Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial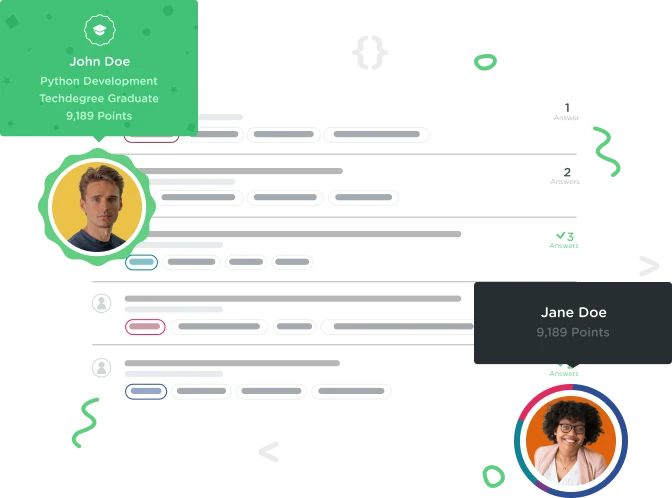
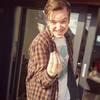
Michael Lauridsen
10,321 PointsMy attempt - did I brainfart too much?
Okay, so I tried to do this challenge without looking up the answer. Quite fun, but also though as I'm still shaky in the language of JavaScript.. well, any 'real' programming language. So please tell me if this is totally illogical:
var questions = [
['Hvilket band har lavet sangen Loud Pipes?', 'RATATAT'],
['Hvilken fransk duo bærer elektroniske masker?', 'DAFT PUNK'],
['Følgende band har bl.a. udgivet sangene "New Slang" & "Simple Song"', 'THE SHINS']
];
var answersCorrect = [];
var answersIncorrect = [];
var questionsCorrect = [];
var questionsIncorrect = [];
function result (questionsX, answersX){
for (var y = 0; y < questionsX.length; y++) {
var out = document.write('<b>' + questionsX[y] + '</b>' + ' ' + answersX[y].toUpperCase() + '<br>');
}
return out;
}
for (var i = 0; i < questions.length; i++) {
var answer = prompt(questions[i][0]);
if (answer.toUpperCase() === questions[i][1]) {
answersCorrect.push(answer);
questionsCorrect.push(questions[i][0]);
console.log('Question answered correctly');
} else {
answersIncorrect.push(answer);
questionsIncorrect.push(questions[i][0]);
console.log('Question answered incorrectly');
}
}
document.write('<h2>Rigtige svar: ' + answersCorrect.length + '</h2>' + '<br>');
result(questionsCorrect, answersCorrect);
document.write('<h2>Forkerte svar: ' + answersIncorrect.length + '</h2>' + '<br>');
result(questionsIncorrect, answersIncorrect);
2 Answers

Iain Simmons
Treehouse Moderator 32,305 PointsSo, a couple of things:
First, you don't really need separate arrays for the correctly answered questions and answers, just leave them grouped together in their own inner array and access the index you need when you want either the question or the answer from it.
Second, you don't need to use both document.write
*and * return
in the function, you should do one or the other. Personally I think you're better off constructing the result HTML and just returning it as a string, so you can use it for things other than document.write
(like updating an element's innerHTML
property, for example).
Then you would call your function within the document.write
call (the below assumes you changed the function to only use one input array argument):
document.write(result(correct));

James Bradd
4,597 PointsFor your questions I would have made them with keys, like
questions = [
{'question' : 'Hvilket band har lavet sangen Loud Pipes?',
'answer' : 'RATATAT'}
]
It would make it easier to work with later, so you could just do question.question and question.answer instead of looking through the array for it. Also means you could refactor your code later (say you add a difficulty, category or something of that nature) without worrying about changing the array index.
I'm curious though why are there "answers correct" and "questions correct" arrays?

Iain Simmons
Treehouse Moderator 32,305 PointsI think they do they in a later video/stage.
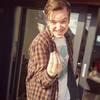
Michael Lauridsen
10,321 PointsAlright thanks! I haven't learnt about keys yet, but I will be sure to look it up. The questionscorrect and answerscorrect are because I wanted to keep track of the questions associated with the answer. But now that I think about, maybe it would have been easier with the array. Join method?
Michael Lauridsen
10,321 PointsMichael Lauridsen
10,321 PointsOh great ideas! My brain started to hurt at the time of questionscorrect and answerscorrect, but now that you planted the idea of using arrays, it all makes sense. Much easier and clean!
I have made both of your ideas work in the code, thanks a lot!