Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial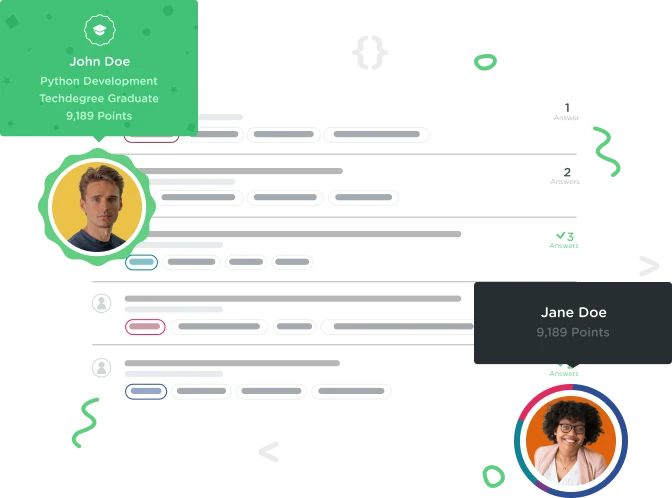

Mayamiko Nanthambwe
1,365 PointsOkay, so let's use our new isFullyCharged helper method to change our implementation details of the charge method.
i'm getting the error message "reached end of file while parsing". how can i fix it?
public class GoKart {
public static final int MAX_ENERGY_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void charge() {
mBarsCount = MAX_ENERGY_BARS;
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
9 Answers

Andrew Brotherton
7,515 PointsI figured it out, the while loop should have been used in the charge method that we created earlier which would be actually charging the kart, then I should keep the return statement. I was looking it seems at the wrong method the entire time.
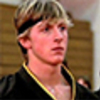
Dan Donche
6,214 PointsI think you are missing a curly brace at the end.

Jonathan Mitchell
1,743 Pointspublic class GoKart {
public static final int MAX_ENERGY_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void charge() {
while (!isFullyCharged()){
mBarsCount++;
}
mBarsCount = MAX_ENERGY_BARS;
/*You must assign the value of bars count to MAX_ENERGY_BARS
in order to prove that it is fully charged
and exit the loop. This will check within the isFullyCharged() method
and prove it to be true,
thus the != true portion is false and you can
exit your while loop. */
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_ENERGY_BARS;
}
}
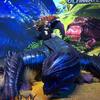
Nicholes Gomez
4,135 Pointspublic boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
while (!isFullyCharged()) {
mBarsCount++;
_ }_
}
}
You needed one more curly bracket where the underscores are.
Sorry couldn't figure out how to put it in the code block style.

Craig Dennis
Treehouse TeacherIf you do 3 backticks followed by the word java and then close with 3 backticks it will format properly.

Andrew Brotherton
7,515 PointsI'm getting still missing a return statement on mine?

Andrew Brotherton
7,515 Points./GoKart.java:27: error: missing return statement } ^ 1 error

Andrew Brotherton
7,515 Pointspublic class GoKart {
public static final int MAX_ENERGY_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void charge() {
mBarsCount = MAX_ENERGY_BARS;
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
while(!isFullyCharged()) {
mBarsCount++;
}
return mBarsCount == MAX_ENERGY_BARS;
}
}

Andrew Brotherton
7,515 PointsThis is what I have listed, I'm incrementing mBarsCount in the while loop, I'm returning mBarsCount equal to MAX_ENERGY_BARS, I'm implementing a while loop and I have all of my closing braces- what am I missing?

Craig Dennis
Treehouse TeacherisFullyCharged
is used to check if the battery is fully charged, it shouldn't actually charge the battery. That is the job of the method named charge
. You should loop in the charge
method checking to see if the battery is not isFullyCharged
.
That make more sense?

Andrew Brotherton
7,515 Pointspublic boolean isFullyCharged() {
while(mBarsCount != MAX_ENERGY_BARS()) {
mBarsCount++;
}
return mBarsCount == MAX_ENERGY_BARS;
}
}

Andrew Brotherton
7,515 PointsI think this possibly fits?

Sean McKeown
23,267 PointsI think I just coded isFullyCharged like this:
public boolean isFullyCharged() {
return mBarsCount == MAX_ENERGY_BARS;
}
Hope that helps