Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial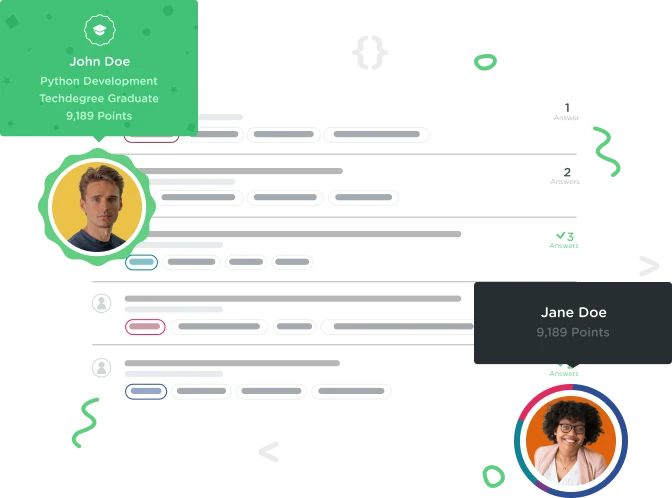

Karl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 PointsMy code has no red lines anymore but its not running, thank you in advance for your help
/***
* `quotes` array
***/
// Empty array
//[]
// Empty object
//{}
// Objects are made up of comma separated pairs of
// properties and values
//{
//propertyOne: valueOne,
//propertyTwo: valueTwo,
//propertyThree: valueThree
//}
//ANSWER:
var quotes = [
{
quote: 'Don’t raise your voice, improve your argument.',
source: 'Harvey Specter',
citation: 'Suits (American TV series)',
year: '2011'
},
{
quote: 'Never destroy anyone in public when you can accomplish the same result in private.',
source: 'Harvey Specter',
citation: 'Suits (American TV series)',
year: '2012'
},
{
quote: 'Let them hate. Just make sure they spell your name right.',
source: 'Harvey Specter',
citation: 'Suits (American TV series)',
year: '2013'
},
{
quote: 'People respond to how we’re dressed, so like it or not this is what you have to do.',
source: 'Harvey Specter',
citation: 'Suits (American TV series)',
year: '2014'
},
{
quote: "Sometimes good guys gotta do bad things to make the bad guys pay.",
source: 'Harvey Specter',
citation: 'Suits (American TV series)',
year: '2011'
}
];
/***
* `getRandomQuote` function
***/
//function getRandomQuote() {
// 1. Create a variable that generates a random number
// between zero and the last index in the `quotes` array
// 2. Use the random number variable and bracket notation
// to grab a random object from the `quotes` array, and
// store it in a variable
// 3. Return the variable storing the random quote object
//}
//ANSWER:
function getRandomQuote(array) {
var quoteIndex = Math.floor(Math.random() * (quotes.length));
for (var i = 0; i < array.length; i++) {
var randomQuote = array[quoteIndex];
}
return randomQuote;
}
/***
* `printQuote` function
***/
//function printQuote() {
// 1. Create a variable that calls the getRandomQuote()
// function
// 2. Create a variable that initiates your HTML string with
// the first two <p></p> elements, their classNames,
// and the quote and source properties, but leave off
// the second closing `</p>` tag for now
// 3. Use an if statement to check if the citation property
// exists, and if it does, concatenate a <span></span>
// element, appropriate className, and citation property
// to the HTML string
// 4. Use an if statement to check of the year property exists,
// and if it does, concatenate a <span></span> element,
// appropriate className, and year property to the HTML
//string
// Basic if statement
//if (/* your condition */) {
// Code to run if your condition evaluates to true
//}
//<p class="quote">quote text</p>
//<p class="source">quote source
//<span class="citation">quote citation</span>
//<span class="year">quote year</span>
//</p>
//<p class="quote">quote text</p>
//<p class="source">quote source</p>
//ANSWER:
function printQuote() {
console.log("clicked")
var actualQuote = getRandomQuote(quotes);
var stringOfQuoteProperties = "";
stringOfQuoteProperties += "<p class='quote'>" + actualQuote.quote +"</p> <p class='source'>" + actualQuote.source + " " + actualQuote.year + "</p>"
if (actualQuote.year.hasOwnProperty()) {
stringOfQuoteProperties += "<span class='year'>" + actualQuote.year + "</span>";
} else {}
document.getElementById("quote-box").innerHTML = stringOfQuoteProperties;
}
/***
* click event listener for the print quote button
* DO NOT CHANGE THE CODE BELOW!!
//ANSWER:
***/
//document.getElementById('quote-box').innerHTML = yourStringHere;
document.getElementById('load-quote').addEventListener("click", printQuote, false);

