Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial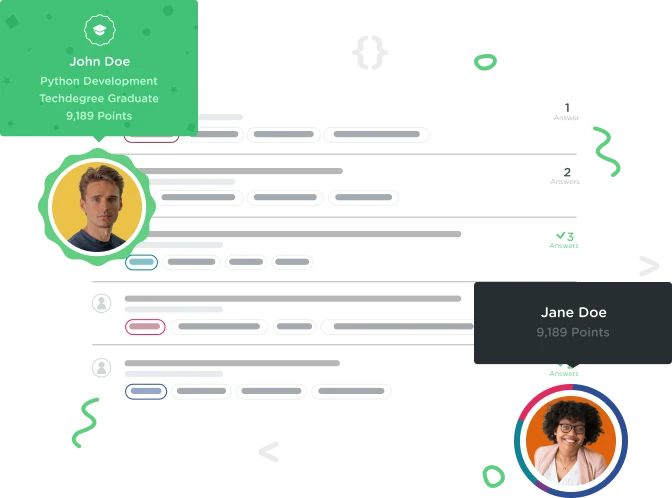
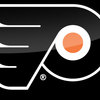
shawn stokes
5,651 Pointslooping through an array?
ok so here is my array
<?php
$category = array();
$category[] = array(
"id" => "100",
"name" => "category name",
"img" => "img/name_100.jpg",
"products" => array(
"product01" => array(
"rel_cat_id" => "100",
"product_id" => "110",
"product_name" => "p_name",
"product_img" => "img/product/name110.jpg",
),
"product02" => array(
"rel_cat_id" => "100",
"product_id" => "120",
"product_name" => "p_name",
"product_img" => "img/product/nam120.jpg",
"alt_product" => array(
"id" => "122",
"rel_product_id" => "120",
"rel_cat_id" => "100",
"name" => "rp_name",
"img" => "img/product/name122.jpg",
)
),
"product03" => array(
"rel_cat_id" => "100",
"product_id" => "130",
"product_name" => "p_name",
"product_img" => "img/product/name130.jpg",
)
)
);
$category[] = array(
"id" => "200",
"name" => "category name",
"img" => "img/name_02.jpg",
"products" => array(
"product01" => array(
"rel_cat_id" => "200",
"product_id" => "210",
"product_name" => "p_name",
"product_img" => "img/product/name210.jpg",
),
"product02" => array(
"rel_cat_id" => "200",
"product_id" => "220",
"product_name" => "p_name",
"product_img" => "img/product/name220.jpg",
"alt_product" => array(
"id" => "222",
"rel_product_id" => "220",
"rel_cat_id" => "200",
"name" => "rp_name",
"img" => "img/product/name222.jpg",
)
),
)
);
$category[] = array(
"id" => "300",
"name" => "category name",
"img" => "img/name_300.jpg",
"products" => array(
"product01" => array(
"rel_cat_id" => "300",
"product_id" => "310",
"product_name" => "p_name",
"product_img" => "img/product/name310.jpg",
)
)
);
?>
i get foreach loops i can make it for some of what i want to get in the category array. now lets say i also want to get names and imgs from all the products and alt_products arrays in this foreach loop. can this be done?
3 Answers

Curtis Schrum
10,174 PointsI would create a class but thats neither here nor there.
