Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial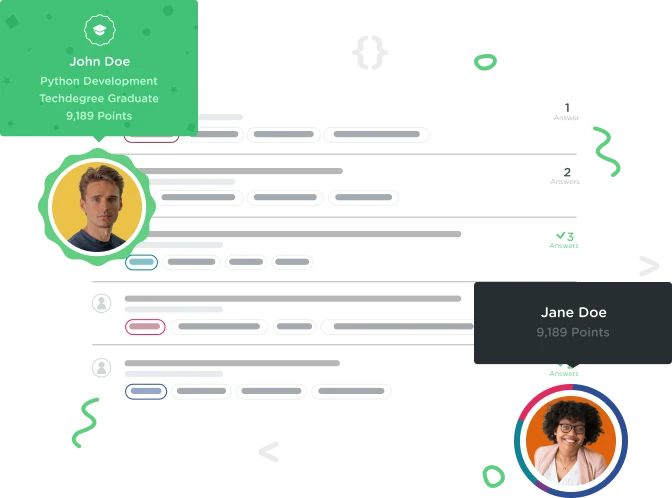

Adrian Reszka
4,555 Pointsno idea... last task in c# basics
someone, please help me understand the issue. Works in Visual Studio...
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
bool check = true;
while (check)
{
try
{
Console.Write("Enter the number of times to print \"Yay!\": ");
string numofyays = Console.ReadLine();
int yays = int.Parse(numofyays);
if (yays < 0)
{
Console.WriteLine("You must enter a positive number.");
continue;
}
for (int i = 0; i < yays; i++)
{
Console.WriteLine("Yay!");
}
check = false;
}
catch (FormatException)
{
Console.WriteLine("FU");
continue;
}
}
}
}
}
Bummer! System.ArgumentNullException: Value cannot be null. Parameter name: String. See output for stack trace.
System.ArgumentNullException: Value cannot be null. Parameter name: String at System.Number.StringToNumber (System.String str, NumberStyles options, System.NumberBuffer& number, System.Globalization.NumberFormatInfo info, Boolean parseDecimal) [0x00054] in /builddir/build/BUILD/mono-4.4.2/external/referencesource/mscorlib/system/number.cs:1074 at System.Number.ParseInt32 (System.String s, NumberStyles style, System.Globalization.NumberFormatInfo info) [0x00014] in /builddir/build/BUILD/mono-4.4.2/external/referencesource/mscorlib/system/number.cs:745 at System.Int32.Parse (System.String s) [0x00000] in /builddir/build/BUILD/mono-4.4.2/external/referencesource/mscorlib/system/int32.cs:120 at Treehouse.CodeChallenges.Program.Main () <0x40cd2f10 + 0x00067> in :0 at MonoTester.Run () [0x00197] in MonoTester.cs:125 at MonoTester.Main (System.String[] args) [0x00013] in MonoTester.cs:28
3 Answers

Harrison Court
4,232 PointsI finally got it! If you would like the full working thing, do this:
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
try
{
Console.Write("Enter the number of times to print \"Yay!\": ");
// Get the input string and convert it to an int using int.Parse
int timesToRun = int.Parse(Console.ReadLine());
// declare a counter variable that will be used to keep track of the number of times the loop has ran
int counter = 0;
if (counter <= 0)
{
Console.WriteLine("You must enter a positive number.");
}
else
{
// Print "Yay!"
Console.Write("Yay!");
// Increase the counter variable by 1
counter++;
}
// Run the loop while the counter is less than the timesToRun variable
while (counter < timesToRun)
{
// Print "Yay!"
Console.Write("Yay!");
// Increase the counter variable by 1
counter++;
}
}
catch (FormatException)
{
Console.WriteLine("You must enter a whole number.");
}
}
}
}
After this, it should work fine.
P.S: I don't know why the text is almost orange.

Stephan Olsen
6,650 PointsThere's nothing wrong with your code really, it works great. I had the same problem as you when I was completing the task some weeks ago. The reason it gives you an error, is because you're wrapping it all in a while loop, and prompting the user again if he enters a negative number. The task only asks you to write a message if it's a negative number however. So basically you don't need the while loop - although you most likely would use one in a real scenario.
try
{
Console.Write("Enter the number of times to print \"Yay!\": ");
string numofyays = Console.ReadLine();
int yays = int.Parse(numofyays);
if (yays < 0)
{
Console.WriteLine("You must enter a positive number.");
}
for (int i = 0; i < yays; i++)
{
Console.WriteLine("Yay!");
}
}
catch (FormatException)
{
Console.WriteLine("You must enter a whole number");
}

Harrison Court
4,232 PointsI'm still getting the exact error.

Geoffrey Arnold
2,948 PointsI had the same issue as your, Harry, because I anticipated the test inputs to continue inputting something until it inputted an acceptable input (that's a lot of inputs, sorry). In short, I also had a "continue;" in the input validation for negative numbers, which breaks the programs since the tester doesn't input something after that. All you need to do to pass the test, as Stephen indicated, is change the "continue;" to a "break;" so that your program doesn't expect to receive another input.