Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial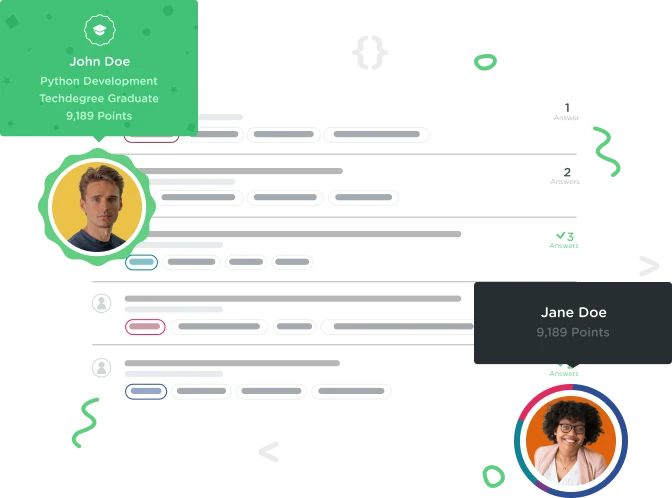

RODRIGO MARTINEZ
5,912 PointsMars Rover Kata in Javascript. Functions for turning and advance. Please help!
Hi. I'm doing this kata in wich i have a rover that i need to be able to give instructions to inside a board of 10x10. Rover can do only 1 movement at a time. Either turn or advance. When i console.log the functions for advance i'm not getting the updated position of the rover on the board. Can't figure it out. I wrote everything and had found a solution but then i basically screwed it overwriting something and can't figure out what I erased or changed. Please help.
// Rover Object Goes Here
// ======================
let grid;
let rover = {
direction: "N",
location: {x:0,y:0},
// path: [{x:0,y:0}]
}
// ======================
//Turn left function
function turnLeft(rover){
switch (rover.direction) {
case "N":
rover.direction = "W";
break;
case "W":
rover.direction = "S";
break;
case "S":
rover.direction = "E";
break;
case "E":
rover.direction = "N";
break;
}
console.log(`turnLeft was called!. Rover is now facing ${rover.direction}` );
}
//Turn right function
function turnRight(rover){
switch (rover.direction) {
case "N":
rover.direction = "E";
break;
case "E":
rover.direction = "S";
break;
case "S":
rover.direction = "W";
break;
case "W":
rover.direction = "N";
break;
}
console.log(`turnRight was called!. Rover is now facing ${rover.direction}` );
}
// Function for moving rover
function moveForward(theRover) {
//Variables
let locationX = theRover.location.x;
let locationY = theRover.location.y;
let roverDir = theRover.direction;
let roverInfoConsole = `Current rover position is x:${locationX} y:${locationY}
Current rover direction is ${roverDir} `;
// Restricted Moves
if ( (roverDir === "N" && locationY <= 0) ||
(roverDir === "E" && locationX >= 9) ||
(roverDir === "S" && locationY >= 9) ||
(roverDir === "W" && locationX <= 0) ) {
console.log(`Cannot move in that direction. The rover would move to a restricted area.
${roverInfoConsole}`);
//allowed moves
//North
} else if (roverDir === "N" && locationY <= 9) {
locationY = locationY - 1;
console.log(`moveForward was called.
Current rover position is x:${locationX} y:${locationY}
Current rover direction is ${roverDir} `);
//East
} else if (roverDir === "E" && locationX < 9) {
locationX = locationX + 1;
console.log(`Current rover position is x:${locationX} y:${locationY}
Current rover direction is ${roverDir} `);
//South
} else if (roverDir === "S" && locationY < 9) {
locationY = locationY + 1;
console.log(`moveForward was called.
Current rover position is x:${locationX} y:${locationY}
Current rover direction is ${roverDir} `);
//West
} else if (roverDir === "W" && locationX < 9) {
locationX = locationX - 1;
console.log(`moveForward was called.
Current rover position is x:${locationX} y:${locationY}
Current rover direction is ${roverDir} `);
}
};
console.log(turnRight(rover));
console.log(moveForward(rover));
console.log(moveForward(rover));
console.log(turnRight(rover))
console.log(moveForward(rover));
console.log(moveForward(rover));
1 Answer

KRIS NIKOLAISEN
54,971 PointsSome things to look at:
1) Your functions don't return anything so no need to use console.log here
console.log(turnRight(rover));
console.log(moveForward(rover));
console.log(moveForward(rover));
console.log(turnRight(rover))
console.log(moveForward(rover));
console.log(moveForward(rover));
That is why you see undefined in the console.
2) In function moveForward() you store theRover values in local variables, do some calculations, but never update theRover. So you aren't keeping track of the location changes for subsequent calls. I would eliminate the local variables (which means you can eliminate the parameter as well) since they seem like a lot of extra work. You also can move the console.log of the current position to the end of the function so it isn't repeated for every condition. So you'd have for example
else if (rover.direction === "E" && rover.location.x < 9) {
rover.location.x += 1;
}
RODRIGO MARTINEZ
5,912 PointsRODRIGO MARTINEZ
5,912 PointsThanks so much. That solved everything. Now I can go on with the remaining tasks for the challenge. Thanks again!!!