Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial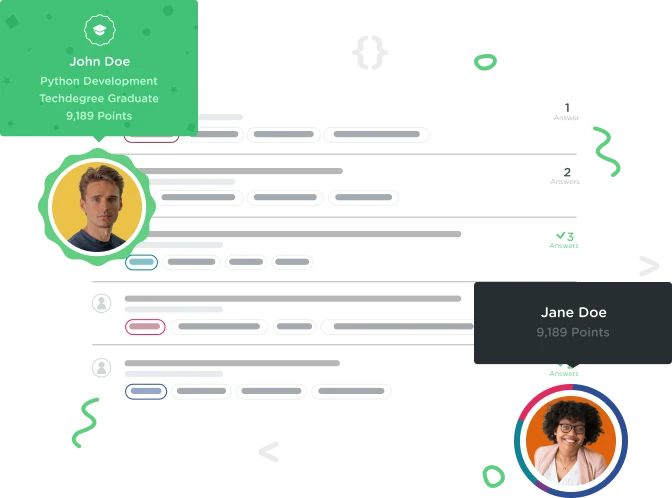

Aaron Captain
2,028 PointsOn the C# Basic final challenge task part 2, my code does all the challenge ask me to do but it is saying it isn't.
The challenge says to let the user input how many times to say "Yay!", then display it. If the user inputs anything that isn't a whole number, tell them "You must enter a whole number." and prompt them to try again. I have done all this in my code but it is telling me Task 1 is no longer working when it is!
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
var truth = true;
while (truth)
{
try
{
Console.Write("Enter the number of times to print \"Yay!\": ");
string entry = Console.ReadLine();
int DisplayNumber = int.Parse(entry);
if (DisplayNumber =< 0)
{
Console.WriteLine("You must enter a whole number.");
continue;
}
else
{
for (int x = 0; x < DisplayNumber; x++)
{
Console.WriteLine("\"Yay!\"");
truth = false;
}
}
Console.ReadKey();
}
catch (FormatException)
{
Console.WriteLine("You must enter a whole number.");
continue;
}
}
Console.WriteLine("PEACE!");
Console.ReadKey();
}
}
}
3 Answers

nolobster
22,543 PointsTake another look at this code bit:
if (DisplayNumber =< 0)
{
Console.WriteLine("You must enter a whole number.");
continue;
}
Is it really looking for a whole number?

Aaron Captain
2,028 PointsEven when I changed it to include zero it still told me it was wrong.

nolobster
22,543 PointsLet me put it this way, 1.2 is greater than 0, but is it a whole number?
Furthermore look at the int.tryparse method.
I would try something like this
if (int.TryParse(entry)
{
for (int x = 0; x < entry; x++)
{
Console.WriteLine("\"Yay!\"");
truth = false;
}
} else {
Console.WriteLine("You must enter a whole number.");
continue;
}

Aaron Captain
2,028 PointsI will try that. I still do not under though, wouldn't any decimal be seen by my catch and tell the user enter a whole number anyways?

nolobster
22,543 PointsA TryParse does try/catch internally, which means it won't throw an exception, it will return false instead
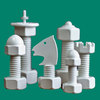
Steven Parker
231,108 Points
Your program deviates from the instructions.
This has been a topic of many previous questions, but I'll repost the info here:
People often over-think this one and get too creative, and their answer is rejected for a variety of reasons (some which don't seem to make any sense).
Here are the secret "rules" you must follow for success on this one:
- Your program must ask for and accept input ONE TIME ONLY
- If the input validates, your program will perform exactly as in Task 1
- If the input does not validate, it will print the error message and exit
- Validation must be done by exception catching. There are other perfectly good methods that can be used for validation, but they will not pass this challenge
It looks like you have an extra loop that is violating the first and third "rule", an extra output displaying "PEACE!", a "ReadKey" that also voilates rule 1 and prevents the program from exiting as expected, and "=<" is not a valid comparison operator (you probably intended "<=").
Aaron Captain
2,028 PointsAaron Captain
2,028 PointsSystem.ArgumentNullException: Value cannot be null. Parameter name: String at System.Number.StringToNumber (System.String str, NumberStyles options, System.NumberBuffer& number, System.Globalization.NumberFormatInfo info, Boolean parseDecimal) [0x00054] in /builddir/build/BUILD/mono-4.4.2/external/referencesource/mscorlib/system/number.cs:1074 at System.Number.ParseInt32 (System.String s, NumberStyles style, System.Globalization.NumberFormatInfo info) [0x00014] in /builddir/build/BUILD/mono-4.4.2/external/referencesource/mscorlib/system/number.cs:745 at System.Int32.Parse (System.String s) [0x00000] in /builddir/build/BUILD/mono-4.4.2/external/referencesource/mscorlib/system/int32.cs:120 at Treehouse.CodeChallenges.Program.Main () <0x41f54f10 + 0x00067> in :0 at MonoTester.Run () [0x00095] in MonoTester.cs:86 at MonoTester.Main (System.String[] args) [0x00013] in MonoTester.cs:28
this is what i am getting when i preview the code but in Visual Studios I am getting no error at all