Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial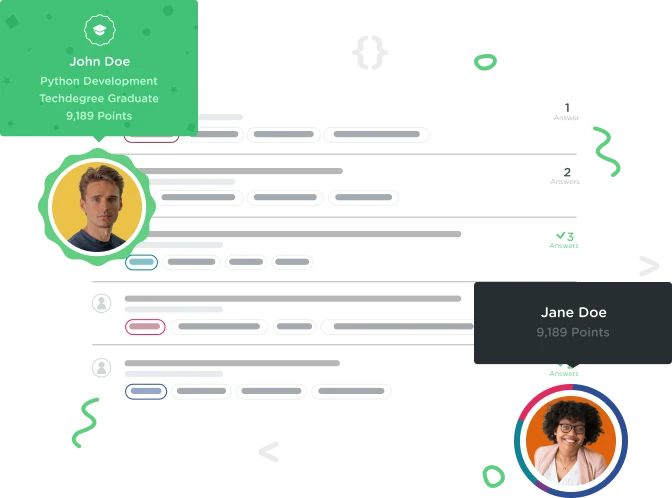

Jeff Styles
2,784 Pointsphp form processing - avoiding a new page for errors...
I'd really like to issue javascript pop ups for error messages instead of echoing the message on another page. The alerts are working but after closing the pop up, contact.php then reloads and I'm left with a blank white page. Any suggestions? My code looks like this:
if ($name == "" OR $email == "" OR $message == "") { ?>
<script type="text/javascript">
alert ("Name, email, and message are required fields.")
</script><?php
exit;
I have tried using header("Location: contact.php")
to reload to the blank form, but with no luck, just loads to blank page again.
Thanks for any advice you can provide!
3 Answers

Corey Cramer
9,453 PointsYou could fix this with Javascript on the page with your form before it submits to the server. On the back end you're still going to want to validate everything but it'll keep you on the same page for people skipping form fields so long as they have Java enabled.
Javascript to process your form:
function ValidateForm(form)
{
if (form.name.value == "") {
alert("Name is a required field");
form.name.focus();
return;
}
if (form.email.value == "") {
alert("E-Mail is a required field");
form.email.focus();
return;
}
if (form.message.value == "") {
alert("Message is a required field");
form.message.focus();
return;
}
form.submit();
}
HTML Form:
<form method="post" action="form_processor.php">
<!-- Form Fields -->
<input type="button" value="Contact Me" onClick="ValidateForm(this.form)">
</form>
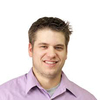
Kevin Korte
28,149 PointsWhat happens if the user has javascript disabled?

Corey Cramer
9,453 PointsIdeally there should still be the same logic in the form processor to check for empty fields before trying to craft an e-mail (this seems to be what is going on). If they have Javascript disabled then the whole question kind of falls away.
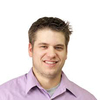
Kevin Korte
28,149 PointsCorrect, except for if you're only returning any errors in Javascript, than any user with Javascript disabled will have no messages returned to them as to why it failed, which is not the best UX.
Now granted, the amount of users running around with JS disabled it minuscule, but really my point is if you're going to check for errors server side (which you should 100% as you mentioned), you should return error messages via the server too. Leave JS error messages to JS validation checks, so you still have graceful fallbacks for users without JS.
That was my point. I wanted Jeff to think about what a user without JS would get back in the event the form had errors.

Jeff Styles
2,784 PointsThanks Kevin, for giving me so much to consider. I was not planning to verify with javascript in leiu of PHP. I really was just trying to use it to output the error messages while remaining on the form page. I spent a bit of time yesterday (while at work lol) to come up with the following:
if ($name == ""){ ?>
<script type="text/javascript">
alert ("Name is a required field.");
window.location.replace("contact.php");</script>
<noscript><?php echo "Name is a required field.";?></noscript>
<?php exit;
}
if ($email == ""){ ?>
<script type="text/javascript">
alert ("Email is a required field.");
window.location.replace("contact.php");</script>
<noscript><?php echo "Email is a required field.";?></noscript>
<?php exit;
}
if ($message == ""){ ?>
<script type="text/javascript">
alert ("Message is a required field.");
window.location.replace("contact.php");</script>
<noscript><?php echo "Message is a required field.";?></noscript>
<?php exit;
}
In my testing this SEEMS TO work to issue errors via javascript alert, while still allowing those who have it disabled to get the php echoed message instead.
HOWEVER, today after reading your advice I definitely agree that it would be best to try and validate before submission, or at the very least, retain the info already input into the fields so the user needn't retype much for a simple error.
I've certainly got much to learn. Thanks again!

Jeff Styles
2,784 PointsHI kevin, thanks for taking the time to help me out. As a greenhorn I really appreciate all the advice I can get. I was hoping to issue a disclaimer on the form page noting that site the would function optimally with js enabled. And as far as graceful degradation, maybe use noscript to redirect to a duplicate form page that will simply echo the errors for those who don't. However, I'm sure I'm not seeing this from all of the angles that you may be. Thanks again!
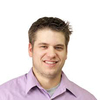
Kevin Korte
28,149 PointsHi Jeff, no problem man, I know how much there is to it. I think, your idea would work, but how I tend to view error handling with forms is this:
If I do any JS validation, it is simply from a UI point. I'll try to tell a user their email might not be a valid email before they hit "submit" to save them a few seconds. Little validation and hits like that. A malicious user can simply turn off their JS, or change your JS validation rules to bypass them, so again, this is just for a better user experience.
The real security validation has to happen on the server side that, after the user hits submit. I tend to do my validation checks, and if a check fails, alert on the input that failed, why it failed and repopulate the form with the rest of the data so the user doesn't have to fill the form out again. Nothing is more frustrating than that, right?
The only other problem I see with your idea is from a UI, is that your error messages are disconnected from where the error happened. By erroring on the form input, it's much easier for a user to zero in on the error, faster.
It's okay if you don't get to this point today. Forms are very tricky and difficult todo. What I outlined is a lot of work. Try it your way, and at least get something somewhat working. You'll learn a lot doing so.
I just wanted you to see where you'll likely end up. It's always good to know your destination before you start the road trip. Happy coding and stick with it! There are some good videos here about forms, and I'd also suggest watching Codecourse on youtube for some great php lessons.
Jeff Styles
2,784 PointsJeff Styles
2,784 PointsThanks so much for the help, Corey! With a little tinkering i think i have found a solution that seems to meet my needs - redirecting with javascript after the alert :