Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial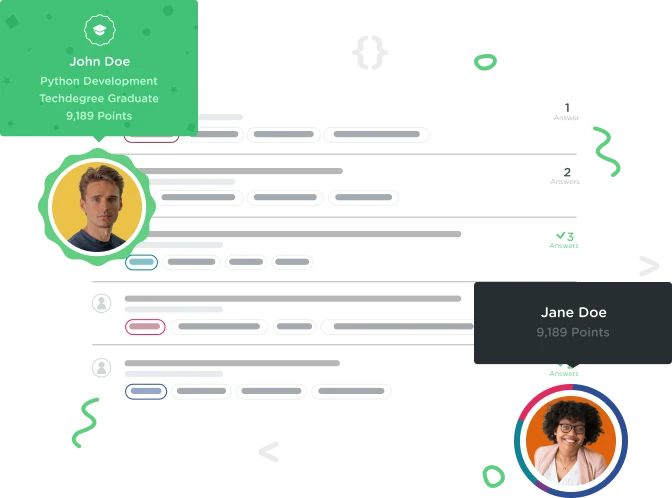
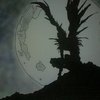
Lucas Santos
19,315 PointsmySQL Question
Quick question using mySQL.
My Code:
<?php
$servername = "localhost";
$username = "root";
$password = "root";
$dbname = "oopdb";
// Create connection
$conn = new mysqli($servername, $username, $password, $dbname);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
$sql = "SELECT * FROM boxes";
$result = $conn->query($sql);
if ($result->num_rows > 0) {
//output data of each row
while($row = $result->fetch_assoc()) {
echo "id: " . $row["id"] . " " . $row['firstname'] . '<br />';
}
} else {
echo "0 results";
}
// Here is where I delete a row with the id of 2
$deleteJohn = "DELETE FROM boxes WHERE id = 2";
if ($conn->query($deleteJohn) === TRUE) {
echo "row deleted successfully";
} else {
echo "Error deleting row: " . $conn->error;
}
$conn->close();
?>
Question:
Where you see me delete a row from my boxes table do I need to keep the actual code in my files because it seems that when I save the row with the id of 2 gets deleted and even if I remove my code the row is still deleted meaning whats the point in keeping that in your code rite?
Also another quick question on the matter of organizing mySQL code. If I wanted to do multiple SQL calls like delete then add a row then delete another then change a row would I do it all in the same block of code where I establish the connection to my database before the $conn->close();
or would I open another php tag and separate all of those SQL Queries with new database connections?
If someone with experience can explain id really appreciate it. Thank you!
1 Answer
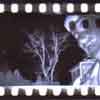
John Breslin
16,963 PointsNo, if you've deleted what you want you don't need to keep the code. Unless you want to keep checking and deleting a query every time you load. Actually, for one time deletes like this, it's better to use phpmyadmin or via the terminal. If they do it like this in the lesson, it's probably just so you know how.
I would connect to the database once, as connecting/disconnecting will probably slow things down a bit.
Lucas Santos
19,315 PointsLucas Santos
19,315 PointsOk I see so I would just probably include the mySQL code in a file and include that in my main php file.