Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial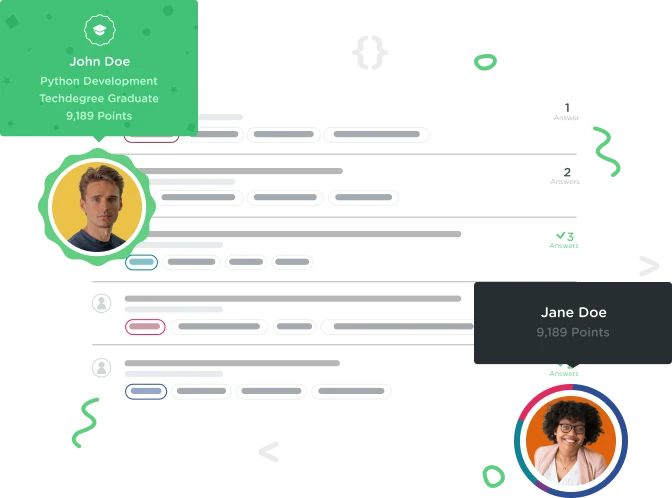
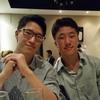
David Choi
8,536 PointsPHP - Logic of POST and GET
I have been practicing the skills I learned in the course "Build a Basic PHP Website" by Alena Holligan. Let's have some context first.
In the project "Build a Basic PHP Website", Alena uses POST to get form data and GET to redirect the page. Alena processes some data before the header of the HTML is included like so:
$error_message = array();
if ($name == "" || $email == "" || $category == "" || $title == "") {
$error_message[] = "Please fill in the required fields: Name, Email, Category, Title";
}
Then redirects the page by using the header function and setting a variable in GET.
```php
if($mail->send()) {
header("location:suggest.php?status=thanks");
exit;
}
The error message is displayed after the redirect by:
if (isset($_GET["status"]) && $_GET["status"] == "thanks") {
echo "<p>Thanks for the email! I’ll check out your suggestion shortly!</p>";
}
However, when I tried to do a similar thing with my own code (which I wrote very quickly, please ignore the bad formatting :p) :
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$test = $_POST["test"];
header("location:test.php?status=done");
exit;
} ?>
<html>
<head>
</head>
<body>
<form action="test.php" method="POST">
<?php if (isset($_GET["status"]) && $_GET["status"] == "done") {
echo $test;
} ?>
<input type="text" name="test" id="test">
<input type="submit">
</form>
</body>
</html>
The variable $test is not set after the redirect. Why does this happen?
1 Answer

Zachary Billingsley
6,187 PointsHello!
Using a redirect header will not carry over your POST variables. The logic of what you are doing is as follows ->
- test.php page loads and there are no POST variables set until you submit the form.
- After submitting the form, you reload test.php with the new POST variables (whatever you put into the 'test' field).
- Since you are using the "REQUEST METHOD" == "POST" the script enters that IF statement.
- Inside the IF statement you redirect and exit which is not a POST.
- test.php is reloaded again with your new GET variables but none of your POST variables.
- You then SKIP the IF statement because it's not a POST (So $test will never get set).
- Then your form is created, GET is set and is equal to 'done' but you should get an error because $test doesn't exist.
So if you get rid of the HEADER() and the EXIT, then change your form like this... <form action="test.php?status=done" method="POST">
You should get your desired result.
Hope that helps!
David Choi
8,536 PointsDavid Choi
8,536 PointsThanks for your answer! That really cleared things up!