Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial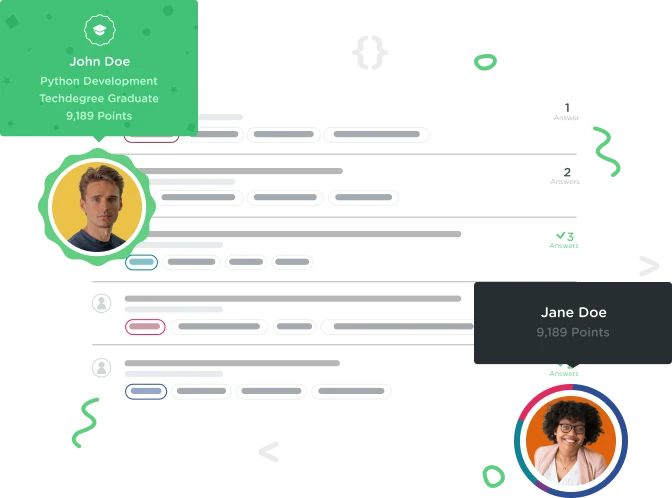
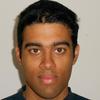
Rohit Gopalan
81,945 PointsNeed help with code challenge task 2 of 2: For looping in PHP Arrays and Control Structures
Here is the following code:
<?php
$facts = array(
57 => ' on Heinz ketchup bottles represents the number of varieties of pickles the company once had.',
2 => ' is the approximate hours a day Giraffes sleeps',
18 => ' is the average hours a Python sleeps per day',
10 => ' per cent of the world is left-handed.',
11 => ' Empire State Buildings, stacked one on top of the other, would be required to measure the Gulf of Mexico at its deepest point.',
98 => '% of the atoms in your body are replaced every year',
69 => ' is the largest number of recorded children born to one woman',
);
//add your loop below this line
for($i=1; $i<=100; $i++) {
echo $i;
if(isset($facts[$i])) {
echo " ".$facts[$i]." ";
}
}
?>
I am getting the error: Make sure the fact is displayed directly after the number.
I am unsure about what is wrong with this code. Could someone please let me know how to correct it?
16 Answers
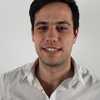
Juan Ignacio Lambardi
3,943 Points<?php
$facts = array(
57 => ' on Heinz ketchup bottles represents the number of varieties of pickles the company once had.',
2 => ' is the approximate hours a day Giraffes sleeps',
18 => ' is the average hours a Python sleeps per day',
10 => ' per cent of the world is left-handed.',
11 => ' Empire State Buildings, stacked one on top of the other, would be required to measure the Gulf of Mexico at its deepest point.',
98 => '% of the atoms in your body are replaced every year',
69 => ' is the largest number of recorded children born to one woman',
);
//add your loop below this line
for ( $i = 1; $i <= 100; $i++ ) {
echo $i;
if (isset($facts[$i])) {
echo $facts[$i] . "<br />\n";
}
}

Steve Berrill
20,016 Pointsi didnt like the way the text was outputted after the numbers so i changed to this.
i added if $facts is not set then add a break. much cleaner
<?php
$facts = array(
57 => ' on Heinz ketchup bottles represents the number of varieties of pickles the company once had.',
2 => ' is the approximate hours a day Giraffes sleeps',
18 => ' is the average hours a Python sleeps per day',
10 => ' per cent of the world is left-handed.',
11 => ' Empire State Buildings, stacked one on top of the other, would be required to measure the Gulf of Mexico at its deepest point.',
98 => '% of the atoms in your body are replaced every year',
69 => ' is the largest number of recorded children born to one woman',
);
//add your loop below this line
for ($i = 1; $i <= 100; $i++){
echo $i;
if(!isset($facts[$i])) {
echo "<br />\n";
}
if(isset($facts[$i])){
echo $facts[$i] . "<br />\n";
}
}
?>
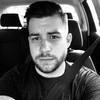
Szabolcs Szána
6,961 Pointshi,
please write the task text too beacuse i don't understand now :) i'd do with foreach when i want to print array.

