Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial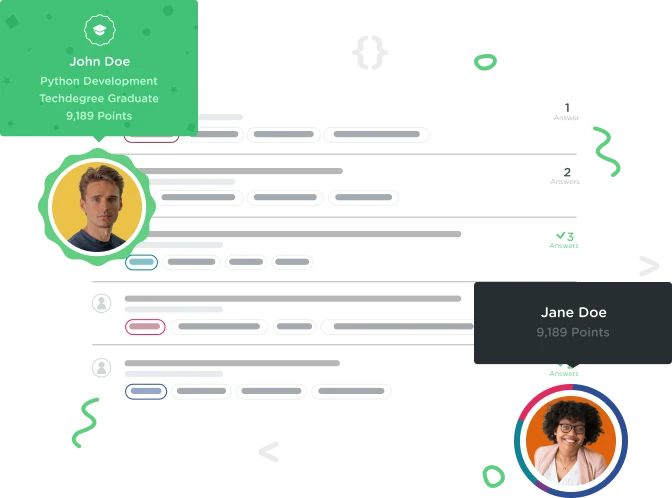
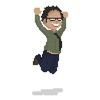
Quintin Marcelino
2,693 PointsNull Pointer Exception for the setText for getDayOfTheWeek when I Click on 7 Day
So, I'm running into a the following error, but only once the app is running and I click on the 7 Day button (which causes the app the crash):
java.lang.NullPointerException: Attempt to invoke virtual method 'void android.widget.TextView.setText(java.lang.CharSequence)' on a null object reference at teamtreehouse.com.stormy.adapters.DayAdapter.getView(DayAdapter.java:63)
The line in DayAdapter it's referencing is: holder.dayLabel.setText(day.getDayOfTheWeek());
I've been bashing at this for a couple hours, reading the boards to see what people with similar issues are doing, but nothing is working. Any help would be greatly appreciated, I'm totally stuck. Here's my code:
package teamtreehouse.com.stormy.adapters;
import android.content.Context; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.BaseAdapter; import android.widget.ImageView; import android.widget.TextView;
import teamtreehouse.com.stormy.R; import teamtreehouse.com.stormy.weather.Day;
/**
-
Created by Quintin on 6/24/2016. */ public class DayAdapter extends BaseAdapter {
private Context mContext; private Day[] mDays;
public DayAdapter(Context context, Day[] days) { mContext = context; mDays = days; }
@Override public int getCount() { return mDays.length; }
@Override public Object getItem(int position) { return mDays[position]; }
@Override public long getItemId(int position) { return 0; //We aren't using this, but it can be used to tag items for easy reference }
@Override public View getView(int position, View convertView, ViewGroup parent) { ViewHolder holder;
if (convertView == null) { //brand new convertView = LayoutInflater.from(mContext).inflate(R.layout.daily_list_item, null); holder = new ViewHolder(); holder.iconImageView = (ImageView) convertView.findViewById(R.id.iconImageView); holder.temperatureLabel = (TextView) convertView.findViewById(R.id.temperatureLabel); convertView.setTag(holder); } else { holder = (ViewHolder) convertView.getTag(); } Day day = mDays[position]; holder.iconImageView.setImageResource(day.getIconId()); holder.temperatureLabel.setText(day.getTemperatureMax() + ""); holder.dayLabel.setText(day.getDayOfTheWeek()); return convertView;
} private static class ViewHolder { ImageView iconImageView; //public by default TextView temperatureLabel; TextView dayLabel; } }
3 Answers

Alex Londono
2,033 PointsI finally traced back this horrible little error. I assume you made a similar mistake Quintin Marcelino, so I reccomend you go into your DayAdapter.java file and look for the line
holder.temperatureLabel = (TextView) convertView.findViewById(R.id.tempLabel);
If your error is exactly like mine, than make sure your (R.id.temperatureLabel) does not accidently have (R.id.tempLabel) in it's place:
holder.temperatureLabel = (TextView) convertView.findViewById(R.id.temperatureLabel);
Below is just a zoomed out view of what the whole method should look like when it's working:
@Override
public View getView(int position, View convertView, ViewGroup parent)
{
ViewHolder holder;
if(convertView == null)
{
convertView = LayoutInflater.from(mContext).inflate(R.layout.daily_list_item, null);
holder = new ViewHolder();
holder.iconImageView = (ImageView) convertView.findViewById(R.id.iconImageView);
holder.temperatureLabel = (TextView) convertView.findViewById(R.id.temperatureLabel);
holder.dayLabel = (TextView) convertView.findViewById(R.id.dayNameLabel);
convertView.setTag(holder);
}
else
{
holder = (ViewHolder) convertView.getTag();
}
Day day = mDays[position];
holder.iconImageView.setImageResource(day.getIconId());
holder.temperatureLabel.setText(day.getTemperatureMax() + "");
holder.dayLabel.setText(day.getDayOfTheWeek());
return convertView;
}
Hope this helps even one person.
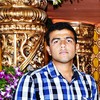
Sagar Suri
6,043 PointsCan you paste your activity code ? I need to go through the complete process of checking.
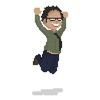
Quintin Marcelino
2,693 PointsSorry for the delay, I really appreciate the help! Here's a link to everything up on GitHub.
https://gist.github.com/quintinmarcelino/15e449121589f85a34954aec2ebee2bf
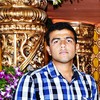
Sagar Suri
6,043 PointsWrite like this:
private Day[] mDays;
public DayAdapter(Context context, Day[] days) { mContext = context; mDays = new Day[days.length]; mDays = days; }
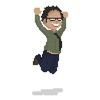
Quintin Marcelino
2,693 PointsHmmm. Tried that extra piece of code and I'm still getting the same result (app runs, but then crashes when clicking on the 7 DAY button, which throws the error on that same line of code from my original question).