseInt(inputLow);
const highNumber = parseInt(inputHigh);
//Select the <main> HTML element
const main = document.querySelector("main");
//Check if lowNumber OR highNumber is not a number
if ( isNaN(lowNumber) && isNaN(highNumber) ) {
main.innerHTML =
`<h2>You need to provide two numbers. Try again.<h2>`;
}
else {
// Use Math.random() and the user's number to generate a random number
const randomNumber = Math.floor( Math.random() * (highNumber - lowNumber + 1) ) + lowNumber;
// Create a message displaying the random number
main.innerHTML =
`<h2>${randomNumber} is a random number between ${lowNumber} and ${highNumber}.</h2> <p>Refresh browser to play again. Thank you!</p>`;
}
1 Answer
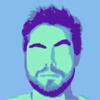
Cameron Childres
11,818 PointsHi Jonathan,
One way to accomplish this would be adding the check with an "else if" statement:
if ( isNaN(lowNumber) && isNaN(highNumber) ) {
main.innerHTML =
`<h2>You need to provide two numbers. Try again.<h2>`;
} else if ( lowNumber < 10 || highNumber > 25) {
main.innerHTML =
`<h2>The first number must be greater than or equal to 10. The second must be less than or equal to 35. Try again.</h2>`
} else {
const randomNumber = Math.floor( Math.random() * (highNumber - lowNumber + 1) ) + lowNumber;
main.innerHTML =
`<h2>${randomNumber} is a random number between ${lowNumber} and ${highNumber}.</h2> <p>Refresh browser to play again. Thank you!</p>`;
}
Hope this helps! Let me know if you have any questions.
Jonathan Tucker
Ubora | Web Development Techdegree Student 16,987 PointsJonathan Tucker
Ubora | Web Development Techdegree Student 16,987 PointsThat worked! I had originally used
||
In my first temp to spot a user's incorrect input, but then I got confused when using the<
and>
. Now it seems so simple. I guess I was making the logic more complicated then needed. Thank you, Cameron Childres!