Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial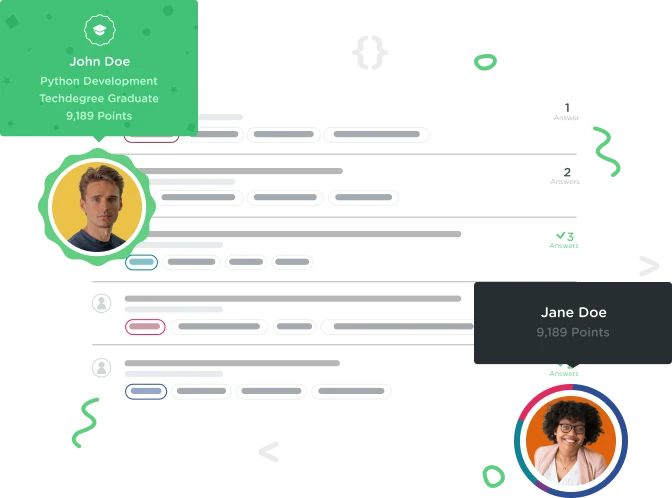

Habibur Rahman Dipto
8,496 PointsLook and Say sequence (PHP)
It’s called the “Look and Say” sequence because each element is what you get by looking at the previous element and saying what’s in it. For example, looking at the first element, 1, you say “one one.” So the second element is “11.” That’s two ones, so the third element is “21.” Similarly, that’s one two and one one, so the fourth element is “1211,” and so on.
I need to know how this program works step by step... Is their any law for the sequence.
<?php
function lookandsay($s) {
// initialize the return value to the empty string
$r = '';
// $m holds the character we're counting, initialize to the first
// character in the string
$m = $s[0];
// $n is the number of $m's we've seen, initialize to 1
$n = 1;
for ($i = 1; $i < strlen($s); $i++) {
// if this character is the same as the last one
if ($s[$i] == $m) {
// increment the count of this character
$n++;
} else {
// otherwise, add the count and character to the return value
$r .= $n.$m;
// set the character we're looking for to the current one
$m = $s[$i];
// and reset the count to 1
$n = 1;
}
}
// return the built up string as well as the last count and character
return $r.$n.$m;
}
for ($i = 0, $s = 1; $i < 10; $i++) {
$s = lookandsay($s);
print "$s\n";
}
2 Answers
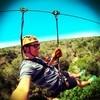
thomascawthorn
22,986 Points<?php
function lookAndSay($currentSequence)
{
// initialize the return value to the empty string
$newSequence = '';
// $m holds the character we're counting, initialize to the first
// character in the string
$character = $currentSequence[0];
// $n is the number of $character's we've seen, initialize to 1
$currentCharacterCount = 1;
for ($i = 1; $i < strlen($currentSequence); $i++) {
// Loop through every character in the current sequence.
if ($currentSequence[$i] == $character) {
// if this character is the same as the last one e.g. sequence is 11
// You're going to increase the count of the number '1' to 2 - you have two number 1's = 21
$currentCharacterCount++;
} else {
// If the next character in the sequence is not the same e.g. 21
// then you're going to restart the count for the number of times you've seen
// the new character.
// You're on a new number, so 'store' the previous block in the new sequence.
$newSequence .= $currentCharacterCount . $character;
// Move the character along to the next one
$character = $currentSequence[$i];
// you've only see one of these new characters so far, so reset the counter bacl
// to 0
$currentCharacterCount = 1;
}
}
// return the built up string as well as the last count and character
return $newSequence . $currentCharacterCount . $character;
}
$sequence = 1;
for ($i = 0; $i < 10; $i++) {
// Loop through the sequence, passing the result back into the fuction.
$sequence = $this->lookandsay($sequence);
print "$sequence<br>";
}
Okay this took me a while because it's poorly named. What's the general thing going on? Well you're looking through each character in a string. For each loop inside the function, you're counting how many times you've seen that character. If the character changes (e.g. 112) then you reset the count to 1 and start looking for the number 2.
You're counting how many times you see each number, then appending (joining) the count and character to the new sequence. You then return everything at the end.
Just keep at it, and you'll eventually see!
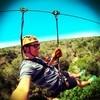
thomascawthorn
22,986 PointsThis would be helped hugely if you had better named variables! To understand this, I would rename the variables from things like 'r' and 's' to what they actually are.
For instance, the comments say that '$m holds the character we're counting', so why not call it 'character' or 'currentCharacter'!
if "$n is the number of $m's we've seen", then why not call is 'characterCount'.
Readable code is much easier to understand!

Habibur Rahman Dipto
8,496 Pointsi found this from a book will you please rewrite the code and help me to understand how its work?
thomascawthorn
22,986 Pointsthomascawthorn
22,986 PointsAlso comments can make it hard to read, maybe take a look without:
Habibur Rahman Dipto
8,496 PointsHabibur Rahman Dipto
8,496 Pointsthnx buddy. :D