Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial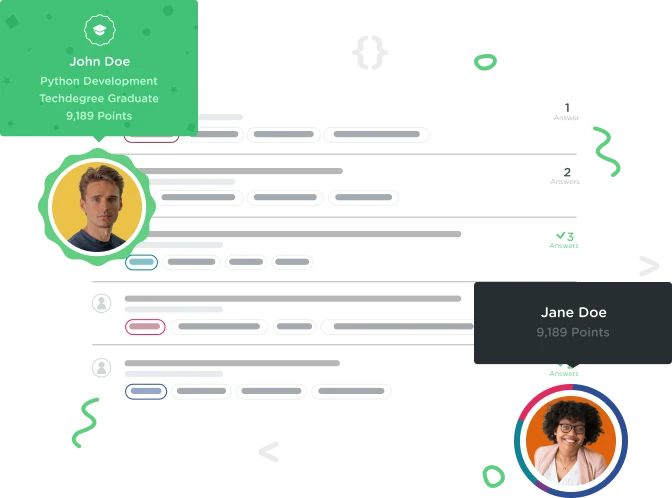
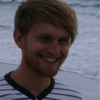
Daniel Schwemmlein
4,960 Pointsnot getting any better with the java challenge
Still stuck at the last challenge of java objects. tried some code again but of course it would not work
public class ForumPost {
private User mAuthor;
private String mTitle;
private String mDescription;
public User getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
// TODO: We need to expose the description
}
public class User {
private mSomeName; //what type do we need? What name would be more appropriate?
private mAnotherName;
public User(String firstName, String lastName) {
// TODO: Set the private fields here
}
public getSomeName() {
return mSomeName
}
}
public class Forum {
private String mTopic;
public String getTopic() {
return mTopic;
}
public void addPost(ForumPost post) {
/* When all is ready uncomment this...
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
*/
}
}
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
// Forum forum = new Forum("Java");
// Take the first two elements passed args
// User author = new User();
// Add the author, title and description
// ForumPost post = new ForumPost();
// forum.addPost(post);
}
}
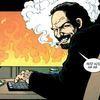
Greg Kitchin
31,522 PointsIt's been a while since I've done this one, but for the user class...
The second two lines are part of the constructor, setting up variables. You need to decide what type of variables they're going to be.
The fourth line, is the beginning of the code setting up methods. Most methods have a return type,. If they don't then 'void' is written as part of the code. In this case, you want to write public 'theReturnType' User (// rest of the code)
It might help to think of the code in blocks, that you stack in a pattern to get the desired effect. To declare methods, I used in my notes... accessLevel returnTypeOrVoid methodName (any arguments) { }
You can set established variables to be the same as another variable, if they are the same type (or you might need to do casting).
String myName = "Greg"; String anotherName = ""; anotherName = myName;
And now anotherName also has "Greg" as it's value.
1 Answer
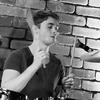
Ollie Prentice
11,685 PointsHi Daniel,
So the parameters have already been written in the constructor - firstName, lastName.
However, the strings mentioned have not been declared. You have written two private variables but have not stated that they are Strings.
instead of naming mSomeName/mAnotherName, it would be clearer to write mFirstName/mLastName as shown in the constructor.
Example:
private String mFirstName;
The next step is to initialise the variables in the constructor, e.g. give them a value. The value is the string defined in the parameters of the constructor.
Below is an example of how a declared String could be initialised (change the names to suit your code).
mFastCar = fastCar;
Then all you need to do is add a getter. You're close with your attempt, but you have forgotten String. also remember to add ; at the end of appropriate lines. You need to add getters for both parameters.
I hope this clears some of the problems. If you are still stuck, drop another message and I'll help you with the correct answer.
Daniel Schwemmlein
4,960 PointsDaniel Schwemmlein
4,960 Pointsi'm stuck at user.java where i have to add private fields and a getter