Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial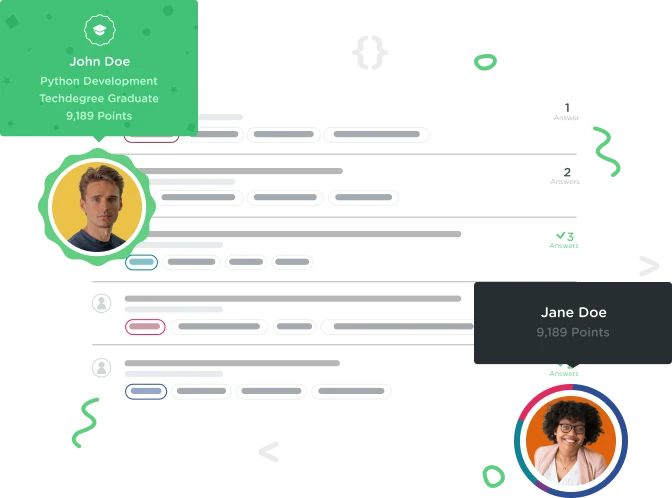

Raymond Yam
Courses Plus Student 345 PointsNeed help with getting the right result
I am getting the result of too cold for temperature 21, and compiler comes back with all three results of just right and too hot in a single run. Can someone help me with this issue?
string input = Console.ReadLine();
int temperature = int.Parse(input);
if (temperature >= 20);
{
Console.WriteLine("Too cold!");
}
if(temperature == 21);
{
Console.WriteLine("Just right.");
}
if(temperature <= 22);
{
Console.WriteLine("Too hot!");
}
2 Answers
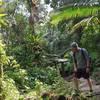
Christian Mateo
6,320 PointsThere are a couple of things I see wrong here:
- You are placing semicolons “;” at the end of each conditional “if()”, semicolons are only to be used at the end of expressions.
- The logic seams a little odd to me. Regarding to temperature reading, the high the number the warmer it should be. The lower the number the colder it should be. So the logic in any case should be: (temperature <= 20) = “Too Cold” and (temperature >= 22) = “Too Hot”. Between 20 and 22 is the sweet spot (21).
So the code should look like this:
string input = Console.ReadLine();
int temperature = int.Parse(input);
if(temperature <= 20)
Console.WriteLine("Too cold!");
else if(temperature >= 22)
Console.WriteLine("Too hot!");
else
Console.WriteLine("Just right");
Console.ReadKey();
Note:
- I didn't include curly brackets "{}" after reach conditional "if()" because only a single line of code follows. This is only optional.
- I included "Console.ReadKey();" so the console would not close.
Cheers! Happy Coding!
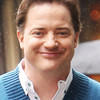
Matthew Loveday
342 Points string input = Console.ReadLine();
int temperature = int.Parse(input);
if (temperature <= 20)
{
Console.WriteLine("too cold");
}
else if (temperature == 21)
{
Console.WriteLine("just right");
}
else if (temperature >= 22)
{
Console.WriteLine("Too hot");
}