Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial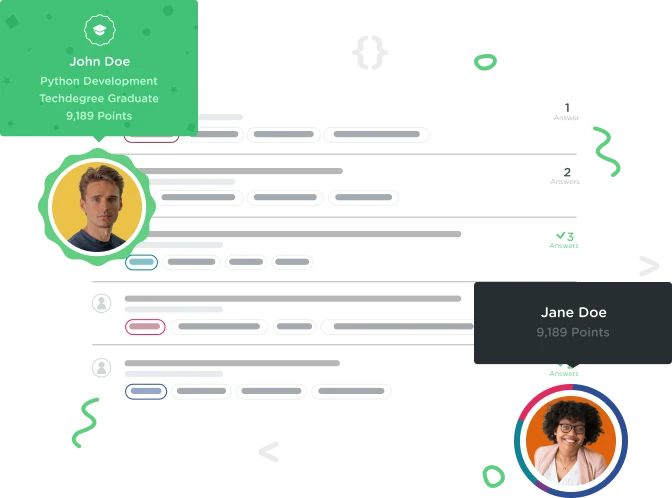

Genaro Garcia Jr
5,481 PointsPagination no longer working
I copied the code exactly how Alena wrote it, but it doesn't seem to work. I re-watched the videos multiple times and still could't find the issue. It's been driving me nuts!
catalog.php
<?php
include("inc/functions.php");
$pageTitle = "Full Catalog";
$section = null;
$search = null;
$items_per_page = 8;
if (isset($_GET["cat"])) {
if ($_GET["cat"] == "books") {
$pageTitle = "Books";
$section = "books";
} else if ($_GET["cat"] == "movies") {
$pageTitle = "Movies";
$section = "movies";
} else if ($_GET["cat"] == "music") {
$pageTitle = "Music";
$section = "music";
}
}
if(isset($_GET["s"])) {
$search = filter_input(INPUT_GET, "s", FILTER_SANITIZE_STRING);
}
if(isset($_GET["pg"])) {
$current_page = filter_input(INPUT_GET, "pg", FILTER_SANITIZE_NUMBER_INT);
}
if(empty($current_page)) {
$current_page = 1;
}
$total_items = get_catalog_count($section, $search);
$total_pages = 1;
$offset = 0;
if($total_items > 0) {
$total_page = ceil($total_items / $items_per_page);
// LIMIT RESULTS IN REDIRECT
$limit_results = "";
if(!empty($search)) {
$limit_results = "s=".urlencode(htmlspecialchars($search))."&";
} else if (!empty($section)) {
$limit_results = "cat=" . $section . "&";
}
// REDIRECT TOO-BIG PAGE NUMBERS TO THE LAST PAGE
if ($current_page > $total_pages) {
header("location:catalog.php?". $limit_results. "pg=".$total_pages);
}
// REDIRECT TOO-SMALL PAGE NUMBERS TO THE FIRST PAGE
if ($current_page < 1) {
header("location:catalog.php?". $limit_results. "pg=1");
}
// DETERMINE THE OFFSET FOR THE CURRENT PAGE
$offset = ($current_page - 1) * $items_per_page;
$pagination = "<div class=\"pagination\">";
$pagination .= "Pages: ";
for ($i = 1;$i <= $total_pages;$i++) {
if ($i == $current_page) {
$pagination .= " <span>$i</span>";
} else {
$pagination .= " <a href='catalog.php?";
if(!empty($search)) {
$pagination .= "s=".urlencode(htmlspecialchars($search))."&";
} else if (!empty($section)) {
$pagination .= "cat=".$section."&";
}
$pagination .= "pg=$i'>$i</a>";
}
}
$pagination .= "</div>";
}
if(!empty($search)) {
$catalog = search_catalog_array($search, $items_per_page, $offset);
} else if(empty($section)) {
$catalog = full_catalog_array($items_per_page, $offset);
} else {
$catalog = category_catalog_array($section, $items_per_page, $offset);
}
include("inc/header.php"); ?>
<div class="section catalog page">
<div class="wrapper">
<h1><?php
if ($search != null) {
echo "Search Results for \"".htmlspecialchars($search)."\"";
} else {
if ($section != null) {
echo "<a href='catalog.php'>Full Catalog</a> > ";
}
echo $pageTitle;
}
?></h1>
<?php
if($total_items < 1) {
echo "<p>No items were found matching that search term.</p>";
