Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial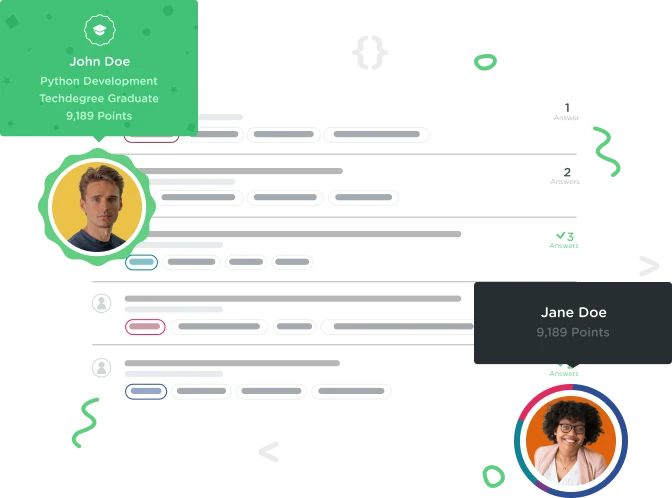
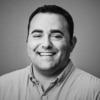
mikes02
Courses Plus Student 16,968 PointsMerge Twitter and Instagram API Results and Order by Date
I am using the https://github.com/J7mbo/twitter-api-php Twitter API PHP wrapper and the https://github.com/cosenary/Instagram-PHP-API#authenticate-user-oauth2 Instagram API PHP wrapper, both are functioning and displaying the results as I intended. However, what I was hoping to accomplish was merging both results and ordering them by date, newest to oldest, and displaying them in a masonry fashion. My PHP isn't the strongest so I am really just looking for guidance to get me on the right path. The code now is pretty basic, basically a couple of foreach loops, one for Instagram and one for Twitter a majority of the work is handled by the wrappers by passing in the appropriate keys/tokens.
2 Answers

Lewis Cowles
74,902 PointsHi Mike,
Well you have both lists right? You need to ensure you map them to have similar fields, and then depending upon the amount of data, you can
small data
- combine both arrays, then use usort with a simple callback
usort($socialfeed, function ($item1, $item2) {
return $item1['date_created'] - $item2['date_created'];
});
larger data-sets
- spin-up an in-memory SQLlite DB using PDO, read each of the data into the SQLite (requires some thinking about fields and mapping), then query using SQL, to sort by date (this will be immensely faster than PHP over large data-sets), and hey, you can cache the SQLite file to disk when done so you are not incurring the rate limiting features.
In any case it may actually be better to get PHP to simply hand-over the responses in two separate calls to the front-end as JSON or XML, and have the user's computer do the sorting saving you server-time.
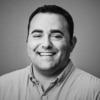
mikes02
Courses Plus Student 16,968 PointsThanks for the feedback. A co-worker actually helped me out a bit to get me on the right track. Using both PHP wrappers I linked to in the original post I was able to normalize both feeds so that it was easier to work with their data. Below is the code I am using to accomplish this, it is working well.
We start out with an empty $mergeSocial array, and then we have Tweets and Instagram, you can see that I created a type field so that I can do a conditional check to see which it is and style accordingly with my CSS. They share some similarities that were mapped to the same name, for example, created_at, url, text, but in cases where they differed you will see how I set those up. The Instagram feed has some conditional checks to make sure that a caption exists and that likes exist.
This is followed by a function to compare dates and then the $mergeSocial array and the dateSort function are supplied to usort to get the desired result.
You'll see that I am also using stdClass() in my code, this article defines it quite well:
"Sometimes all that is necessary is a property bag to throw key value pairs into. One way is to use array, but this requires quoting all keys. Another way is to use dynamic properties on an instance of StdClass. StdClass is a sparsely documented class in PHP which has no predefined members."
<?php
// Empty Merge Social Array
$mergeSocial = array();
// Normalize Tweets
foreach($jsonTweets as $tweet) {
$tweetObj = new stdClass();
$tweetObj->type = 'tweet';
$tweetObj->created_at = strtotime($tweet->created_at);
$tweetObj->url = $tweet->id_str;
$tweetObj->text = $tweet->text;
$tweetObj->name = $tweet->user->screen_name;
$mergeSocial[] = $tweetObj;
}
// Normalize Instagram
foreach($media->data as $instagram) {
$photoObj = new stdClass();
$photoObj->type = 'instagram';
$photoObj->created_at = $instagram->created_time;
$photoObj->url = $instagram->link;
$photoObj->img = $instagram->images->standard_resolution->url;
// Check for existence of caption text
if(isset($instagram->caption->text)) {
$photoObj->text = $instagram->caption->text;
}
// Check for likes
if(isset($instagram->likes->count) && $instagram->likes->count > 0) {
$photoObj->likes = $instagram->likes->count;
}
$mergeSocial[] = $photoObj;
}
// Organize by date
function dateSort($a, $b) {
return $b->created_at - $a->created_at;
}
// Sort Merged Array by Date Descending
usort($mergeSocial, "dateSort");
?>