Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial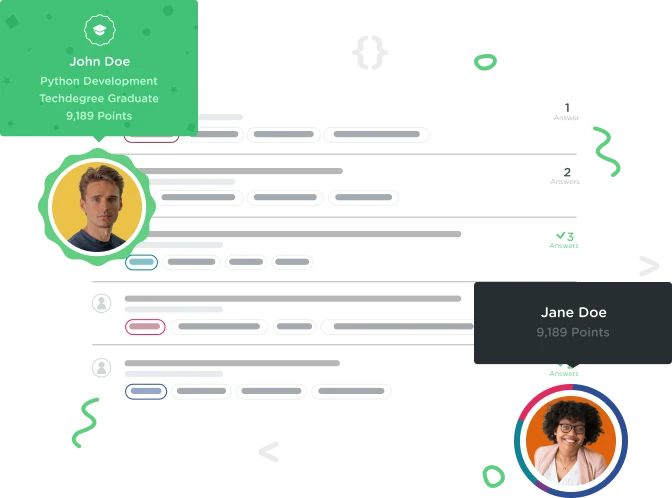
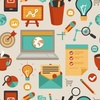
saad
26,165 PointsPassing a PHP variable of mysqli_insert_id() to another PHP page
Hi Guys,
I’m working on building a toy website and have been unable to resolve the issue below (i.e. passing a variable of mysqli_insert_id() to other pages ).
I have two pages 1) toy_form.php and 2) toy_details.php.
toy_form,php
The toy_form,php has a form with two input fields namely “Toy Name” and “Toy Price”. The values are transmitted via a PHP script into a MySQL database.
The MySQL database has three columns 1) toy_id (auto incremented & primary 2) toy_name 3) toy_price
After pressing the “submit” button on the form the user is sent to toy_details.php.
toy_details.php
The toy_details.php is meant to show all three MySQL columns for the row/entry that was just sent via the toy_form.php. However, an error is produced here.
The Problem
The PHP/SQL statement “$query = "SELECT * FROM toys_db WHERE toy_id = $toy_id";” produces the following error:
Undefined variable: toy_id in /Applications/MAMP/htdocs/Sites/Toys/toy_details.php on line 18
Could someone please kindly tell me how I can pass the variable "$toy_id = mysqli_insert_id($con);” to another page (i.e toy_details.php) so that my reference to it won’t be undefined. Otherwise, please kindly suggest another way I may display all the columns for an auto incremented ID which is the most recent entry (for that connection) into the database.
The Code
toy_form.php
<html>
<head>
<title>Toy Form</title>
</head>
<body>
<h1>Toy Form</h1>
<form role="form" method="post" action="">
<div class="form-group">
<label for="toy_name">Toy Name</label>
<input type="text" name="toy_name">
</div>
<div class="form-group">
<label for="toy_price">Toy Price</label>
<input type="text" name="toy_price">
</div>
<button type="submit" name="submit">Submit</button>
</form>
</body>
</html>
<?php
$con=mysqli_connect("localhost","root","root","toys_db");
if (mysqli_connect_errno()) {
echo "Failed to connect to MySQL: " . mysqli_connect_error();
}
if(isset($_POST ['submit'])) {
$toy_name = $_POST['toy_name'];
$toy_price = $_POST['toy_price'];
if($toy_name=="") {
echo "There was an error, please enter a name.";
exit();
}
if($toy_price=="") {
echo "There was an error, please enter a price.";
exit();
}
$query = "INSERT INTO toys_db (toy_name,toy_price) VALUES ('$toy_name','$toy_price')";
$run = mysqli_query($con,$query);
$toy_id = mysqli_insert_id($con);
/*if ($toy_id == true) {
echo $toy_id;
}*/
if ($toy_id == true) {
echo "<script>window.open('toy_details.php', '_self')</script>";
}
}
mysqli_close($con);
?>
toy_details.php
<html>
<head>
<title>Toy Details</title>
</head>
<body>
<h1>Toy Details</h1>
<?php
$con=mysqli_connect("localhost","root","root","toys_db");
if (mysqli_connect_errno()) {
echo "Failed to connect to MySQL: " . mysqli_connect_error();
}
$query = "SELECT * FROM toys_db WHERE toy_id = $toy_id";
$run = mysqli_query($con,$query);
while ($row=mysqli_fetch_array($run)) {
$toy_id = $row[0];
$toy_name = $row[1];
$toy_price = $row[2];
?>
<?php echo $toy_id; ?></br>
<?php echo $toy_name; ?></br>
<?php echo $toy_price; ?></br>
<?php } ?>
<?php mysqli_close($con); ?>
</body>
</html>
Thank You :-)
2 Answers
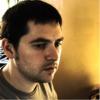
geoffrey
28,736 PointsI think what you need is sessions. Check this link for more information. With this, you'll be able to transmit the value accross all the page if needed.
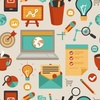
saad
26,165 PointsHi Geoffrey,
Thank you very much for your guidance :-), I'm new to PHP thus I had no idea which direction to head in.
For the benefit of beginners like me, I added the following three pieces of code:
//added to the beginning of toy_form.php and toy_details.php
<?php
session_start();
?>
//added after "$toy_id = mysqli_insert_id($con);". Here the row ID for the toy is assigned to a session variable
$_SESSION['toys_id'] = $toy_id;
//amended SQL query to extract the data from the session variable
$query = "SELECT * FROM toys_db WHERE toy_id = '"
. ($_SESSION['toys_id']) . "'";