Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial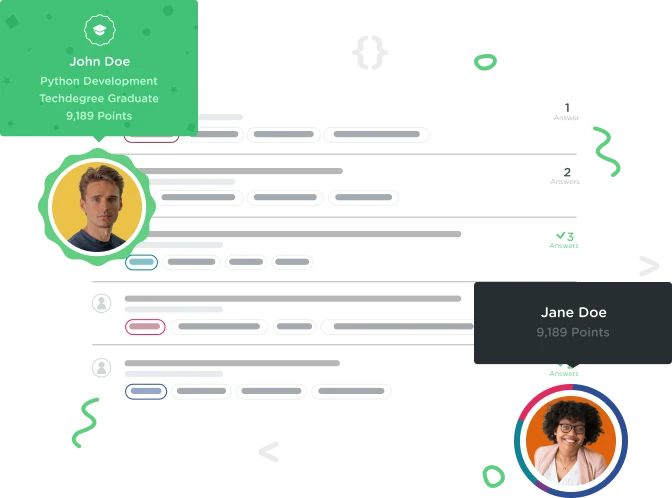

Kenneth Phillips
Courses Plus Student 10,188 PointsNotification keeps repeating
I already have published an app to the Google Play Store called SubjectOfThought. In the app I publish a daily topic on something interesting about science, technology, or history. Every day I have the app set to send a notification at 8:00 am because that is when I update the topic. However I didn't notice until now that the notification will repeat several times after this because I put the method in the onCreate Method. So how would I set notifications to repeat daily without sending one every time the user opens the app?
Here is my code for my activities.
MainActivity:
package com.subjectofthought.subjectofthought;
import android.app.AlarmManager;
import android.app.PendingIntent;
import android.content.Intent;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.webkit.WebSettings;
import android.webkit.WebView;
import android.webkit.WebViewClient;
import java.util.Calendar;
public class MainActivity extends AppCompatActivity {
private WebView webview;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
webview = (WebView) findViewById(R.id.myWebView);
WebSettings webSettings = webview.getSettings();
webSettings.setJavaScriptEnabled(true);
webview.loadUrl("http://opensourceco.com/");
webview.setWebViewClient(new WebViewClient());
setNotification();
}
public void setNotification() {
Calendar calendar = Calendar.getInstance();
calendar.set(calendar.HOUR_OF_DAY, 8);
calendar.set(calendar.MINUTE, 1);
Intent intent = new Intent(getApplicationContext(), Notification_receiver.class);
PendingIntent pendingIntent = PendingIntent.getBroadcast(getApplicationContext(), 100, intent, PendingIntent.FLAG_CANCEL_CURRENT);
AlarmManager alarmManager = (AlarmManager) getSystemService(ALARM_SERVICE);
alarmManager.setRepeating(AlarmManager.RTC_WAKEUP, calendar.getTimeInMillis(), AlarmManager.INTERVAL_DAY, pendingIntent);
}
}
Broadcast Receiver:
package com.subjectofthought.subjectofthought;
import android.app.NotificationManager;
import android.app.PendingIntent;
import android.content.BroadcastReceiver;
import android.content.Context;
import android.content.Intent;
import android.support.v4.app.NotificationCompat;
/**
* Created by gener on 2/1/2018.
*/
public class Notification_receiver extends BroadcastReceiver{
@Override
public void onReceive(Context context, Intent intent) {
NotificationManager notificationManager = (NotificationManager) context.getSystemService(Context.NOTIFICATION_SERVICE);
Intent repeating_intent = new Intent(context, MainActivity.class);
repeating_intent.setFlags(Intent.FLAG_ACTIVITY_CLEAR_TOP);
PendingIntent pendingIntent = PendingIntent.getActivity(context, 100, repeating_intent, PendingIntent.FLAG_UPDATE_CURRENT);
NotificationCompat.Builder builder = new NotificationCompat.Builder(context).setContentIntent(pendingIntent)
.setSmallIcon(R.mipmap.ic_launcher)
.setContentTitle("SubjectOfThought")
.setContentText("New Topic for today")
.setAutoCancel(true);
notificationManager.notify(100, builder.build());
}
}
PS: I apologize that I didn't just put this on GitHub. I'm having issues with it and the app is small so I just put it on here.
1 Answer

Seth Kroger
56,413 PointsLet answer this with a question. What time does setNotification() set an alarm for if the app is opened before 8 am verses after 8 am on any particular day?
Kenneth Phillips
Courses Plus Student 10,188 PointsKenneth Phillips
Courses Plus Student 10,188 PointsRight I know it will trigger after someone opens it because I put it in the onCreate() method. So how would I trigger this process once and have it keep running without doing that every time someone opens the app?