echo "<p>Search Again or "
. "<a href=\"catalog.php\">Browse the Full Catalog</a></p>";
} else {
echo $pagination; ?>
<ul class="items">
<?php
foreach ($catalog as $item) {
echo get_item_html($item);
}
?>
</ul>
<?php echo $pagination;
}?>
</div>
</div>
<?php include("inc/footer.php"); ?>
functions.php
<?php
// CATALOG.PHP
// PAGINATION
function get_catalog_count($category = null, $search = null) {
$category = strtolower($category);
include("connection.php");
try {
// QUERY COUNTS ALL THE ITEMS IN THE DATABSE
$sql = "SELECT COUNT(media_id) FROM Media";
if(!empty($search)) {
$result = $db->prepare($sql . " WHERE title LIKE ? ");
$result->bindValue(1, '%'.$search.'%', PDO::PARAM_STR);
} else if(!empty($category)) {
$result = $db->prepare($sql . " WHERE LOWER(category) = ? ");
$result->bindParam(1, $category, PDO::PARAM_STR);
} else {
$result = $db->prepare($sql);
}
$result->execute();
} catch(PDOException $e) {
echo "bad query";
echo $e->getMesage();
}
$count = $result->fetchColumn(0);
return $count;
}
function full_catalog_array($limit = null, $offset = 0) {
include("connection.php");
// RETRIEVE RESULTS FROM THE DATABSE USING A QUERY
try {
// STORE THE QUERY IN A VARIABLE
$sql = "SELECT media_id ,title, category, img
FROM Media
ORDER BY
REPLACE(
REPLACE(
REPLACE(title,'The ',''),
'An ',
''
),
'A ',
''
)";
if(is_integer($limit)) {
$results = $db->prepare($sql . " LIMIT ? OFFSET ?");
$results->bindParam(1, $limit, PDO::PARAM_INT);
$results->bindParam(2, $offset, PDO::PARAM_INT);
} else {
$results = $db->prepare($sql);
}
$results->execute();
} catch(PDOException $e) {
echo "unable to retrieve results";
echo $e->getMessage();
exit;
}
$catalog = $results->fetchAll();
return $catalog;
}
// CATALOG.PHP
// FILTERING DATA BY CATEGORY
function category_catalog_array($category, $limit = null, $offset = 0) {
include("connection.php");
$category = strtolower($category);
// RETRIEVE RESULTS FROM THE DATABSE USING A QUERY
try {
// STORE THE QUERY IN A VARIABLE
$sql = "SELECT media_id ,title, category, img
FROM Media
WHERE LOWER(category) = ?
ORDER BY
REPLACE(
REPLACE(
REPLACE(title,'The ',''),
'An ',
''
),
'A ',
''
)";
if(is_integer($limit)) {
$results = $db->prepare($sql . " LIMIT ? OFFSET ?");
$results->bindParam(1, $category, PDO::PARAM_STR);
$results->bindParam(2, $limit, PDO::PARAM_INT);
$results->bindParam(3, $offset, PDO::PARAM_INT);
} else {
$results = $db->prepare($sql);
$results->bindParam(1, $category, PDO::PARAM_STR);
}
$results->execute();
} catch(PDOException $e) {
echo $e->getMessage();
echo "unable to retrieve results";
exit;
}
$catalog = $results->fetchAll();
return $catalog;
}
// SEARCH FUNCTION
function search_catalog_array($search, $limit = null, $offset = 0) {
include("connection.php");
try {
$sql = "SELECT media_id, title, category,img
FROM Media
WHERE title LIKE ?
