Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial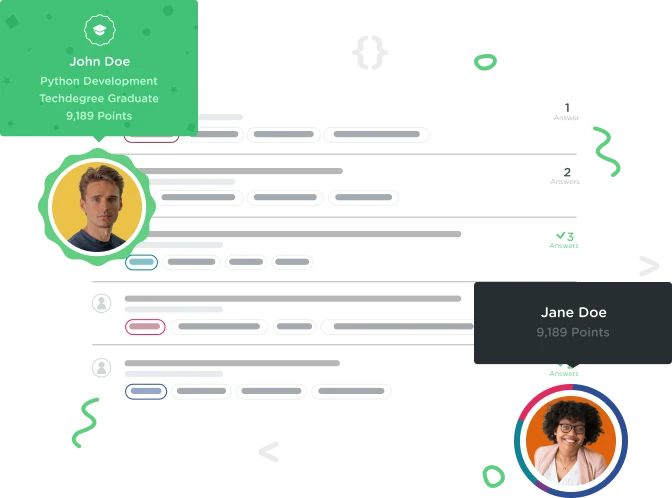

Kimberly Lu
2,861 PointsNest array multiplication table
I'm getting this error message "Object reference not set to an instance of an object." I checked StackOverflow and it suggests I did not declare my array correctly. I re-watched the video and still can't see the problem. Any advice? I'm new to Treehouse, so please let me know if you can't see my code.
namespace Treehouse.CodeChallenges
{
public static class MathHelpers
{
public static int[][] BuildMultiplicationTable(int maxFactor)
{
int[][] multTable = new int[maxFactor+1][];
for(int rowFactor = 0; rowFactor <= maxFactor; rowFactor++)
{
for (int colFactor =0; colFactor<= maxFactor; colFactor++)
{
multTable[rowFactor][colFactor] = rowFactor * colFactor;
}
}
return multTable;
}
}
}
1 Answer

andren
28,558 PointsThe issue is that you have not set the nested array to an instance, only the outer array.
Basically when you call this code:
int[][] multTable = new int[maxFactor+1][];
You create an array of size (maxFactor+1), and each entry in that array contains an object. But that object is not by default an array itself, it is simply null
. Meaning you have to set it to an instance before you use it.
In your code you try to assign something to that object before actually assigning an array to it. Basically you just need to add this line:
multTable[rowFactor] = new int[maxFactor+1];
At the start of your loop, in order to actually make the object stored in the array an array. Which is what leads you to have an array nested under an array.
Like this:
namespace Treehouse.CodeChallenges
{
public static class MathHelpers
{
public static int[][] BuildMultiplicationTable(int maxFactor)
{
int[][] multTable = new int[maxFactor+1][];
for(int rowFactor = 0; rowFactor <= maxFactor; rowFactor++)
{
multTable[rowFactor] = new int[maxFactor+1];
for (int colFactor =0; colFactor <= maxFactor; colFactor++)
{
multTable[rowFactor][colFactor] = rowFactor * colFactor;
}
}
return multTable;
}
}
}
Kimberly Lu
2,861 PointsKimberly Lu
2,861 PointsThank you! That's much clearer than the video. I was under the wrong impression that by just using the 2 sets of brackets, I've "instantiated" an array within an array, so I didn't think I needed to do more. But you're right. I've only created an outer array to store objects, but didn't say what those objects would be (in this case arrays).