Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial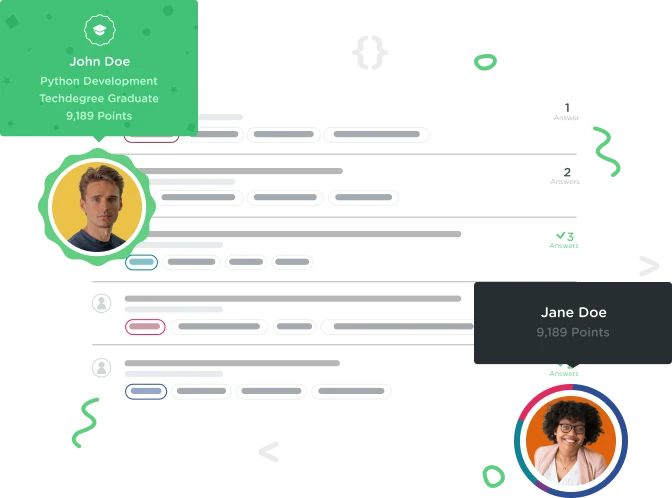
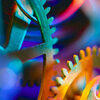
ja5on
10,338 PointsLearning the DOM - hoping my use of appendChild is ok, first time I've used it.
<!DOCTYPE html>
<html>
<head>
<title>The DOM tree.</title>
<body>
<div id="page">
<h1 id="myHeader">List</h1>
<h2>Buy groceries</h2>
<br>
<input type="text" id="inputColor">
<button id="myButton">Text to color</button>
<ul>
<li>Apples</li>
<li>Oranges</li>
<li>Potatoes</li>
<li>balsamic vinegar</li>
<li>Ready Salted Crisps</li>
</ul>
<p id="myText"></p>
<script src="list.js"></script>
</div>
</body>
</head>
</html>
const myHeader = document.getElementById("myHeader");
const myButton = document.getElementById("myButton");
const myText = document.getElementById("myText");
const pElement = document.createElement("p");
myButton.addEventListener("click", function(){
myHeader.style.color = inputColor.value;
});
/* ------- myText can be used many times instead
of using document.getElementById ------- */
myText.textContent = "This is my added text";
myText.style.color = "white";
myText.style.fontSize = "35px";
myText.style.backgroundColor ="grey";
/* ------- Just check here please if my code is correct
seems so, first time i've used appendChild ------- */
pElement.textContent = "Hello I'm the new Element";
document.body.appendChild(pElement);
7 Answers
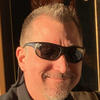
Peter Vann
36,427 PointsHi jas0n!
I tested your code like this:
<!DOCTYPE html>
<html>
<head>
<title>The DOM tree.</title>
<body>
<div id="page">
<h1 id="myHeader">List</h1>
<h2>Buy groceries</h2>
<br>
<input type="text" id="inputColor">
<button id="myButton">Text to color</button>
<ul>
<li>Apples</li>
<li>Oranges</li>
<li>Potatoes</li>
<li>balsamic vinegar</li>
<li>Ready Salted Crisps</li>
</ul>
<p id="myText"></p>
</div>
<script>
const myHeader = document.getElementById("myHeader");
const myButton = document.getElementById("myButton");
const myText = document.getElementById("myText");
const pElement = document.createElement("p");
myButton.addEventListener("click", function(){
myHeader.style.color = inputColor.value;
});
/* ------- myText can be used many times instead
of using document.getElementById ------- */
myText.textContent = "This is my added text";
myText.style.color = "white";
myText.style.fontSize = "35px";
myText.style.backgroundColor ="grey";
/* ------- Just check here please if my code is correct
seems so, first time i've used appendChild ------- */
pElement.textContent = "Hello I'm the new Element";
document.body.appendChild(pElement);
</script>
</body>
</head>
</html>
When I typed blue in the textbox "List" turned blue, so that works.
If you notice, the script tag is the last element before the closing body tag.