Santis Smagars
980 PointsDon't understand how $i can become a key of an $facts array - $facts[$i]- I solved 2part only because I simply copy paste from here because i would never figure out on my own that $i should be written as a key to $facts and that I shouldn't specify a key for each case when I need to echo the sentence. I was trying many variations like - if(isset($facts) && ($i == 57)) {echo $facts[57];} and other variations which I guess is very stupid and of course it did not work. But I just want to understand which part of leraning so far i missed. So far i have re-watched every video like 3 times for the whole course and I still could not figure out this on my own
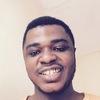
Mohammed Bashiru
Courses Plus Student 4,816 PointsJust note that $i is only a variable holding an incremented value from the loop condition.
inside the variable is an incremented value (integer) between 1 to 100 so let's assume after the first condition. $i is now holding an integer value of 2
then if(isset($facts[$i])){ echo $facts[$i]; }
so assume in-place of the $i is the value 2 which makes the code if(isset($facts[2])){ echo $facts[2]; } Making "2" the reference key to the document in the array.
Hope this helps.

Johnathan Guzman
7,432 PointsI found that this best outputs the desired results.
<?php
$facts = array(
57 => ' on Heinz ketchup bottles represents the number of varieties of pickles the company once had.',
2 => ' is the approximate hours a day Giraffes sleeps',
18 => ' is the average hours a Python sleeps per day',
10 => ' per cent of the world is left-handed.',
11 => ' Empire State Buildings, stacked one on top of the other, would be required to measure the Gulf of Mexico at its deepest point.',
98 => '% of the atoms in your body are replaced every year',
69 => ' is the largest number of recorded children born to one woman',
);
for ($i = 0; ++$i <= 100;) {
echo $i;
if (isset($facts[$i])) {
echo $facts[$i] . "\n";
} if (!isset($facts[$i])) {
echo "\n";
}
}

Duncan Michael-MacGregor
15,204 PointsTidiest way I have found.
<?php
$facts = array(
57 => ' on Heinz ketchup bottles represents the number of varieties of pickles the company once had.',
2 => ' is the approximate hours a day Giraffes sleeps',
18 => ' is the average hours a Python sleeps per day',
10 => ' per cent of the world is left-handed.',
11 => ' Empire State Buildings, stacked one on top of the other, would be required to measure the Gulf of Mexico at its deepest point.',
98 => '% of the atoms in your body are replaced every year',
69 => ' is the largest number of recorded children born to one woman',
);
//add your loop below this line
for ($i = 1; $i <= 100; $i++) {
echo $i;
if(isset($facts[$i])){
echo $facts[$i] . "<br />\n";
} else {
echo "<br />\n";
}
}
?>
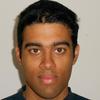
Rohit Gopalan
81,945 PointsOops sorry about that. The task text is the following:
Use the function isset to test if the incremented value equals one of the keys in the $facts array. If there is a key that matches, display the value after the number.

Jason Anello
Courses Plus Student 94,610 PointsHi Rohit,
The extra spaces that you're echoing before and after the fact might be throwing off the challenge tester.
Try to echo the fact only.
echo $facts[$i];

Phillip Legault
Courses Plus Student 18,888 Points<?php
$facts = array(
57 => ' on Heinz ketchup bottles represents the number of varieties of pickles the company once had.',
2 => ' is the approximate hours a day Giraffes sleeps',
18 => ' is the average hours a Python sleeps per day',
10 => ' per cent of the world is left-handed.',
11 => ' Empire State Buildings, stacked one on top of the other, would be required to measure the Gulf of Mexico at its deepest point.',
98 => '% of the atoms in your body are replaced every year',
69 => ' is the largest number of recorded children born to one woman',
);
//add your loop below this line
for($i=1; $i<=100; $i++) {
echo $i;
if(isset($facts[$i])) {
echo $facts[$i] ;
}
}
?>
This does not work for me, tried a lot of other variations and nothing. Any help?

