Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial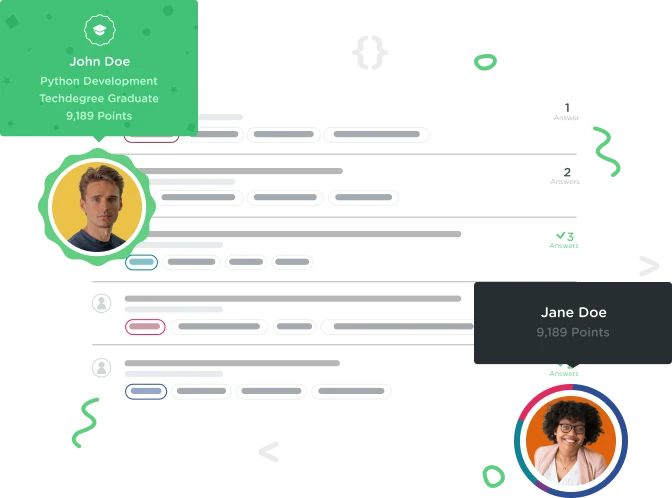

Uriah Nevins
1,121 PointsMember variable checker
I am trying to write a bit of code to check whether or not the given variable (fieldName) is the correct syntax for a member variable. If not, it should throw an Illegal Argument Exception. I have tried everything I can think of, but to no avail. Please help me get this Member variable Checker!
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
// These things should be verified:
// 1. Member fields must start with an 'm'
// 2. The second letter in the field name must be uppercased to ensure camel-casing
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
public char secondLetter(){
return fieldName.charAt(1);
}
if (fieldName.charAt(0) != m || !(Character.isUpperCase('secondLetter')) {
throw new IllegalArgumentException("Wrong Syntax.");
}
return fieldName;
}
}
2 Answers
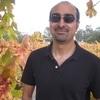
Kourosh Raeen
23,733 PointsIt looks like you've written a method to handle returning the second letter and there are a few problems with that.
1 - You've defined the secondLetter() method inside the validatedFieldName() method. Move it outside the validatedFieldName() method.
2 - You've put the call to secondLetter() inside single quotes. You need to remove those. You also need parenthesis after the call.
3 - The variable fieldName is not available inside the secondLetter() method. You need to pass that in. Also, you need to change the method's signature to let the compiler know that this method is accepting a String argument.
4 - Since you are calling the secondLetter() method from inside a static method you need to declare it as static as well.
5 - You need quotes around m.
6 - The if statement is missing a closing parenthesis.
Here's the code:
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
// These things should be verified:
// 1. Member fields must start with an 'm'
// 2. The second letter in the field name must be uppercased to ensure camel-casing
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
if (fieldName.charAt(0) != 'm' || !(Character.isUpperCase(secondLetter(fieldName)))) {
throw new IllegalArgumentException("Wrong Syntax.");
}
return fieldName;
}
public static char secondLetter(String fieldName){
return fieldName.charAt(1);
}
}

Uriah Nevins
1,121 PointsIf I had a dime for every time one of my questions has been answered by you, I would be rich. Thanks again Kourosh!
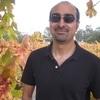
Kourosh Raeen
23,733 PointsHi Uriah - I'm glad to be of help. Happy coding!