Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial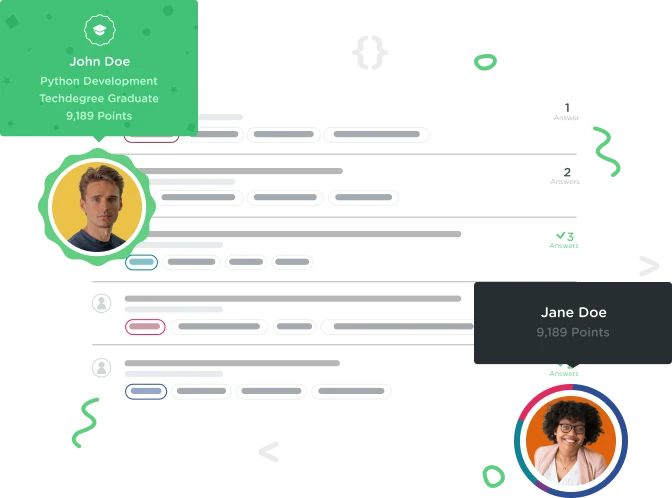

Bruce Gordon
6,850 PointsNeed help with last exercise in Java Objects - how to make objects work together
This exercise uses 4 classes: Forum, User, ForumPost and Example. I don't really understand how to get the classes to talk to each other. The challenge is to get Example to work correctly which posts an author, title and description.
The Forum class requires a topic and prints out the author's first name, last name and title derived from the other classes.
The User class requires a first and last name.
The ForumPost class requires an author which is type User, which I don't understand, a title and a description.
I don't understand how the first and last name that are in the User class relates to the author which is type User.
I tried using the code in Example which I attached, but it doesn't compile because the ForumPost class requires a User type value.
public class ForumPost {
private User mAuthor;
private String mTitle;
private String mDescription;
public ForumPost(User author, String title, String description) {
mAuthor = author;
mTitle = title;
mDescription = description;
}
public String getDescription() {
return mDescription;
}
public User getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
// TODO: We need to expose the description
}
public class User {
private String mFirstName;
private String mLastName;
public User(String firstName, String lastName) {
// TODO: Set the private fields here
mFirstName = firstName;
mLastName = lastName;
}
public String getFirstName() {
return mFirstName;
}
public String getLastName() {
return mLastName;
}
}
public class Forum {
private String mTopic;
public Forum(String topic) {
mTopic = topic;
}
public String getTopic() {
return mTopic;
}
public void addPost(ForumPost post) {
// When all is ready uncomment this...
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
}
}
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
Forum forum = new Forum("Java");
// Take the first two elements passed args
User author = new User("Bruce","Gordon");
// Add the author, title and description
ForumPost post = new ForumPost(author.getFirstName() + author.getLastName(),forum.getTopic(),"Help with Java");
forum.addPost(post);
}
}
5 Answers

Bruce Gordon
6,850 PointsI understand the concept, but I have trouble translating the requirements of the challenge questions into something I recognize. Trying to decipher somebody else's code is like reverse engineering, which is easier to do when you have a good foundation of the concepts.
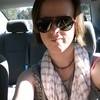
karis hutcheson
7,036 PointsHe wants to be able to pass his name into Example(args) and have that be set as the author. Remember that our String[] args can take any number of arguments and give them to us in an indexed list so (according to his comment) he wants to pass in his name to Example(String[] args) as firstName lastName. So instead of using your name, instantiate the User object with:
User author = new User(args[0], args[1]);
Also note that the ForumPost object initialization takes a User object for the author. So you would pass in author rather than the string concatenation that is currently being passed:
ForumPost post = new ForumPost(author, forum.getTopic(), "Help with Java");
I think the rest should work out fine?

Bruce Gordon
6,850 PointsHere is what I have so far (see below): I don't get a compiler error, but the output reads:
Starting forum example... New post from Craig Dennis about Java.
The message from the "Bummer" is: I expected to see the names I passed in through the args, but I do not. Hmm.
Forum forum = new Forum("Java"); //Take the first two elements passed args User author = new User("Craig","Dennis"); //Add the author, title and description ForumPost post = new ForumPost(author,forum.getTopic(),"Help with Java"); forum.addPost(post);
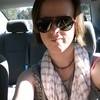
karis hutcheson
7,036 PointsLike I said above, change your new User() initiation to read: new User(args[0], args[1]); So it will pass those args in from the function call.

Bruce Gordon
6,850 PointsKaris,. Thanks for your help. It's going to take me some time to look at code and try to figure out what it is doing.
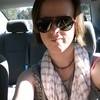
karis hutcheson
7,036 PointsNo prob :) I'm not great at explaining things, either. So that doesn't help.

Bruce Gordon
6,850 PointsFor me I think that rather than hardcoding a lot of stuff inside an object constructor in the examples, it would have been better to start the course with explaining that the user interface always starts with main that accepts an array of arguments that is used by all of the objects.
So for example, instead of Treet treet = new Treat("craigdennis","Want to be Famous?",new Date(123123123123123L)) it would have been better to say args[0] = "craigdennis"; args[1] = "Want to be Famous?"; args[2] = 12312312312312312312L Treet treet = new Treat(args[0],args[1],args[2]);
This problem would have taken me only a minute or two to solve if I had understood these concepts from the beginning.
Thanks again for helping me to get past the halfway point in the course.

Craig Dennis
Treehouse TeacherHi Bruce!
Do you understand that those args are passed in from the command line right?
Check out this video around 1:45 on.
That make more sense?