Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial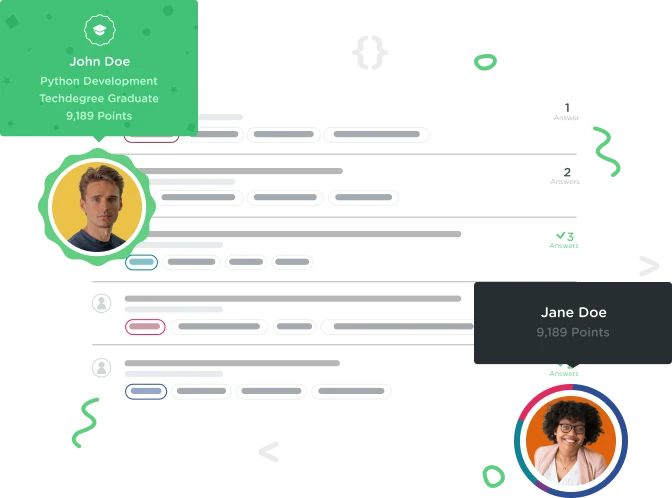
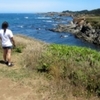
Nancy Melucci
Courses Plus Student 36,143 PointsLoading arraylist of objects into Android Recycler View, no crash but nothing visible.
I am assigned to make a recycler view of objects using names, positions and images of characters from the office. When I loaded these as separate components (string/string/image) it worked great but the instructions are pretty clear - it needs to be an arraylist of objects. I am posting the java code and layouts. Maybe someone can tell me what I am missing. It's always great when the app doesn't crash but I am shooting a little higher here. Thanks. Java first, then xml.
//CardDemoActivity
package com.ebookfrenzy.carddemo;
import android.os.Bundle;
import android.support.design.widget.Snackbar;
import android.support.v7.app.AppCompatActivity;
import android.support.v7.widget.Toolbar;
import android.view.View;
import android.view.Menu;
import android.view.MenuItem;
import android.support.v7.widget.LinearLayoutManager;
import android.support.v7.widget.RecyclerView;
import android.support.design.widget.CollapsingToolbarLayout;
import android.graphics.Color;
public class CardDemoActivity extends AppCompatActivity {
RecyclerView recyclerView;
RecyclerView.LayoutManager layoutManager;
RecyclerView.Adapter adapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_card_demo);
Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
CollapsingToolbarLayout collapsingToolbarLayout =
(CollapsingToolbarLayout) findViewById(R.id.collapsing_toolbar);
collapsingToolbarLayout.setTitle("");
collapsingToolbarLayout.setContentScrimColor(Color.GREEN);
recyclerView =
(RecyclerView) findViewById(R.id.recycler_view);
layoutManager = new LinearLayoutManager(this);
recyclerView.setLayoutManager(layoutManager);
adapter = new RecyclerAdapter();
recyclerView.setAdapter(adapter);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_card_demo, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
//noinspection SimplifiableIfStatement
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
}
//RecyclerAdapter
package com.ebookfrenzy.carddemo;
import android.graphics.Color;
import android.os.Build;
import android.support.v7.widget.RecyclerView;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.view.Window;
import android.view.WindowManager;
import android.widget.ImageView;
import android.widget.TextView;
import android.support.design.widget.Snackbar;
import java.util.ArrayList;
public class RecyclerAdapter extends RecyclerView.Adapter<RecyclerAdapter.ViewHolder> {
ArrayList<Employee> list = new ArrayList<>();
Employee employee1 = new Employee("Pamela Beesly", "Receptionist", R.drawable.pamela_beesly);
Employee employee2 = new Employee("Andy Bernard", "Sales Director", R.drawable.andy_bernard);
Employee employee3 = new Employee("Creed Bratton", "Quality Assurance", R.drawable.creed_bratton);
Employee employee4 = new Employee("Jim Halpert", "Assistant Regional Manager", R.drawable.jim_halpert);
Employee employee5 = new Employee("Ryan Howard", "Vice President, Northeast", R.drawable.ryan_howard);
Employee employee6 = new Employee("Stanley Hudson", "Sales Representative", R.drawable.stanley_hudson);
Employee employee7 = new Employee("Kelly Kapoor", "Customer Service Rep", R.drawable.kelly_kapoor);
Employee employee8 = new Employee("Phyllis Lapin", "Sales Representative", R.drawable.phyllis_lapin);
Employee employee9 = new Employee("Kevin Malone", "Accountant", R.drawable.kevin_malone);
Employee employee10 = new Employee("Angela Martin", "Senior Accountant", R.drawable.angela_martin);
Employee employee11 = new Employee("Oscar Martinez", "Accountant", R.drawable.oscar_martinez);
Employee employee12 = new Employee("Meredith Palmer", "Supplier Relations", R.drawable.meredith_palmer);
Employee employee13 = new Employee("Dwight Schrute", "Assistant Regional Manager", R.drawable.dwight_schrute);
Employee employee14 = new Employee("Michael Scott", "Regional Manager", R.drawable.michael_scott);
Employee employee15 = new Employee("Toby Flenderson", "Human Resources Manager", R.drawable.toby_flenderson);
public void loadData(){
list.add(employee1);
list.add(employee2);
list.add(employee3);
list.add(employee4);
list.add(employee5);
list.add(employee6);
list.add(employee7);
list.add(employee8);
list.add(employee9);
list.add(employee10);
list.add(employee11);
list.add(employee12);
list.add(employee13);
list.add(employee14);
list.add(employee15);
}
class ViewHolder extends RecyclerView.ViewHolder {
public ImageView itemImage;