Karl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 PointsBrandon White ok sir no problem, i got it working :)
2 Answers
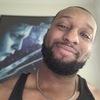
Brandon White
Full Stack JavaScript Techdegree Graduate 34,662 PointsHi Karl Russell Sumando Menil,
I subbed your script in with the html for JS Tech Degree project #1, and the browser informed me that in this line of your code: document.getElementById('load-quote').addEventListener("click", printQuote, false);
document.getElementById('load-quote') was returning null
.
Unless the html file has changed since when I first coded this project, the element you're targeting doesn't have an ID of "load-quote", it has an ID of "loadQuote". Once I made that change, your code worked just fine.
If that's the issue you're having I'll offer a friendly reminder that when you come across a line in your Tech Degree studies that says "DO NOT CHANGE THE CODE BELOW", don't change it. They've put that there for a reason.
Also, in the future questions that deal with techdegree projects might get more response in the slack channel for your techdegree. Assuming the issue is with the naming of load-quote instead of loadQuote, that's a bug that only someone who's doing or has done the techdegree could help you with (because one would only know about that typing error if they have access to the html file that goes with the script).
Just something to be mindful of.
Great job with coding the functionality. Keep it up, coder!

Karl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 PointsGood day, hello Mr. Brandon White the original code is supposed to be " document.getElementById('load-quote').addEventListener("click", printQuote, false); ", for my project how do i change the ID from loadQuote to "load-quote"? thank you in advance for your help.
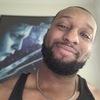
Brandon White
Full Stack JavaScript Techdegree Graduate 34,662 PointsYou don't. If you haven't changed the code, then my advice is to check your browser console to see if you're getting any error messages. If so, use those error messages to figure out why your code isn't running properly.
If that doesn't work (and even if it does), try reaching out to your Slack group. Lee, and Robert, and all the other moderators and champions there are great and super willing to help out.
I don't see any real issues with your code. In your getRandomQuote function you've hard coded the quotes.length
to the quoteIndex variable which tightly couples this code with this particular app, but that's not affecting your current functionality (and as you move forward you'll learn why that's not the best of practices). And in the for loop for the same function you're creating the var randomQuote in the loop's block scope, and using/calling it outside of that scope (and that's also something you'll learn about as you move forward), but it too did not affect your code running for me.
Have you made sure that the file is linked to the html file? If your script file isn't linked to your index.html(?), then your js code won't run. Also, if you've maybe set the wrong path for the link to your script file, your code won't run.
When you get a minute, post a link to your repo here, and I'll take a look.

Karl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 PointsMr.Brandon White hey um, this is awesome i deleted it, and then downloaded my repo again, i think its the read.md that i need to run the program, so um, i have one more question, can i submit this now? because the instructions said to get the peer review before submitting? or an approval? thank you for your response.

Karl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 PointsGood day thank you for your favourable response Mr.Brandon White this is the link to my repo: https://github.com/KMenil/RQG
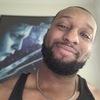
Brandon White
Full Stack JavaScript Techdegree Graduate 34,662 PointsI just downloaded your code, Karl, and it's running fine for me. When I click "show another quote", another quote appears on the screen. What happens for you when you run your code?

Karl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 PointsBrandon White so sir you mean it runs for you? thats great news maybe my computer has a problem, do you think i can submit it for grading? is the grades final? thank you for your response.
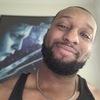
Brandon White
Full Stack JavaScript Techdegree Graduate 34,662 PointsThat's not really a question I can answer for you, Karl. I can't be sure of the criteria anymore (so I don't know that you've met all of them). All I know is that when I clicked the button to show another quote, I got another quote.
That said, I would not submit my own project to be graded unless it ran properly when I tested it.
Karl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 PointsKarl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 PointsMr.Brandon White Thank you for your response i have managed to get most of it done, but i have one problem, i do not have an "if else" statement please see link for any hints and suggestion: https://github.com/KMenil/RQG, also please tell me if its okay to submit already thank you. again apologies for the inconvenience and trouble that I have caused you.