When your code runs as written, the last element before the closing body tag (and after the closing script tag) becomes:
<p>Hello I'm the new Element</p>
Full body as inspected:
<body>
<div id="page">
<h1 id="myHeader" style="color: blue;">List</h1>
<h2>Buy groceries</h2>
<br>
<input type="text" id="inputColor">
<button id="myButton">Text to color</button>
<ul>
<li>Apples</li>
<li>Oranges</li>
<li>Potatoes</li>
<li>balsamic vinegar</li>
<li>Ready Salted Crisps</li>
</ul>
<p id="myText" style="color: white; font-size: 35px; background-color: grey;">This is my added text</p>
</div>
<script>
const myHeader = document.getElementById("myHeader");
const myButton = document.getElementById("myButton");
const myText = document.getElementById("myText");
const pElement = document.createElement("p");
myButton.addEventListener("click", function(){
myHeader.style.color = inputColor.value;
});
/* ------- myText can be used many times instead
of using document.getElementById ------- */
myText.textContent = "This is my added text";
myText.style.color = "white";
myText.style.fontSize = "35px";
myText.style.backgroundColor ="grey";
/* ------- Just check here please if my code is correct
seems so, first time i've used appendChild ------- */
pElement.textContent = "Hello I'm the new Element";
document.body.appendChild(pElement);
</script><p>Hello I'm the new Element</p> <!-- YOUR APPENDED ELEMENT -->
</body>
Because you appended to the body element, that's where it got placed in the DOM.
Not sure if that's exactly what you intended, so it's difficult for me to say if it's "correct" or not.
I wish these forums had the ability to attach or include screenshots, so I could show you the result visually.
I hope that helps.
Stay safe and happy coding!
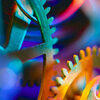
ja5on
10,338 PointsThanks yes I forgot about not using script tag as I only copied it out of VS (sorry), and workspaces still down so I couldn't snapshot either :-( But yes I was trying to create a new P element with a sentence in via the DOM so yes I think it works and I've more encouraged now. It doesn't matter about the layout or look of the program as I'm just expanding (learning) and having fun and new ideas with it all the while, I know you can probably tell what I'm doing just by reading my code I just wanted a more intelligent person in JavaScript to browse through, thanks :-)
About appending to the body, I'm not sure if its ok to do that or in this case would you recommend something else/better practice?
Did you ever watch hitch hikers guide to the galaxy? The original series? Quirky - well just completely bizarre, but funny nonetheless.... As always from across the seas thanks for your help/advice.
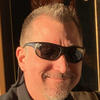
Peter Vann
36,427 PointsYo jas0n!
No worries...
You are very wise to be running experiments to grapple with this stuff.
I certainly encourage it, and of course, I do it all the time.
In fact, when you really start deviating from the courses and challenges, it's a good sign that you are absorbing what you are learning.
It shows you have enough confidence to get creative.
It's kind of like learning the alphabet, phonics, grammar, punctuation, etc., and eventually moving on to forming your own sentences and then eventually writing your own stories (i.e.: apps!).
As far as appending to the body, it's certainly OK, but it's more of a question of is that precisely the placement you intended?!?
Just keep in mind that, obviously as demonstrated in this case, appendChild adds the new element as the last element nested inside the parent element.
One comment: since the JavaScript tag should be the very last tag in the body element, by my way of thinking, it would probably be better to figure out to make sure it gets added just before the script tag (and it will still be the last visible element on the page). Also, you want to add it, in the JS code, before any other JS code that might further manipulate that new node.
See if you can figure out how to do that. There are several ways it could be achieved, though, for sure - and no one way is necessarily better than another way. If the new node ends up where you intend it - voila! Success!?!
Also, keep in mind, the whole point of making the script tag dead last in the body is to ensure the entire DOM had loaded before the JS monkeys with it. (Otherwise, your code would have to execute in the document.onload function or equivalent).
You definitely want to make sure the whole DOM is loaded before you try to run DOM manipulation with JS. It's very difficult to debug why your script didn't work right if the JS executes before its intended targets are fully rendered.
BTW, I have seen the HHGTTG series, but I did read the book years ago and very much enjoyed it. I'll have to look out for the series. I imagine I'd like that too. (42!?! LOL)
If you are much of a reader, it's not comedy, but the entire Ender Game series was fantastic. Orson Scott Card is a phenomenal author. One thing he did in the series, which I thought was really cool, was this:
The (1st) book Ender's Game and the (5th) book, Ender's Shadow basically cover a lot of the same events (by and large, a significant amount, anyway), but Ender's Game is told from Ender's perspective and Ender's shadow is told from another character, Bean's perspective. I found it very clever, especially because it definitely calls attention to how separate individuals can perceive the same events differently, sometimes drastically so. Like in the case of if one character is privy to some related facts that the other one is not. Or in judging whether another character is being genuine or not. It really added some unusual depth to the material.
