Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial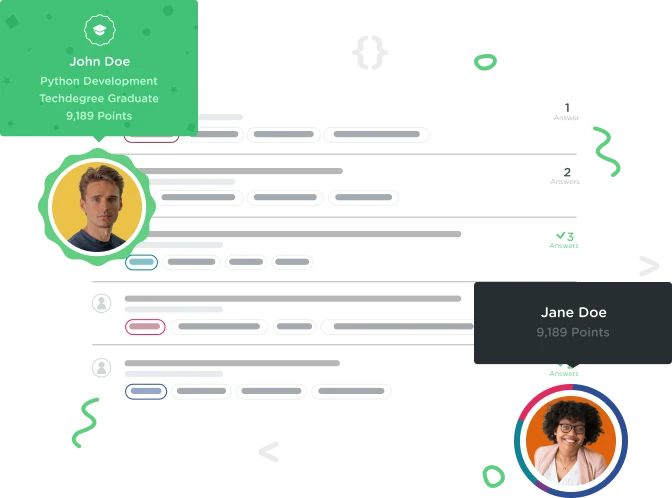
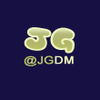
Jonathan Grieve
Treehouse Moderator 91,253 PointsLocalstorage not saving searches
Hi all,
I've followed this great workshop and filled in the functions with the right code but the searches are not being saved into localStorage. I might be able to work out why only no errors are actually showing up on the console.
Any ideas from JS experts out there? :)
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="x-ua-compatible" content="ie=edge">
<title>Search Bar Demo</title>
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="stylesheets/page.css" />
<link href='https://fonts.googleapis.com/css?family=Bitter:400,700' rel='stylesheet' type='text/css'>
</head>
<body>
<div class="header">
<h1 class="header-title">Search</h1>
<form autocomplete="off" id="searchForm">
<input class="text-input" type="text" id="searchBar">
<button class="button" type="submit">Search</button>
</form>
</div>
<div class="recent">
<h2 class="recent-title">Recent Searches</h2>
<button class="button" type="button" id="clearStorage">Clear Storage</button>
<ul class="recent-list" id="recentSearchList"></ul>
</div>
<script type="text/javascript">
'use strict';
function supportsLocalStorage() {
try {
return 'localStorage' in window && window['localStorage'] !== null;
} catch(e) {
return false;
}
}
function getRecentSearches() {
var searches = localStorage.getItem('recentSearches');
if(searches) {
return JSON.parse(searches);
} else {
return [];
}
}
function saveSearchString() {
var searches = getRecentSearches();
if(!str || searches.indexOf(str) > -1) {
return false;
}
searches.push(str);
localStorage.setItem('recentSearches', JSON.stringify(searches));
return true;
}
function removeSearches() {
localStorage.removeItem('recentSearches');
}
// Create an li, given string contents, append to the supplied ul
function appendListItem(listElement, string) {
var listItemElement = document.createElement('LI');
listItemElement.innerHTML = string;
listElement.appendChild(listItemElement);
}
// Empty the contents of an element (ul)
function clearList(listElement) {
listElement.innerHTML = '';
}
window.onload = function() {
if(localStorage) {
var searchForm = document.getElementById('searchForm');
var searchBar = document.getElementById('searchBar');
var recentSearchList = document.getElementById('recentSearchList');
var clearButton = document.getElementById('clearStorage');
// Initialize display list
var recentSearches = getRecentSearches();
recentSearches.forEach(function(searchString) {
appendListItem(recentSearchList,searchString);
});
// Set event handlers
searchForm.addEventListener('submit', function(event) {
var searchString = searchBar.value;
if (saveSearchString(searchString)) {
appendListItem(recentSearchList, searchString);
}
});
clearButton.addEventListener('click', function(event) {
removeSearches();
clearList(recentSearchList);
});
}
};
</script>
</body>
</html>
2 Answers

Simon McKeon
15,517 Pointsif(!str || searches.indexOf(str) > -1) {
return false;
}
What is str
referring to? It is undefined in this context. I assume you are meaning to pass this value in to the function, judging on how you used the function.
saveSearchString(searchString)
Try this.
function saveSearchString(str) {
var searches = getRecentSearches();
if(!str || searches.indexOf(str) > -1) {
return false;
}
...

Craig Curtis
19,985 PointsHad a similar problem, but window also reloaded.
So at the top of the submit event listener, I added: event.preventDefault()
Jonathan Grieve
Treehouse Moderator 91,253 PointsJonathan Grieve
Treehouse Moderator 91,253 PointsFabulous! Got it working. I'd forgotten to add the variable as a parameter to the function. :-)