Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial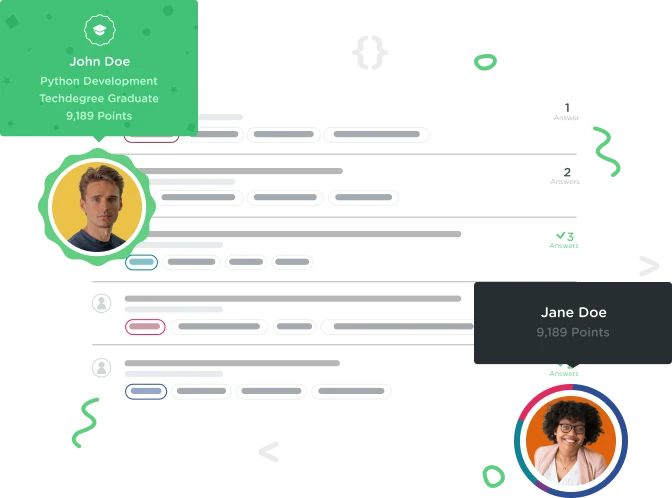

Unsubscribed User
5,777 PointsNullPointerException error...
I'm trying to understand the logic behind why I'm getting this error. This is how I understand this program to work:
Example.java
public class Example {
public static void main(String[] args) { //takes an array of strings args at the command
System.out.println("Starting forum example...");
if (args.length < 2) { //this makes you input your name at the command
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
Forum forum = new Forum("Java"); // we start a new object forum with topic "Java"
User author = new User(args[0], args[1]); //this takes the strings at positions 0 and 1
// that were entered as args at the command and puts them in a User object author
ForumPost post = new ForumPost(author, "Please be done", "This is really awesome if it works" );
//this throws all these inputs into a post object of type ForumPost
forum.addPost(post); //this takes the post object and tosses it into the addPost method
// which uses the inputs from post to print out "New post from %s %s about %s.\n"
}
}
I'm getting a NullPointerException here in the Forum.java file:
public void addPost(ForumPost post) {
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(), // this line here
post.getAuthor().getLastName(),
post.getTitle());
}
Is the method getAuthor(). accessing a null object? If so, how do I make it not null...
Jeff Wilton
16,646 PointsJeff Wilton
16,646 PointsI can't say for certain without seeing all of your code and the actual code you are using to start your program, but I can explain a few things to you:
1) You are getting a nullPointerException because you are trying to call a getter on a null object. On the line you indicated, you are calling two getters, so there are two possibilities for null objects: either the Author (aka User) is null, or the ForumPost itself is null. In the typical programming world it is bad practice to call a getter on an object that has the possibility of being null without first checking to see if it is null:
2) The code you posted looks OK, so the problem might be in your User or Forum classes.
Mine look like this:
Please note, using the 'this' keyword in the constructors is not necessary in this case because the variable names are different (firstName vs mFirstName), but it is a good programming practice. Same thing where I am using the final keyword before my arguments.. just a good programming practice to make sure you don't try to change the argument value to something else inside the method.
3) your code is Example.java looks good to me. I am able to run it with my other classes and get the expected result. :)