ORDER BY
REPLACE(
REPLACE(
REPLACE(title,'The ',''),
'An ',
''
),
'A ',
''
)";
if (is_integer($limit)) {
$results = $db->prepare($sql . " LIMIT ? OFFSET ?");
$results->bindValue(1,"%".$search."%",PDO::PARAM_STR);
$results->bindParam(2,$limit,PDO::PARAM_INT);
$results->bindParam(3,$offset,PDO::PARAM_INT);
} else {
$results = $db->prepare($sql);
$results->bindValue(1,"%".$search."%",PDO::PARAM_STR);
}
$results->execute();
} catch (Exception $e) {
echo "Unable to retrieved results";
echo $e->getMessage();
exit;
}
$catalog = $results->fetchAll();
return $catalog;
}
// INDEX.PHP
function random_catalog_array() {
include("connection.php");
// RETRIEVE RESULTS FROM THE DATABSE USING A QUERY
try {
// STORE THE QUERY IN A VARIABLE
// SELECTING FOUR RANDOM ITEMS USER A QUERY
$results = $db->query(
"SELECT media_id ,title, category, img
FROM Media
ORDER BY RAND()
LIMIT 4"
);
} catch(PDOException $e) {
echo "Unable to retrieve results";
echo $e->getMessage();
exit;
}
// $catalog = $results->fetchAll(PDO::FETCH_ASSOC);
$catalog = $results->fetchAll();
return $catalog;
}
// DETAILS.PHP
function single_item_array($id) {
include("connection.php");
// RETRIEVE RESULTS FROM THE DATABSE USING A QUERY
try {
// STORE THE QUERY IN A VARIABLE
$results = $db->prepare(
"SELECT title, category, img, format, year, publisher, isbn, genre
FROM media
JOIN genres ON media.genre_id = genres.genre_id
LEFT OUTER JOIN books
ON media.media_id = books.media_id
WHERE media.media_id = ?"
);
// binds the passed argument before executing the query
$results->bindParam(1, $id, PDO::PARAM_INT);
$results->execute();
} catch(PDOException $e) {
echo "unable to retrieve results";
echo $e->getMessage();
exit;
}
// GOING TO RETURN ON ITEM ONLY
$item = $results->fetch();
if (empty($item)) return $item;
try {
// STORE THE QUERY IN A VARIABLE
$results = $db->prepare(
"SELECT fullname, role
FROM media_people
JOIN people ON media_people.people_id = people.people_id
WHERE media_people.media_id = ?"
);
// binds the passed argument before executing the query
$results->bindParam(1, $id, PDO::PARAM_INT);
$results->execute();
} catch(PDOException $e) {
echo "unable to retrieve results";
echo $e->getMessage();
exit;
}
while ($row = $results->fetch(PDO::FETCH_ASSOC)) {
$item[$row["role"]] [] = $row["fullname"];
}
return $item;
}
function genre_array($category = null) {
$category = strtolower($category);
include("connection.php");
try {
$sql = "SELECT genre, category"
. " FROM Genres "
. " JOIN Genre_Categories "
. " ON Genres.genre_id = Genre_Categories.genre_id ";
if (!empty($category)) {
$results = $db->prepare($sql
. " WHERE LOWER(category) = ?"
. " ORDER BY genre");
$results->bindParam(1,$category,PDO::PARAM_STR);
} else {
$results = $db->prepare($sql . " ORDER BY genre");
}
$results->execute();
} catch (Exception $e) {
echo "bad query";
echo $e->getMessage();
}
$genres = array();
while ($row = $results->fetch(PDO::FETCH_ASSOC)) {
$genres[$row["category"]][] = $row["genre"];
}
return $genres;
}
// HTML TEMPLATE
function get_item_html($item) {
$output = "<li><a href='details.php?id="
. $item["media_id"] . "'><img src='"
. $item["img"] . "' alt='"
. $item["title"] . "' />"
. "<p>View Details</p>"
. "</a></li>";
return $output;
}
function array_category($catalog,$category) {
$output = array();
foreach ($catalog as $id => $item) {
if ($category == null OR strtolower($category) == strtolower($item["category"])) {
$sort = $item["title"];
$sort = ltrim($sort,"The ");
$sort = ltrim($sort,"A ");
$sort = ltrim($sort,"An ");
$output[$id] = $sort;
}
}
asort($output);
return array_keys($output);
}
?>
Brian Kidd
UX Design Techdegree Graduate 36,477 PointsBrian Kidd
UX Design Techdegree Graduate 36,477 PointsHi Genaro, What, if any, error messages are you getting when you try to load the page?