Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial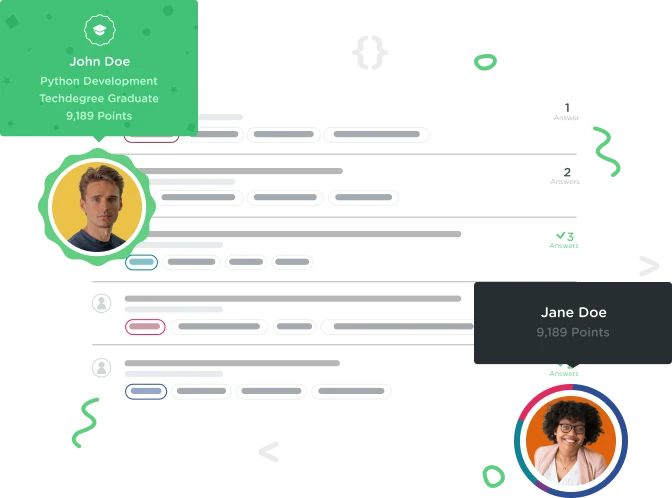

Greg P
Full Stack JavaScript Techdegree Student 1,133 PointsNot sure where my error is
I am unable to locate the issue with my code when trying to append. I receive:
"app.js:26 Uncaught TypeError: Cannot read property 'value' of null at HTMLButtonElement.<anonymous> (app.js:26)"
const toggleList = document.getElementById('toggleList');
const listDiv = document.querySelector('.list');
const descriptionInput = document.querySelector('input.description');
const descriptionP = document.querySelector('p.description');
const descriptionButton = document.querySelector('button.description');
const addItemInput = document.querySelector('input.addItemInput');
const addItemButton = document.querySelector('button.addItemButton');
toggleList.addEventListener('click', () => {
if (listDiv.style.display == 'none') {
toggleList.textContent = 'Hide list';
listDiv.style.display = 'block'
} else {
toggleList.textContent = 'Show list';
listDiv.style.display = 'none';
}
});
descriptionButton.addEventListener('click', () => {
descriptionP.innerHTML = descriptionInput.value + ':';
});
addItemButton.addEventListener('click', () => {
let ul = document.getElementsByTagName('ul')[0];
let li = document.createElement('li');
li.textContent = addItemInput.value;
ul.appendChild(li);
});
this has been driving me crazy for maybe over half an hour. :-(
2 Answers
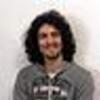
Gergely Bocz
14,244 PointsAlright, I have found the problem in your code.
In the JS this line tries to select an element with the class "addItemInput" and there is no such element :
const addItemInput = document.querySelector('input.addItemInput');
So i rewrote this part of your HTML:
<input type="text" class="description">
To this:
<input type="text" class="addItemInput">
So the whole HTML looks like this:
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<h1 id="myHeading">JavaScript and the DOM</h1>
<p>Making a web page interactive</p>
<button id="toggleList">Hide list</button>
<div class ="list">
<p class="description">Things that are purple:</p>
<input type="text" class="description">
<button class="description">Change list description</button>
<ul>
<li>grapes</li>
<li>amethyst</li>
<li>lavender</li>
<li>plums</li>
</ul>
<input type="text" class="addItemInput">
<button class="addItemButton">Add item</button>
<button class="removeItemButton">Remove last button</button>
</div>
<script src="app.js"></script>
</body>
</html>
I figured it out by checking out why this JS line "li.textContent = addItemInput.value;" has a null value. I moved forward with checking the queryselector "const addItemInput = document.querySelector('input.addItemInput');". If you check it out in the dev tools console (F12 in most browsers), you can see that the variable "addItemInput" has a null value. If you ever run into such a problem again, the console is a great tool to help you with debugging!
If something is not clear, feel free to ask me!
Good luck!
Gergő
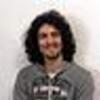
Gergely Bocz
14,244 PointsHi Greg P!
Can you please share your html code aswell? I want to see what is it that you are trying to select.

Greg P
Full Stack JavaScript Techdegree Student 1,133 PointsThis is from the video in https://teamtreehouse.com/library/appending-nodes
My HTML below
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<h1 id="myHeading">JavaScript and the DOM</h1>
<p>Making a web page interactive</p>
<button id="toggleList">Hide list</button>
<div class ="list">
<p class="description">Things that are purple:</p>
<input type="text" class="description">
<button class="description">Change list description</button>
<ul>
<li>grapes</li>
<li>amethyst</li>
<li>lavender</li>
<li>plums</li>
</ul>
<input type="text" class="description">
<button class="addItemButton">Add item</button>
<button class="removeItemButton">Remove last button</button>
</div>
<script src="app.js"></script>
</body>
</html>
Greg P
Full Stack JavaScript Techdegree Student 1,133 PointsGreg P
Full Stack JavaScript Techdegree Student 1,133 PointsWhat confuses me is that same line is still used in Guil's video with no issues and continues for several after as well.
But thank you.