More soon...
As usual, I hope that helps.
Stay safe and happy coding!
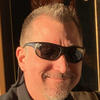
Peter Vann
36,427 PointsBTW, when I was a Junior in college, I was able to go on Westmont College's Europe semester, spending three months (late august through just before Christmas) traveling all over the UK, southern Europe, and even spending 3 weeks in Israel. I was able to see London, Cheltenham, Stratford, Brighton, Exeter, and Edinburough (Ireland didn't make the itinerary, unfortunately). I did get to go to my own personal Mecca at the time, The Marquee Club in Soho. England was definitely my favorite country to visit, although Greece was pretty cool, too. I really enjoyed traveling around London on foot and via The Tube. Fortunately, I took my sister's advice, and instead of frequenting the lame fast-food places, like most of my fellow students, I tended to gravitate towards the pubs for "real food", such as Sheppard's pie and bangers-n-mash.
One funny story, we stayed for two weeks at the University of London in Camden Town. Every morning I would end up eating the classic English full breakfast plate of eggs, bacon, bangers, stewed tomatoes, sauteed mushroom, and beans. (I liked to eat hearty back then!?!). By the end of the two weeks, though, I was absolutely sick of eating it, so I talked some friends into skipping the school breakfast and heading straight to a local restaurant for lunch. I glanced at the menu and found something called "The Hamburger Deluxe Special". It sounded perfect, so I ordered without paying attention to the details. What arrived was a plate with a hamburger patty, eggs, bacon, bangers, stewed tomatoes, sauteed mushrooms, and beans. My friends laughed SOOOOO hard!?! LOL
Also, riding on the top of a double-decker bus in London, my buddy asked the conductor (ticket-taker?!?, worked on the bus anyway, not sure his exact proper title!?!) if he ever ate McDonald's. His reply was, "That stuff is shite. I wouldn't touch it with a barge pole!?!" My friend was, like, "What's a barge pole?" He replied, "Y'know a pole about 10 feet long!?! "Oh, OK, now I got it!?!" We say "I wouldn't touch (such and such) with a 10-foot pole." all the time. In fact, we have a joke: "She'd be a lot prettier if she didn't have all those ten-foot-pole marks all over her!?! LOL
Bonus: Have you ever heard of a "bird" with a butterface?
(That's where you like everything but her face!?! LOL)
https://www.youtube.com/watch?v=NTMINrFtPlU
Cheers for now,
-Pete
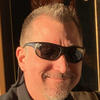
Peter Vann
36,427 PointsOh and given your obvious gravity toward comedy Sci-Fi, I implore you to give Resident Alien a chance. You will be so happy you did!
I'm like a puppy peeing myself waiting for the new season to come out. LOL
The Tick on Amazon Prime is really funny in similar weird and warped ways, too!?! (The actor who plays The Tick is the guy who was Shaun's older brother in "Shaun of the Dead" - Peter Serafinowicz)
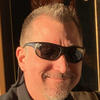
Peter Vann
36,427 PointsOdd sidebar: Are you a Mac or Windows aficionado?!?
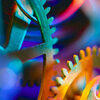
ja5on
10,338 PointsWindows:- Infact I got new system when deciding to learn this stuff:
Windows 10 x64
AMD Ryzen 5 3600 6-core, hyperthreaded 12 core
16gb ram
Nvidia GeForce GTX 1660 Super 6gb
250SSDrive + 1tb drive
Two acer 27inch monitors
Epson ET-2710 Eco Printer
Huge desk too house it all on
and leather chair
Oh and 7 big fans cooling it all so Its like the windy city right by my legs lol.
Its not the best but it certainly plays most games, I'll be on the lookout later in the year for another 16gb ram and a cool ASUS GeForce RTX 3070 8 GB g/card. But really I just needed to "make sure" I have a system well built for any plans to do programming. I used to upgrade systems but now haven't the time.
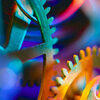
ja5on
10,338 PointsBTW With moving the appendChild you were saying earlier, I found a neat trick of using:-
document.getElementById("appendedHere").appendChild(pElement);
now in the html I used:-
<p id="appendedHere"></p> then just repositioned it according to where I wanted it to show, after all html is the structure..