public TextView itemTitle;
public TextView itemDetail;
public ViewHolder(View itemView) {
super(itemView);
loadData();
itemImage =
(ImageView) itemView.findViewById(R.id.item_image);
itemTitle =
(TextView) itemView.findViewById(R.id.item_title);
itemDetail =
(TextView) itemView.findViewById(R.id.item_detail);
itemView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
int position = getAdapterPosition();
Snackbar.make(v, "Employee: " + list.get(position),
Snackbar.LENGTH_LONG)
.setAction("Action", null).show();
}
});
}
}
@Override
public ViewHolder onCreateViewHolder(ViewGroup viewGroup, int i) {
View v = LayoutInflater.from(viewGroup.getContext())
.inflate(R.layout.card_layout, viewGroup, false);
ViewHolder viewHolder = new ViewHolder(v);
return viewHolder;
}
@Override
public void onBindViewHolder(ViewHolder viewHolder, int i) {
viewHolder.itemTitle.setText(list.get(i).getEmployeeTitle());
viewHolder.itemDetail.setText(list.get(i).getEmployeeDetail());
viewHolder.itemImage.setImageResource(list.get(i).getEmployeePicture());
}
@Override
public int getItemCount() {
return list.size();
}
}
Layout files
tools:context="com.ebookfrenzy.carddemo.CardDemoActivity">
<android.support.v7.widget.RecyclerView
android:id="@+id/recycler_view"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_behavior="@string/appbar_scrolling_view_behavior" />
<android.support.design.widget.AppBarLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:theme="@style/AppTheme.AppBarOverlay">
<android.support.design.widget.CollapsingToolbarLayout
android:id="@+id/collapsing_toolbar"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_scrollFlags="scroll|exitUntilCollapsed"
android:fitsSystemWindows="true"
app:contentScrim="?attr/colorPrimary"
app:expandedTitleMarginStart="48dp"
app:expandedTitleMarginEnd="64dp">
<ImageView
android:id="@+id/backdrop"
android:layout_width="match_parent"
android:layout_height="200dp"
android:scaleType="centerCrop"
android:fitsSystemWindows="true"
app:layout_collapseMode="parallax"
android:src="@drawable/appbar_image" />
<android.support.v7.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
app:popupTheme="@style/AppTheme.PopupOverlay"
app:layout_scrollFlags="scroll|enterAlways"
app:layout_collapseMode="pin"/>
</android.support.design.widget.CollapsingToolbarLayout>
</android.support.design.widget.AppBarLayout>
<include layout="@layout/content_card_demo" />
</android.support.design.widget.CoordinatorLayout>
<!-- card content ->
<?xml version="1.0" encoding="utf-8"?>
<android.support.v7.widget.CardView xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:card_view="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/card_view"
android:layout_margin="5dp"
card_view:cardBackgroundColor="#ffffff"
card_view:cardCornerRadius="12dp"
card_view:cardElevation="3dp"
card_view:contentPadding="4dp"
android:foreground="?selectableItemBackground"
android:clickable="true" >
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="16dp" >
<ImageView
android:layout_width="100dp"
android:layout_height="100dp"
android:id="@+id/item_image"
android:layout_alignParentStart="true"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/item_title"
android:layout_toEndOf="@+id/item_image"
android:layout_toRightOf="@+id/item_image"
android:layout_alignParentTop="true"
android:textSize="30sp" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/item_detail"
android:layout_toEndOf="@+id/item_image"
android:layout_toRightOf="@+id/item_image"
android:layout_below="@+id/item_title" />
</RelativeLayout>
</android.support.v7.widget.CardView>
That's a lot so I'll stop. If something else would be useful I'll be happy to add it.

Ken Alger
Treehouse TeacherNancy;
Did you ever figure out what the issue with this was?
Ken
1 Answer
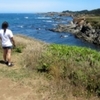
Nancy Melucci
Courses Plus Student 36,143 PointsSadly, no. I have to polish up my skills a bit. Hoping to work on a Treehouse tutorial or two this summer.

Ken Alger
Treehouse TeacherIs your project on GitHub or someplace I can download and play with it?
Nancy Melucci
Courses Plus Student 36,143 PointsNancy Melucci
Courses Plus Student 36,143 PointsNot just sitting around waiting for an answer. Working on it...added ButterKnife. I can see some errors in what I posted, but it's still doing the same thing (running but not showing anything.) I am not going to post anymore code, unless someone is interested in giving me a hint or some help. It's due tonight, and I'll have to submit it as is. I would still love to know what I have missed. I have looked at both Java and XML and made revisions.