Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial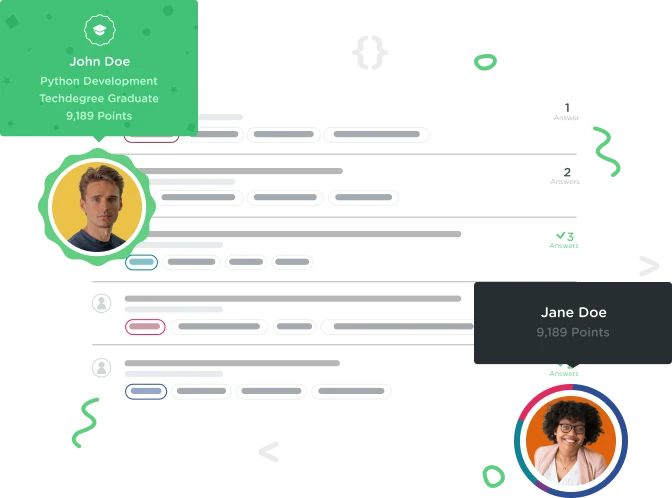
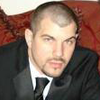
Gary Calhoun
10,317 PointsJust failed my first Fizz Buzz PHP assessment test..
So I just had a chance to take an online assessment test for a php developer job I applied for. I finished the php dev track here on treehouse and I know we just scratched the surface of object oriented programming, nonetheless I wasn't sure how to write out the answer for this can someone explain it to me? I believe I need to have the functions evaluate to be equal or not equal and generate fizz or buzz. Was I supposed to write an if statement or something similar?
Instructions: The aim of this test is to implement the FizzBuzz problem.
FizzBuzz is defined for the "natural numbers" (numbers greater than zero) as:
When divisible by 3, you should return "Fizz". When divisible by 5, you should return "Buzz". When divisible by both 3 and 5, you should return "FizzBuzz". When divisible by neither, you should return the number itself.
This is where I am supposed to put my solution
<?php
class FizzBuzz {
public function __construct($number) {
$this->number = $number;
}
public function __toString() {
return '1';
}
}
?>
<?php
require 'FizzBuzz.php';
class FizzBuzzTest extends PHPUnit_Framework_TestCase
{
public function testInputOne()
{
$fizzbuzz = new FizzBuzz(1);
$this->assertEquals('1', (string) $fizzbuzz);
}
public function testInputThree()
{
$fizzbuzz = new FizzBuzz(3);
$this->assertEquals('Fizz', (string) $fizzbuzz);
}
public function testInputFive()
{
$fizzbuzz = new FizzBuzz(5);
$this->assertEquals('Buzz', (string) $fizzbuzz);
}
public function testInputFifteen()
{
$fizzbuzz = new FizzBuzz(15);
$this->assertEquals('FizzBuzz', (string) $fizzbuzz);
}
public function testInputOneHundredAndOne()
{
$fizzbuzz = new FizzBuzz(101);
$this->assertEquals('101', (string) $fizzbuzz);
}
public function testInputNinetyNine()
{
$fizzbuzz = new FizzBuzz(99);
$this->assertEquals('Fizz', (string) $fizzbuzz);
}
public function testInputOneHundred()
{
$fizzbuzz = new FizzBuzz(100);
$this->assertEquals('Buzz', (string) $fizzbuzz);
}
public function testInputOneHundredAndFive()
{
$fizzbuzz = new FizzBuzz(105);
$this->assertEquals('FizzBuzz', (string) $fizzbuzz);
}
}
?>
The console logs out 7 errors
PHPUnit 3.6.10 by Sebastian Bergmann.
.FFFFFFF
Time: 0 seconds, Memory: 1.75Mb
There were 7 failures:
1) FizzBuzzTest::testInputThree Failed asserting that two strings are equal. --- Expected +++ Actual @@ @@ -'Fizz' +'1'
/tmp/sandbox-dde76935d8b8506f0c22a2927b5f978eaf9e42e4/FizzBuzzTest.php:16 /usr/local/bin/phpunit:46
2) FizzBuzzTest::testInputFive Failed asserting that two strings are equal. --- Expected +++ Actual @@ @@ -'Buzz' +'1'
/tmp/sandbox-dde76935d8b8506f0c22a2927b5f978eaf9e42e4/FizzBuzzTest.php:22 /usr/local/bin/phpunit:46
3) FizzBuzzTest::testInputFifteen Failed asserting that two strings are equal. --- Expected +++ Actual @@ @@ -'FizzBuzz' +'1'
/tmp/sandbox-dde76935d8b8506f0c22a2927b5f978eaf9e42e4/FizzBuzzTest.php:28 /usr/local/bin/phpunit:46
4) FizzBuzzTest::testInputOneHundredAndOne Failed asserting that '1' matches expected '101'.
/tmp/sandbox-dde76935d8b8506f0c22a2927b5f978eaf9e42e4/FizzBuzzTest.php:34 /usr/local/bin/phpunit:46
5) FizzBuzzTest::testInputNinetyNine Failed asserting that two strings are equal. --- Expected +++ Actual @@ @@ -'Fizz' +'1'
/tmp/sandbox-dde76935d8b8506f0c22a2927b5f978eaf9e42e4/FizzBuzzTest.php:40 /usr/local/bin/phpunit:46
6) FizzBuzzTest::testInputOneHundred Failed asserting that two strings are equal. --- Expected +++ Actual @@ @@ -'Buzz' +'1'
/tmp/sandbox-dde76935d8b8506f0c22a2927b5f978eaf9e42e4/FizzBuzzTest.php:46 /usr/local/bin/phpunit:46
7) FizzBuzzTest::testInputOneHundredAndFive Failed asserting that two strings are equal. --- Expected +++ Actual @@ @@ -'FizzBuzz' +'1'
/tmp/sandbox-dde76935d8b8506f0c22a2927b5f978eaf9e42e4/FizzBuzzTest.php:52 /usr/local/bin/phpunit:46
FAILURES! Tests: 8, Assertions: 8, Failures: 7.
1 Answer

Aaron Graham
18,033 PointsYou want to change the __toString() function to suit your needs. You are on the right track with the if/else idea. Basically, your __toString() function should test $this->number against the different possibilities given to you, and return one of the following based on that set of tests - "Fizz", "Buzz", "FizzBuzz", or the string equivalent of $this->number.
If your confusion was about the FizzBuzzTest class and all of its assertions, don't worry about it. That is just a set of tests to make sure your code does what it should. For example:
$fizzbuzz = new FizzBuzz(1);
$this->assertEquals('1', (string) $fizzbuzz);
Will test to see that, when given the number '1', your code returns the correct value. In this case, the string representation of the number itself, since '1' isn't divisible by '3' or '5'.
Gary Calhoun
10,317 PointsGary Calhoun
10,317 PointsThanks, I will research it more but at least now I have an idea of what to expect from potential employers. I need to really sharpen my php skills.