Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial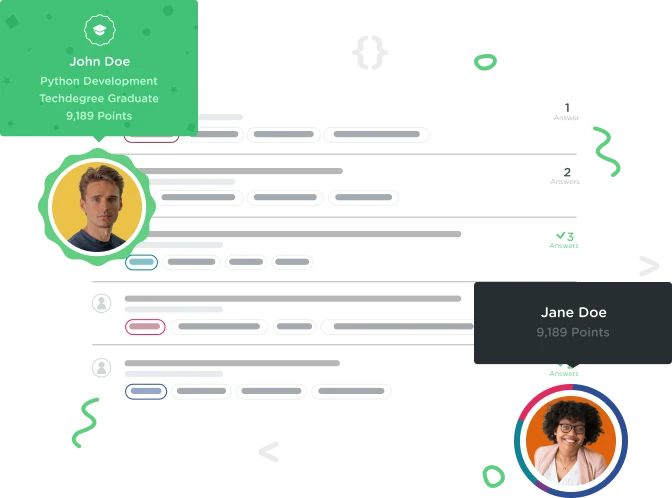
Trevor Wood
17,828 PointsPHP, just trying to find out whats wrong here.
Not quite sure why this doesn't work. Can anybody tell me why? Was trying to keep it "DRY" and reuse old code but, if this code doesn't work here, is it possible?
Basically, trying to use the getInfo in Fish and then add to it in Trout without having to rewrite the whole code a second time(which would be reptitive).
<?php
class Fish
{
public $common_name;
public $flavor;
public $record_weight;
function __construct($name, $flavor, $record){
$this->common_name = $name;
$this->flavor = $flavor;
$this->record_weight = $record;
}
public function getInfo() {
$output = "The {$this->common_name} is an awesome fish. ";
$output .= "It is very {$this->flavor} when eaten. ";
$output .= "Currently the world record {$this->common_name} weighed {$this->record_weight}.";
return $output;
}
}
class Trout extends Fish
{
public $species;
function __construct($name, $flavor, $record, $species){
parent::__construct($name, $flavor, $record);
$this->species = $species;
}
function getInfo(){
parent::getInfo($name, $flavor, $record);
$output .= "This trout is a {$this->species} trout.";
return $output;
}
}
$brook_trout = new Trout("Trout","Delicious","14 pounds 8 ounces","Brook");
?>
2 Answers

Seth Kroger
56,416 Points$output is a local variable in both getInfo methods, meaning it it can only be accessed in its respective method. This is why you return the $output at the end, so the value will be usable elsewhere. It you want to append the Trout's info to the fish's then you'd need to do it like this:
<?php
function getInfo(){
$output = parent::getInfo($name, $flavor, $record);
$output .= "This trout is a {$this->species} trout.";
return $output;
}
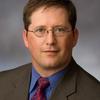
Ted Sumner
Courses Plus Student 17,967 PointsI don't think you can do parent:: for a method. You should be able to call the method in the class. My one concern is that it has the same name. You will not pass the challenge without writing the entire getInfo() in the Trout class. It would be interesting to try this in a development environment, though:
<?php
// Fish class stuff here
// Beginning Trout class stuff here
public function getTroutInfo() {
$output = getInfo($this->common_name, $this->flavor, $this->record);
$output .= " This is a {$this->species} trout.";
return $output;
}
Ted Sumner
Courses Plus Student 17,967 PointsTed Sumner
Courses Plus Student 17,967 Pointsedited to add <?php for syntax highlighting.
This is a good point, but I am still not sure you need the parent::. Since is Trout is an extension of Fish, getInfo from Fish is available. You can also solve the local variable issue by defining $output as a public or protected variable in Fish.
This approach is beyond anything I have done. I am just trying to make educated guesses, so I could easily be completely wrong.
Seth Kroger
56,416 PointsSeth Kroger
56,416 PointsFWIW I checked the code and it does pass the challenge. I'm fairly sure parent:: is necessary to specify which class to use the getInfo from. Having a child class call the parent's version of an overridden method then perform actions specific to the child is a common enough pattern in OOP.
Ted Sumner
Courses Plus Student 17,967 PointsTed Sumner
Courses Plus Student 17,967 PointsThat makes sense when the method has the same name. I wonder why you would use the variable names like you have them when you have already used a constructor. I would have thought you would use $this->name etc.