Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial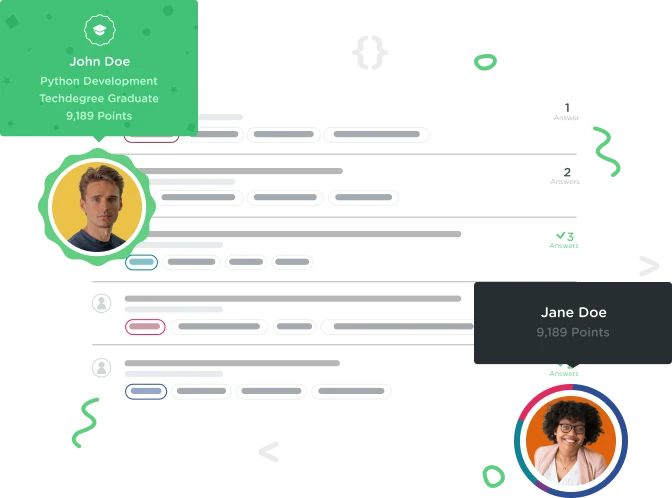
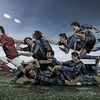
San Francisco
28,373 PointsPart 2 of code challenge: The code in Main.java is going to throw an IllegalArgumentException when the drive method is c
Part 2 of the handling exceptions code challenge: The code in Main.java is going to throw an IllegalArgumentException when the drive method is called. Catch it and warn the user.
Here is my code, can someone please give an actual working code example (no hints or fake help please)
public class Main
{
public static void main(String[] args)
{
GoKart kart = new GoKart("yellow");
if (kart.isBatteryEmpty())
{
System.out.println("The battery is empty");
}
kart.drive(2);
while (kart.isBatteryEmpty())
catch (IllegalArgumentException)
{
System.out.println("Warning Brah");
}
}
}
4 Answers

Stone Preston
42,016 PointsCraig covers using try/catch at 3:37 of this video
you need to wrap the call to the drive method in a try/catch block (you are missing the try, you just have catch)
also remember that you must create a parameter in the catch, most of the time this is just called e for exception.
you then need to use the exception parameter e to get the message
public class Main {
public static void main(String[] args) {
GoKart kart = new GoKart("yellow");
if (kart.isBatteryEmpty()) {
System.out.println("The battery is empty");
}
// wrap potentially dangerous code in the try block
try {
kart.drive(2);
}
// catch an illegal argument exception e and use e to get the message
catch (IllegalArgumentException e) {
System.out.println(e.getMessage());
}
}
}
you can read more about try/catch blocks in the documentation

Rahmat Abdhir
15,198 Pointse is the new object you created from IllegalArgumentException class, and you invoke getMessage method on this new object
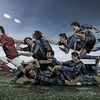
San Francisco
28,373 PointsThank you very much Stone!

Stone Preston
42,016 Pointsno problem
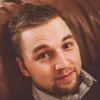
Andy Hammond
5,415 PointsWhere is "e.getMessage()" ?? and what are you referring to when you put the e after IllegalArgumentException?