Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial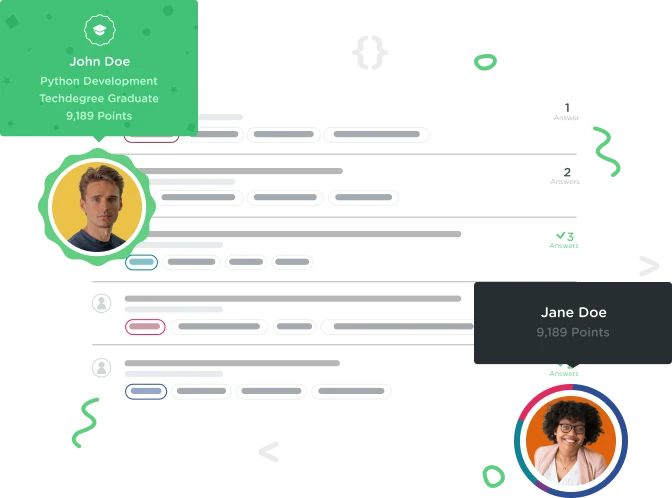

Derek Vha
10,452 Points.NetCore MVC - Creating a ViewModel from two Models
Hi,
So in my basic application, I have two Models - Member and MembershipType.
I am trying to create a view model that combines certain properties from the above two models, and displays that on my Index page.
However I am struggling to understand what exactly I need to do in my Index action method, in order to get the data, as i also use EntityFramework in order to read the data from the database:
public class Member
{
public int Id { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
public DateTime DateOfBirth { get; set; }
// foreign key for MembershipType
[ForeignKey("MembershipType")]
public int MembershipTypeId { get; set; }
}
public class MembersController : Controller
{
private readonly GymContext _context;
public MembersController(GymContext context)
{
_context = context;
}
// GET: Members
public async Task<IActionResult> Index()
{
List<MemberViewModel> memberViewModel = new List<MemberViewModel>(); //declared my VIewModel instance
//link the viewModel instance to the context class somehow..
return View(await _context.Members.ToListAsync());
}
} }
public class GymContext : DbContext
{
public GymContext(DbContextOptions<GymContext> options) : base(options)
{
}
public DbSet<Member> Members { get; set; }
public DbSet<MembershipType> MembershipTypes { get; set; }
}
public class MemberViewModel
{
public string FirstName { get; set; }
public string LastName { get; set; }
public DateTime DateOfBirth { get; set; }
public string PaymentType { get; set; }
}
Above is my Member class and my index action method, as well as the view model class I have created. I am not sure how exactly to get pass the values of the view model into the view.
Any ideas?
Thanks
1 Answer
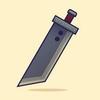
Allan Clark
10,810 PointsSo first I would add the navigation property to your Member class. You have the id so it is linked but that will just give you the id value not the whole MembershipType object. So Member should look like this:
public class Member
{
public int Id { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
public DateTime DateOfBirth { get; set; }
// foreign key for MembershipType
[ForeignKey("MembershipType")]
public int MembershipTypeId { get; set; }
public MembershipType MembershipType { get;set; }
}
Really once you have that you don't really need a view model. The Member object will have access to all the data you require (assuming the MembershipType class holds the 'PaymentType' the view model calls for). So your controller action would be very simple:
public async Task<IActionResult> Index()
{
return View(await _context.Members.ToListAsync());
}
Then it will be up to Razor on your front end to access the data and put it in the correct position. For example:
@model.MembershipType.PaymentType