Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial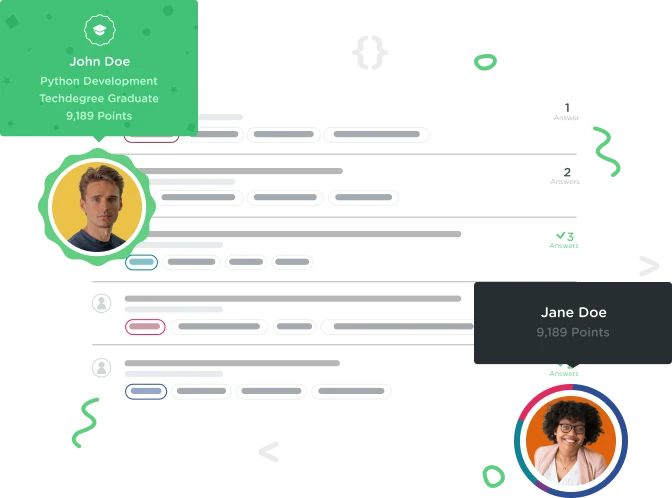
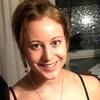
Ingrid Pettersson
9,916 PointsMy buttons don't work - why?
None of my buttons work. In the console there is no error message when I click on them, it is just no reaction at all. Here is my code:
From index.html:
<div class="list">
<ul>
<li>
<button class="button up">Up</button>
<button class="button down">Down</button>
<button class="button remove">Remove</button>Grapes
</li>
<li class="error-white">
<button class="button up">Up</button>
<button class="button down">Down</button>
<button class="button remove">Remove</button>Salt
</li>
<li class="error-white">
<button class="button up">Up</button>
<button class="button down">Down</button>
<button class="button remove">Remove</button>Snow
</li>
<li>
<button class="button up">Up</button>
<button class="button down">Down</button>
<button class="button remove">Remove</button>Amethyst
</li>
<li class="error-red">
<button class="button up">Up</button>
<button class="button down">Down</button>
<button class="button remove">Remove</button>African sunset
</li>
<li class="error-brown">
<button class="button up">Up</button>
<button class="button down">Down</button>
<button class="button remove">Remove</button>Cinnamon
</li>
<li>
<button class="button up">Up</button>
<button class="button down">Down</button>
<button class="button remove">Remove</button>Lavender
</li>
<li class="error-red">
<button class="button up">Up</button>
<button class="button down">Down</button>
<button class="button remove">Remove</button>Fire trucks
</li>
<li>
<button class="button up">Up</button>
<button class="button down">Down</button>
<button class="button remove">Remove</button>Plums
</li>
</ul>
</div><!--/.list-->
From app.js:
const list = document.querySelector('.list');
list.addEventListener('click', (event) =>
{
if (event.target.tagName == 'BUTTON')
{
if (event.target.className == 'remove')
{
let li = event.target.parentNode;
let ul = li.parentNode;
ul.removeChild(li);
}
if (event.target.className == 'up') {
let li = event.target.parentNode;
let prevLi = li.previousElementSibling;
let ul = li.parentNode;
ul.insertBefore(li, prevLi);
}
if (event.target.className == 'down') {
let li = event.target.parentNode;
let nextLi = li.nextElementSibling;
let ul = li.parentNode;
ul.insertBefore(nextLi, li);
}
}
});
It's the last code of the file and the code snippet above it works well and ends properly with }); .
It's a long code/question, I know, and I would be sooo glad for a bit of help here!
1 Answer
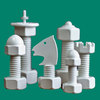
Steven Parker
231,140 PointsNone of the tests match because the items have two classes, and the tests are checking for an exact match with just one. There are several ways to fix this:
- remove the extra HTML class
<button class="up">Up</button>
- check for a match with the full class set
event.target.className == 'button up'
- use a function to check an individual class
event.target.classList.contains('up')
These will all work in this particular instance, but the third solution is the most robust as it will continue to work if additional classes are added in the future.
Ingrid Pettersson
9,916 PointsIngrid Pettersson
9,916 PointsThank you Steven! I wouldn't have figured that out myself. I chose the second solution for now and it works perfect - wow!