Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial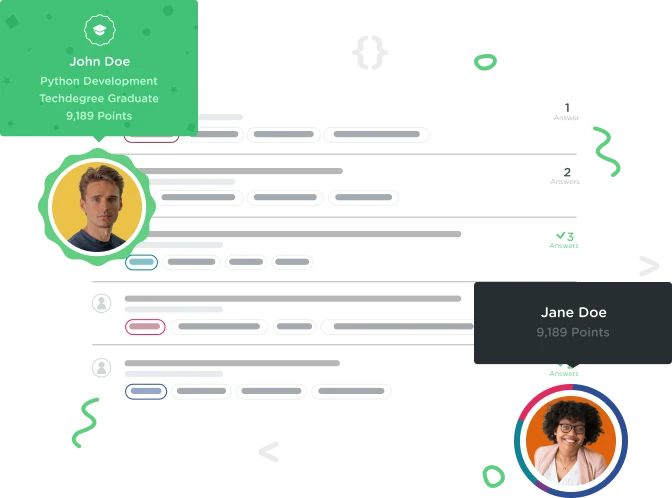

Doug C
Courses Plus Student 1,594 PointsNo sure what step 2 is asking here? Please help.
I am having trouble understanding the very basics of what this question is asking me to do. In particular I don't get how one would set the value of a variable to the results of calling the function. Doesn't the the variable need to have a value before returning the function?
Here is the code I started with:
function returnValue( doug ) { var echo = return doug; }
Thanks for any help.
function returnValue( doug ) {
var echo =
return doug;
}
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
3 Answers
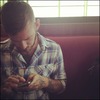
Erik McClintock
45,783 PointsDoug,
For task two, they want you to:
1) Create a function called returnValue that takes one argument and then simply returns it.
2) Create a variable called "echo" outside of the function.
3) Assign as the value of that variable the result of calling the returnValue function.
Let's break it down into smaller steps and look at what's going on as we go!
First, we have our returnValue function:
function returnValue( myArg ) {
return myArg;
}
This function isn't very useful, but that's beside the point. This function will simply take in whatever argument you pass it, and then return it. You can think of that as meaning that, when you call this function, it is going to equal whatever the value it returns is set to. In this particular case, it returns the argument you pass into it, so if you pass "waffles" into it, then the result of calling this function will be the string "waffles."
Now, we are told to create a variable "echo" outside of the function.
function returnValue( myArg ) {
return myArg;
}
var echo;
Here, we have created a new variable in the global scope, and we've called it "echo". It is currently undefined, so we need to fix that by assigning it a value. The value that the task wants it to have assigned to it is whatever value is returned from the "returnValue" function, which we just learned is going to be whatever the argument is that you pass into it. That means, that if we do something like the following:
function returnValue( myArg ) {
return myArg;
}
var echo = returnValue( "The Empire Strikes Back" );
...then "myArg" inside your function is equal to "The Empire Strikes Back," which the function will then return (i.e. "be equal to", in a sense), and since we're assigning that function call to be the value of our echo variable, then the value that gets stored into our "echo" variable is going to be "The Empire Strikes Back"! Again, this kind of illustrates how useless that function is, since we could just as easily assign the value of "The Empire Strikes Back" to the echo variable directly in this case (since the function is doing nothing with that value), but still. This challenge helps to show you how functions and return values work.
Hopefully this has helped to make the process a little more manageable and sensible. Happy coding!
Erik
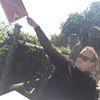
Dennis Klarenbeek
17,168 PointsCan you please enter the objective of this challenge?
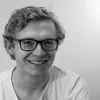
Luke Glazebrook
13,564 PointsHi Doug!
If you set the variable echo equal to your parameter of doug, whatever you pass into the function will then be set to equal echo.
For example, if you called the function as follows then the word "Hello!" will be returned.
function returnValue(word)
{
var echo = word;
return echo;
}
returnValue("Hello!");
I hope that I managed to help you out here. Try and apply the information that I have just given to you to the challenge and see if you can get it all working. Think about, as well, how you could return the value without having to set a variable.
-Luke
Doug C
Courses Plus Student 1,594 PointsDoug C
Courses Plus Student 1,594 PointsThis is for task 2, specifically.