Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial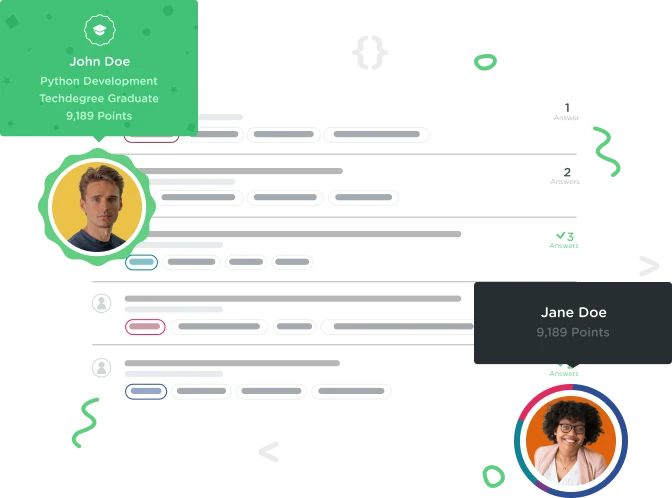

Luke Strama
6,928 PointsNeed help with bug in personal project
I'm working on an app that will calculate the available Tax Free Savings Account room for an individual based on their year of birth. It's similar to an IRA in the States I believe...
TFSAActivity.java
package com.example.straml1.tfsacalculator.ui;
import android.content.Intent;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.widget.EditText;
import com.example.straml1.tfsacalculator.R;
import com.example.straml1.tfsacalculator.model.Calculator;
public class TFSAActivity extends AppCompatActivity {
public static final String TAG = TFSAActivity.class.getSimpleName();
private EditText tfsaValue;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_tfsa);
tfsaValue = findViewById(R.id.tfsaValue);
Intent intent = getIntent();
String year = intent.getStringExtra(getString(R.string.key_year));
if (year == null || year.isEmpty()) {
year = "Before 1992";
}
Log.d(TAG, year);
showResult(year);
}
private void showResult(String year) {
Calculator calculator = new Calculator();
int answer = calculator.limitByAge(year);
tfsaValue.setText(String.valueOf(answer));
}
}
Calculator.java
package com.example.straml1.tfsacalculator.model;
import java.util.Arrays;
public class Calculator {
// TFSA contributions up to 2018
private int[] yearlyLimit = {5000, 5000, 5000, 5000, 5500, 5500, 10000, 5500, 5500, 5500};
public int limitByAge(String year) {
int roomTotal = 0;
if (year == "Before 1992") {
for (int num : yearlyLimit) {
roomTotal += num;
}
}
else {
int yearBorn = Integer.parseInt(year);
int yearsToSubtract = 18 - (2009 - yearBorn);
int[] validContributions = Arrays.copyOfRange(yearlyLimit, 0+yearsToSubtract, yearlyLimit.length);
for (int num : validContributions) {
roomTotal += num;
}
}
return roomTotal;
}
}
strings.xml
<resources>
<string name="app_name">TFSA Calculator</string>
<string name="key_year">year</string>
<string name="year_born">What year were you born in?</string>
<string-array name="year_born_array">
<item> </item>
<item>Before 1992</item>
<item>1992</item>
<item>1993</item>
<item>1994</item>
<item>1995</item>
<item>1996</item>
<item>1997</item>
<item>1998</item>
<item>1999</item>
<item>2000</item>
</string-array>
</resources>
The main idea is the user selects for a drop down menu (as seen in the xml file) and then it spits out the contribution room they have available based on what year they are born (as seen in Calculator.java).
The bug happens when the 'Before 1992' is selected. Instead of being caught by the 'if' statement, it skips to the 'else' part of the block and then throws an exception when it can't be parsed into an int (obviously). The weird part of it is that when the default blank value is selected, it gets assigned the 'Before 1992' value (as seen in TFSAActivity.java) and gets caught by the 'if' portion of the loop like intended. Everything else goes off like it is supposed to...
Any help is much appreciated!
Ps. There's a MainActivity.java that I didn't include as I don't think it's relevant here but will if need be.
1 Answer

Seth Kroger
56,413 PointsThe equality of Strings should be checked with .equals(), not == or !=. The latter two don't check the values of a non-primitive Object. They check they are the exact same Object in memory. String.equals() on the other hand is overridden to check for equal values.
Luke Strama
6,928 PointsLuke Strama
6,928 PointsPerfect, that was exactly it, thanks!