Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial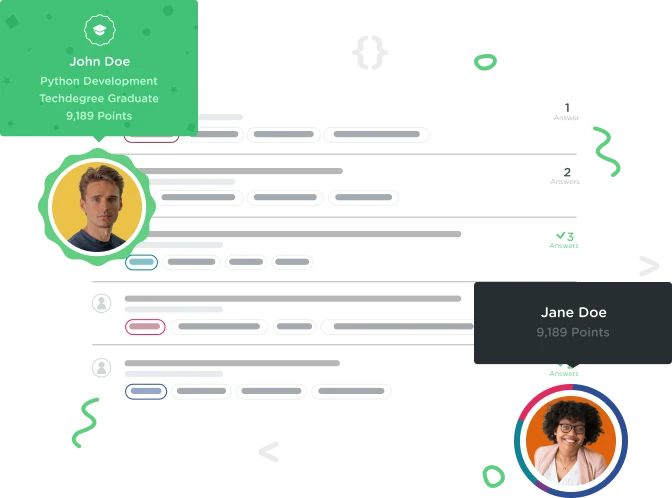

Sam Begdouri
2,328 PointsMaking a function that returns the great of 2 numbers. HOW?
I am stuck on this challenge. I understand the theory, but have no idea how to express it in code. I know I am missing a var, but I have no idea where to place it.
I am becoming more and more confused.
HELP??
function max(num1, num2) {
if (num1>num2) { return num1; } else (num1<num2) { return num2; } } console.log(max);
function max(num1, num2) {
if (num1>num2) {
return num1;
}
else (num1<num2) {
return num2;
}
}
console.log(max);
3 Answers
Tiffany McAllister
25,806 PointsYou do not need to specify the second conditional for the else statement because if the first condition isn't true it will automatically run whatever is in the else block.
function max(num1, num2) {
if (num1 > num2) {
return num1;
}
else {
return num2;
}
}
You also need to log the function to the console like this, because you have to pass in the 2 numbers as arguments for it to work.
console.log(max(2,3));

Alexander Lee
5,964 Pointsfunction max(num1, num2) {
if (num1 > num2) {
return num1; // return num1 if num1 is greater than num2
}
return num2; // return num2 by default, i.e. if the above condition fails then num1 <= num2
}
var x = 10, y = 5; // declare test variables
console.log(max(x,y)); // output to console

Rachelle Wood
15,362 PointsYou can declare your variables right in your function in this case or you can decide to declare them before and outside the function.
Hannah Mills
3,599 PointsHannah Mills
3,599 PointsI was so stuck on this! Thank you! Though still not quite sure what I was doing worng....?!