Jason Anello
Courses Plus Student 94,610 PointsMaybe try again.
Your code is working for me.

Phillip Legault
Courses Plus Student 18,888 PointsOK that is just odd I tested this, it does work now

Phillip Legault
Courses Plus Student 18,888 PointsIn the for loop you are setting i as a variable $i=1 and the the $i <=100 is saying you only want it to go to 100 when you are adding 1 each time with the i++.
if(isset($facts[$i])) {
echo $facts[$i] ;
//starts $i=1
}
if(isset($facts[$i])) {
echo $facts[$i] ;
//then $i=2
}
if(isset($facts[$i])) {
echo $facts[$i] ;
//then $i=3
}
if(isset($facts[$i])) {
echo $facts[$i] ;
//then $i=4
}
etc. until it $i=100 then the for loop ends and goes on the next actions in your php file.
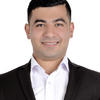
Samandar Mirzayev
11,834 Points<?php
$facts = array(
57 => ' on Heinz ketchup bottles represents the number of varieties of pickles the company once had.',
2 => ' is the approximate hours a day Giraffes sleeps',
18 => ' is the average hours a Python sleeps per day',
10 => ' per cent of the world is left-handed.',
11 => ' Empire State Buildings, stacked one on top of the other, would be required to measure the Gulf of Mexico at its deepest point.',
98 => '% of the atoms in your body are replaced every year',
69 => ' is the largest number of recorded children born to one woman',
);
//add your loop below this line
for($i=1; $i<=100; $i++ ){
echo $i;
if(isset($facts[$i])){
echo $facts[$i];
}
echo "<br />\n";
}
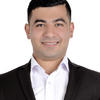
Samandar Mirzayev
11,834 Points<?php $facts = array( 57 => ' on Heinz ketchup bottles represents the number of varieties of pickles the company once had.', 2 => ' is the approximate hours a day Giraffes sleeps', 18 => ' is the average hours a Python sleeps per day', 10 => ' per cent of the world is left-handed.', 11 => ' Empire State Buildings, stacked one on top of the other, would be required to measure the Gulf of Mexico at its deepest point.', 98 => '% of the atoms in your body are replaced every year', 69 => ' is the largest number of recorded children born to one woman', );
//add your loop below this line
for($i=1; $i<=100; $i++ ){ echo $i; if(isset($facts[$i])){ echo $facts[$i]; } echo "<br />\n"; }
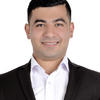
Samandar Mirzayev
11,834 Pointsshould help this guys
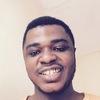
Mohammed Bashiru
Courses Plus Student 4,816 PointsThe question was wrong. Try the code below. It should work
<?php $facts = array( 57 => ' on Heinz ketchup bottles represents the number of varieties of pickles the company once had.', 2 => ' is the approximate hours a day Giraffes sleeps', 18 => ' is the average hours a Python sleeps per day', 10 => ' per cent of the world is left-handed.', 11 => ' Empire State Buildings, stacked one on top of the other, would be required to measure the Gulf of Mexico at its deepest point.', 98 => '% of the atoms in your body are replaced every year', 69 => ' is the largest number of recorded children born to one woman', ); //add your loop below this line for ($i = 1; $i < 101; ++$i ){ echo $i . "\n";
if(isset($facts[$i])){ echo $facts[$i]; } echo "<br />\n"; }
?>
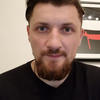
Igor Pavlenko
12,925 Pointshey guys i did it this way and it still works
for ($i = 2; $i < 99; $i++) { if(isset($facts)){ echo "$facts[$i]" ; }
do you need to add [$i] in the if statement ?
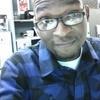
Ezell Frazier
22,110 PointsI found a ternary useful for this challenge.
for ($count = 1; $count <= 100; $count ++) {
echo $count . (isset($facts[$count]) ? $facts[$count] : NULL) . "\n";
}
Juan Ignacio Lambardi
3,943 PointsJuan Ignacio Lambardi
3,943 PointsHi Rohit, This should work! :)