Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial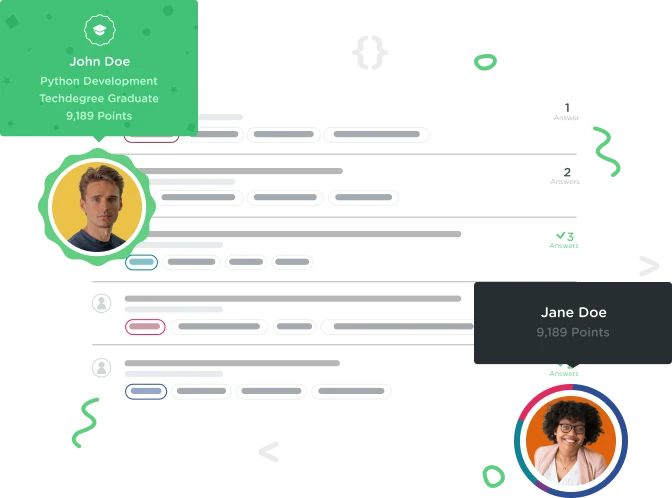
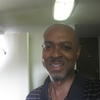
trell story
1,520 PointsNeed some help on this one
Not getting this one.
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
String readConsole;
Console.Write("Enter the number of times to print \"Yay!\": ");
result = Console.ReadLine()
Convert.ToInt32(readConsole);
int number;
Console.WriteLine(number = "Yay!");
}
}
}
1 Answer

Keli'i Martin
8,227 PointsDan Henning pointed out pretty much all the issues with your code in his comment. In summary:
-
readConsole
doesn't have a value, so the callConvert.ToInt32(readConsole)
will cause an exception. -
result
is never defined, so compiler error. -
number
is defined as anint
and cannot be assigned a string value.
On top of these issues, there are some missing elements, namely a loop. So let's start from the top.
First, it appears that you intended to use readConsole
where you actually used result
. That is easily fixed by replacing result
with readConsole
.
Now, as the instructions hint at, you will need a loop in order to accomplish the task of printing out "Yay!" the specified number of times. You could either use a for loop here or a while loop. I would recommend using a for loop, but a while loop will also work; you would just need to remember to increment the counter variable inside the loop.
Here is just a snippet of what the for loop would look like:
for (int i = 0; i < number; i++)
{
Console.WriteLine("Yay!");
}
You will also want to assign the variable number
with the return of the Convert.ToInt32(readConsole)
call. In other words:
int number = Convert.ToInt32(readConsole);
Hope this helps!
Dan Henning
8,691 PointsDan Henning
8,691 PointsThe code example looks like it will fail. "readConsole" has not been initialized with a value, so the static method "Convert.ToInt32()" will throw an exception. "Console.ReadLine()" expects user input, but "result" has not been declared properly, and it will not compile. "number" has been declared properly, but you cannot assign a string value to an integer.
I hope that helps, Dan