Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial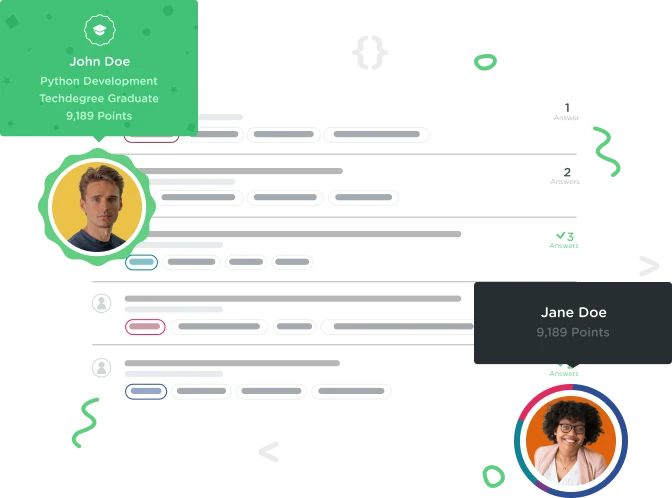

John Fouts
12,234 PointsNeed help understanding where I've gone wrong with this code. Rec. a msg. saying that I may be missing an assembly ref.
Please help me understand where I have mis-stepped with this code. I am receiving a message saying that lastName does not exist, and that I may be missing an assembly reference...
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
namespace Treehouse.CodeChallenges
{
public class Course
{
public Course()
{
Students = new List<CourseStudent>();
}
public int Id { get; set; }
public int TeacherId { get; set; }
[Required, StringLength(200)]
public string Title { get; set; }
public string Description { get; set; }
public int Length { get; set; }
public Teacher Teacher { get; set; }
public ICollection<CourseStudent> Students { get; set; }
}
}
using System.Collections.Generic;
using System.Data.Entity;
using System.Linq;
namespace Treehouse.CodeChallenges
{
public static class Repository
{
public static List<Course> GetCourses()
{
using (var context = new Context())
{
return context.Courses.ToList();
}
}
public static List<Course> GetCoursesByTeacher(string lastName)
{
using (var context = new Context())
{
var Courses = context.Courses
.Where(ct=>ct.lastName==Teacher.lastName)
.ToList();
return Courses;
}
return null; // TODO
}
}
}
namespace Treehouse.CodeChallenges
{
public class Teacher
{
public int Id { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
}
}
2 Answers
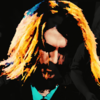
Justin Horner
Treehouse Guest TeacherHello John,
The challenge is asking for you to find course teachers. Since the Course model does not define the last name of the teacher, you need to access the last name of the teacher from the Teacher property.
ct => ct.Teacher.LastName == lastName
Also, you can remove return null; // TODO as it will not be reachable due to the first return statement.
I hope this helps.

John Fouts
12,234 PointsThank you Justin. I had ordered my Lambda expression that way the first time, but I had written it like this.... ct=>ct.LastName == lastName...so the code had failed because I had neglected to explicitly identify the Teacher property.
So I switched around the sides of the equality expression before I sent my code in which of course did not work, but after seeing your explanation, I have more clarity. Thank you for your help!
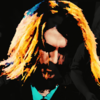
Justin Horner
Treehouse Guest TeacherYou're welcome!
Ole Vølund Skov Mortensen
27,842 PointsOle Vølund Skov Mortensen
27,842 Pointsjust a quickie question: how am I to interpret "ct"? in the course he used. I might have forgotten but where did I set "cb" or "ct" to be what I associate with something else??