Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial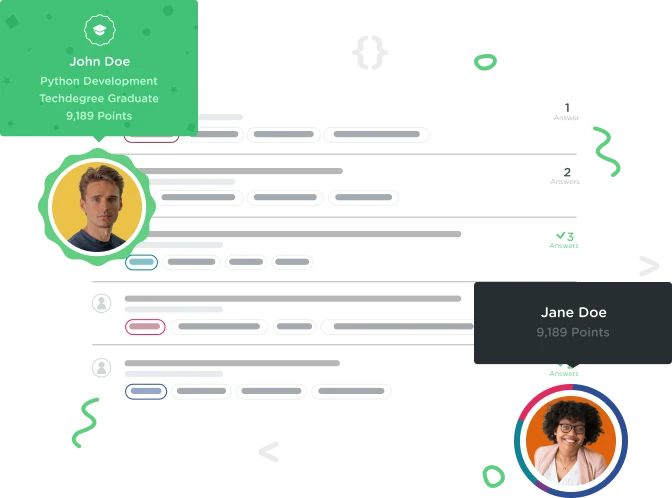

Abe Daniels
Courses Plus Student 2,781 PointsNeed help either converting to string, or changing my constructor type.
"Alright so now let's focus on ForumPost. It's going to need a constructor that takes a User author a String title and a String description."
So I am having 1 error pop up telling me that author = mAuthor isn't possible because one is a string and the other isn't. If i try to change the constructor type. (public post (blahblahblah) opposed to (public String post(blahblahblah) my error log shows a bunch more issues.
I am wondering exactly how I am supposed to get (User author) to be defined in my constructor. To me String title and String description make complete sense, and I understand that User author can't technically work, (with the code I have) I just need to know how to fix that.
public class ForumPost {
private User mAuthor;
private String mTitle;
private String mDescription;
public User getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getDescription() {
return mDescription;
}
public String post(User author, String title, String description) {
author = mAuthor;
title = mTitle;
description = mDescription;
return author;
return title;
return description;
}
// TODO: We need to expose the description
}
public class User {
private String mFirstName;
private String mLastName;
public User(String firstName, String lastName) {
// TODO: Set the private fields here
mFirstName = firstName;
mLastName = lastName;
}
public String getFirstName() {
return mFirstName;
}
public String getLastName() {
return mLastName;
}
}
public class Forum {
private String mTopic;
public Forum (String topic) {
mTopic = topic;
}
public String getTopic() {
return mTopic;
}
public void addPost(ForumPost post) {
/*
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
*/
}
}
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
// Forum forum = new Forum("Java");
// Take the first two elements passed args
// User author = new User();
// Add the author, title and description
// ForumPost post = new ForumPost();
// forum.addPost(post);
}
}
3 Answers
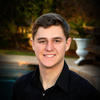
Colton Ehrman
Courses Plus Student 5,859 PointsAlso, if you wish to keep author as type User, then you could utilize User constructor and do something along the lines of
author = new User(mAuthor.getFirstName(), mAuthor.getLastName());
Again, this is javascript, not sure how to do in Java

Seth Kroger
56,413 PointsFirst, a constructor always has the same name as the class. Second, constructors don't have a return type (not even void).

Abe Daniels
Courses Plus Student 2,781 PointsThat's what I thought! I continually got errors expressing that I NEEDED a return type though. That thoroughly threw me off.
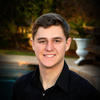
Colton Ehrman
Courses Plus Student 5,859 PointsSo really, I do not know Java, but it looks like you are assigning a variable with a class type to another variable with a class type, which doesn't work in C++. What you could do is, change author to a type of String, and then access the two properties (firstName, lastName) from the variable mAuthor, by using the getter methods and do
author = mAuthor's firstName + mAuthor's lastName
Does this make sense? The code I have is psuedo and wouldn't be what you would actually code, but just the idea.
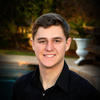
Colton Ehrman
Courses Plus Student 5,859 PointsAssuming you are familiar with javascript the code would look like
author = mAuthor.getFirstName() + " " + mAuthor.getLastName();

Abe Daniels
Courses Plus Student 2,781 PointsThis does make sense and I am going to play with it a little more. Thanks for the input!

Abe Daniels
Courses Plus Student 2,781 PointsYES that makes perfect sense.
Abe Daniels
Courses Plus Student 2,781 PointsAbe Daniels
Courses Plus Student 2,781 PointsThis was the key thing that lit the lightbulb. Thank you.
Colton Ehrman
Courses Plus Student 5,859 PointsColton Ehrman
Courses Plus Student 5,859 PointsLol no problem glad to help :)