Why not reorganize your data a bit? I do not know if this is supposed to be dynamic data or not, if it is you would likely need a nested foreach (mentioned above) for the products array. However, given the data above I did a little reorganization of it below:
<?php
$categories = array(
0 => array(
"id" => "100",
"name" => "category name",
"img" => "img/name_100.jpg",
"products" => array(
"product01" => array(
"rel_cat_id" => "100",
"product_id" => "110",
"product_name" => "p_name",
"product_img" => "img/product/name110.jpg",
),
"product02" => array(
"rel_cat_id" => "100",
"product_id" => "120",
"product_name" => "p_name",
"product_img" => "img/product/nam120.jpg",
"alt_product" => array(
"id" => "122",
"rel_product_id" => "120",
"rel_cat_id" => "100",
"name" => "rp_name",
"img" => "img/product/name122.jpg",
)
),
"product03" => array(
"rel_cat_id" => "100",
"product_id" => "130",
"product_name" => "p_name",
"product_img" => "img/product/name130.jpg",
)
),
),
);
foreach($categories as $category) {
echo 'id: '. $category['id'] . '<br/>';
echo 'name: '. $category['name']. '<br/>';
echo 'img: '. $category['img']. '<br/>';
echo 'rel_cat_id: ' . $category['products']['product01']['rel_cat_id']. '<br/>';
echo 'product_id: '.$category['products']['product01']['product_id']. '<br/>';
echo 'product_name: '. $category['products']['product01']['product_name']. '<br/>';
echo 'product_img: '. $category['products']['product01']['product_img'];
}
?>
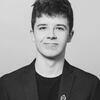
John MacDonald
8,593 PointsTry nesting in 2 foreach loops
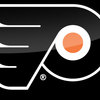
shawn stokes
5,651 Pointsso like
<?php
foreach($category as $title){
echo $title["name"];
foreach ($products as $product){
echo $product["name"];
foreach ($alt_product and $alternate){
echo $alternate["name"];
}
}
}
?>
wouldn't i need to check so see if they are set some how?
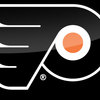
shawn stokes
5,651 Pointsok so this is were i am at now i am getting a Illegal string offset 'alt_name'
<?php
$category = array();
$category[] = array(
"id" => "100",
"name" => "category name 100",
"img" => "img/name_100.jpg",
"products" => array(
"product01" => array(
"rel_cat_id" => "100",
"product_id" => "110",
"product_name" => "p_name 110",
"product_img" => "img/product/name110.jpg",
),
"product02" => array(
"rel_cat_id" => "100",
"product_id" => "120",
"product_name" => "p_name 120",
"product_img" => "img/product/nam120.jpg",
"alt_product" => array(
"id" => "122",
"rel_product_id" => "120",
"rel_cat_id" => "100",
"alt_name" => "rp_name 122",
"img" => "img/product/name122.jpg",
)
),
"product03" => array(
"rel_cat_id" => "100",
"product_id" => "130",
"product_name" => "p_name 130",
"product_img" => "img/product/name130.jpg",
)
)
);
$category[] = array(
"id" => "200",
"name" => "category name 200",
"img" => "img/name_02.jpg",
"products" => array(
"product01" => array(
"rel_cat_id" => "200",
"product_id" => "210",
"product_name" => "p_name 210",
"product_img" => "img/product/name210.jpg",
),
"product02" => array(
"rel_cat_id" => "200",
"product_id" => "220",
"product_name" => "p_name 220",
"product_img" => "img/product/name220.jpg",
"alt_product" => array(
"id" => "222",
"rel_product_id" => "220",
"rel_cat_id" => "200",
"alt_name" => "rp_name 222",
"img" => "img/product/name222.jpg",
)
),
)
);
$category[] = array(
"id" => "300",
"name" => "category name 300",
"img" => "img/name_300.jpg",
"products" => array(
"product01" => array(
"rel_cat_id" => "300",
"product_id" => "310",
"product_name" => "p_name 310",
"product_img" => "img/product/name310.jpg",
)
)
);
?>
and this is my loop it works to products and when i var_dump $alt the name i want is there but when i try to echo it i get the error.
<?php
include("array.php");
foreach($category as $title){
echo $title["name"] . '<br>';
foreach($title as $product){
if (is_array($product)){
foreach($product as $item){
echo $item["product_name"] . '<br>';
foreach($item as $alt_product){
if(is_array($alt_product)){
foreach($alt_product as $alt){
echo $alt["alt_name"] . '<br>';
}
}
}
}
}
}
}
?>
shawn stokes
5,651 Pointsshawn stokes
5,651 Pointsah never though about setting it up that way thanks.
Curtis Schrum
10,174 PointsCurtis Schrum
10,174 PointsI should note I wrote this reply to the original problem I had not seen the additional information yet