Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial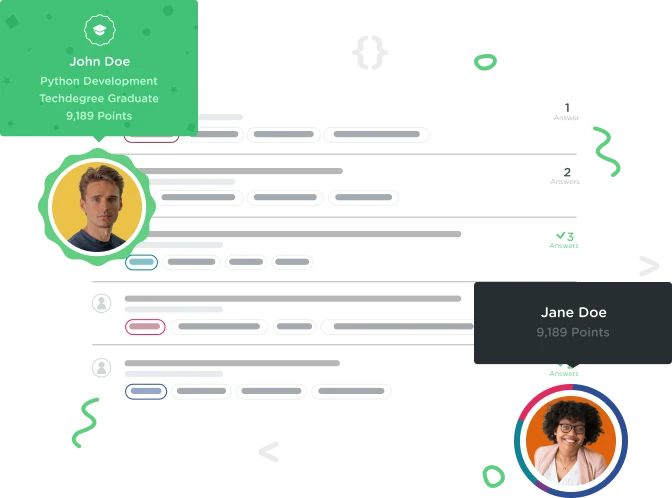
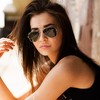
Domnick Knowlton
Courses Plus Student 2,437 PointsPHP Function Code Challenge
I am on this code challenge
Here is my code
<?php
$numbers = array(1,5,8);
$array_sum = 0; foreach($numbers as $number) { $sum = array_sum($numbers); + $number; }
echo $sum;
?>
5 Answers

Ken Alger
Treehouse TeacherDomnick;
Okay, let's break this down again.
Challenge Task 1
The code below creates an array of numbers, loops through them one at a time, and sums their values. PHP actually has a native function that does this same thing:
array_sum(
). [Thearray_sum()
function receives an array as its one and only argument, and it sends back the sum as the return value.] Modify the code below: remove the foreach loop and the working sum variable, replacing them with a call to thearray_sum()
function instead.
Here is the code with which we are prompted:
<?php
$numbers = array(1,5,8);
$sum = 0;
foreach($numbers as $number) {
$sum = $sum + $number;
}
echo $sum;
?>
As the challenge instructions say, the foreach statement in the code loops through the given array and adds the numbers together into the variable $sum
. With the given array of numbers for the challenge then, we should expect $sum
to be equal to 1+5+8, or 14. Hopefully that much hasn't lost you. If so, I would encourage to watch the video(s) again.
As is stated in the instructions there is a native PHP function that does the the exact same thing as the foreach loop. That function is the array_sum()
function. Our task as described then is to:
Modify the code below: remove the foreach loop and the working sum variable, replacing them with a call to the
array_sum()
function instead.
We would do that as follows:
<?php
$numbers = array(1,5,8);
$sum = 0;
$sum = array_sum($numbers);
echo $sum;
?>
Our new function then does the same thing as the foreach loop, just in a simpler to code and read fashion. Plus... less typing!
I apologize again for any confusion and frustration my previous posts caused. I was simply attempting to demonstrate the concept without providing the answer straight away.
Please post back if you are still stuck, frustrated, or just pleased to be done with this challenge.
Ken

Ken Alger
Treehouse TeacherDomnick;
You need to completely remove the foreach loop in this challenge and replace it with the array_sum()
function. If you look at the PHP docs for the function you will see that it takes an array of numbers as it's argument and then return's the sum. Basically doing the same thing as the provided foreach loop in a simpler way.
For example:
<?php
$number_array = array(1,2,3,4,5,6);
$sum_of_array = 0;
$sum_of_array = array_sum($number_array);
echo $sum_of_array;
?>
If we were to run the above code we should expect to have it output the sum of 1,2,3,4,5,6 or 21.
Post back if you are still stuck.
Happy coding,
Ken
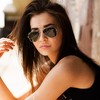
Domnick Knowlton
Courses Plus Student 2,437 PointsI give up.

Ken Alger
Treehouse TeacherDomnick;
Don't give up yet!
Let's take another crack at this. The array_sum() function does the same thing as the foreach loop. So replace the loop with the function.
$sum = array_sum($numbers);
Should do the trick instead of the foreach statement.
Post back if you are still stuck.
Happy coding,
Ken
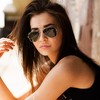
Domnick Knowlton
Courses Plus Student 2,437 Points** Sigh **
This is not working <?php
$numbers = array(1,5,8); $sum_of_array = 0; $sum_of_array = array_sum($number_array);
echo $sum_of_array;
?>

Ken Alger
Treehouse TeacherDomnick;
Sorry for the confusion on this. Rereading my posts I see how I could have led to some confusion.
Okay, take the code from my first post and think if it as a very close code sample to get through the challenge without giving you the exact answer. Now, ignore it.
My second answer code, use that in the code challenge in place of the foreach statement.
Again, sorry for the confusion and please post back if you are still stuck and I'll see if I can explain things better.
Ken
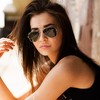
Domnick Knowlton
Courses Plus Student 2,437 PointsI am so lost now, I'm seriously about to give up.

Ken Alger
Treehouse TeacherDon't give up! Give me a little bit to get back to my main computer and I'll post a new, and hopefully better, answer.
Is that a deal?
Ken
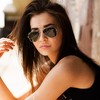
Domnick Knowlton
Courses Plus Student 2,437 PointsYes, but try to hurry if you can, I don't want to continue unless I complete the challenge.
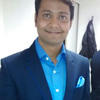
Sarfaraj Alam
14,074 Points<?php
$numbers = array(1,5,8);
$sum = 0; $sum = array_sum($numbers); $sum = $sum + $number;
echo $sum;
?>
here is the correct code.
Domnick Knowlton
Courses Plus Student 2,437 PointsDomnick Knowlton
Courses Plus Student 2,437 PointsThank you so much! I almost got the code but, it was in the wrong places. Thanks so much! :)
Ken Alger
Treehouse TeacherKen Alger
Treehouse TeacherYou bet. Sorry that it took so long and was so frustrating. I hate to say it, but I'm sure it won't be the last frustrating coding experience in your future.
Way to stick it out though!
Nice work!
Ken
Domnick Knowlton
Courses Plus Student 2,437 PointsDomnick Knowlton
Courses Plus Student 2,437 PointsYep, I am already stuck on the PHP Mailer part with sending the E-Mail. But, I am trying to figure it out by myself first.
Ken Alger
Treehouse TeacherKen Alger
Treehouse TeacherDomnick;
If you get really stuck and have watched the video again and still can't get through it start a new forum post and I'm sure someone will assist.
Ken