Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial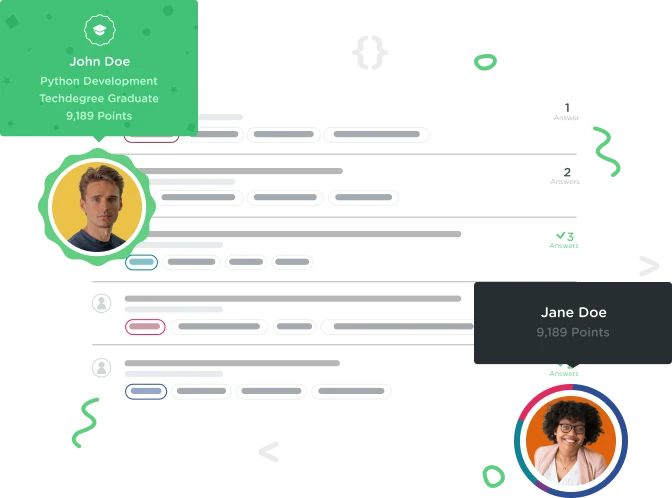
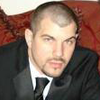
Gary Calhoun
10,317 PointsOk I think I got it now, but how to do it with the indexOf method?
I got this working properly with the != but how would I write it as if it is equal to -1 utilizing the indexOf method
var question = [
['What was Bernini\'s profession?', 'sculptor'],
['What was Michaelangelo\'s profession?', 'sculptor'],
['What was Van Gogh\'s profession?', 'painter']
]
var correct= 0;
function print(message) {
document.write(message);
}
for (var i = 0; i < question.length; i += 1) {
var askQuestion = prompt(question[i][0]);
if(askQuestion.toLowerCase() === question [i][1]){
correct++;
} else if(askQuestion.toLowerCase() != question [i][1]){
prompt("That answer was incorrect.");
}
}
print('You got ' + correct + ' out of 3 questions correct.');
2 Answers

Owen Campbell
6,702 PointsHi,
I think you're after:
var question = [
['What was Bernini\'s profession?', 'sculptor'],
['What was Michaelangelo\'s profession?', 'sculptor'],
['What was Van Gogh\'s profession?', 'painter']
]
var correct= 0;
function print(message) {
document.write(message);
}
for (var i = 0; i < question.length; i += 1) {
var userInput = prompt(question[i][0]);
if(question[i][1].indexOf(userInput) > -1){
correct++;
} else {
prompt("That answer was incorrect.");
}
}
print('You got ' + correct + ' out of 3 questions correct.');
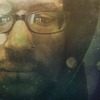
Gary Alan Jackson
14,014 PointsStrangely, I didn't even think to use IndexOf... Although, this was fun to write out.
var answer;
var message = "<h1>Quiz Results</h1><ul>";
var answerArray;
var qAndA = [
["Where was I born?", "RENO"],
["What was the make of my first car?", "FORD"],
["Where do I live now?", "PITTSBURGH"]
];
var getAnswer = function(){
var answerArray = [];
var score = 0;
for(var i = 0; i < qAndA.length; i++){
answer = prompt(qAndA[i][0])
answer = answer.toUpperCase();
answerArray.push(answer);
if(answerArray[i] === qAndA[i][1]){
message += "<li>" + qAndA[i][0] + " <b>" + answerArray[i] + "</b> is <b>CORRECT</b>!</li>";
score++
} else {
message += "<li>" + qAndA[i][0] + " <b>" + answerArray[i] + "</b> is <b>INCORRECT</b>! The correct answer is <b>" + qAndA[i][1] + "</b></li>";
}
console.log(message);
};
message += "</ul> <h1>Thanks for playing!!!</h1><h2>You final score was <b>" + score + "</b>!</h2>";
document.write(message);
}
getAnswer();
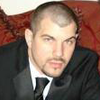
Gary Calhoun
10,317 PointsYeah it was fun i think its good to always explore other options and see which gives your code the most flexibility in case things ever change down the road.
Gary Calhoun
10,317 PointsGary Calhoun
10,317 PointsThat is exactly what i was looking for I was doing it partial it seems I didn't add anything in the indexOf parentheses thats why it wasn't working.. thank you!
Enemuo Felix
1,895 PointsEnemuo Felix
1,895 PointsI like this method used by Owen but my worry is, Will the
.toLowerCase()
be necessary the just in case the user decides to answer in other cases or does theindexOf() > -1
override or cover it(not sure of the correct word to use here)? Jennifer Nordell Katie WoodKatie Wood
19,141 PointsKatie Wood
19,141 PointsResponding to Emenuo's question here:
You would still want to use .toLowerCase() if you wanted to accept answers in any case. That can absolutely be used along with this method, though - you could just change the comparison to:
if(question[i][1].indexOf(userInput.toLowerCase()) > -1){
The above would have it compare to the lowercase version of the user's input. You could also convert the user's input as it is accepted, depending on the program's needs.