Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial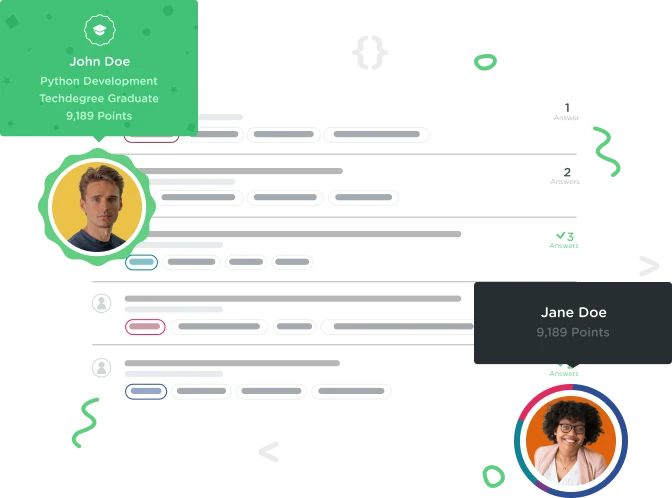

Vijay Ramdass
13,275 PointsPHP - Modifying Arrays Quiz (Question 2)
Without setting the entire array directly, change the element value of "Red" to "Magenta" and the element value of "Blue" to "Cyan".
I am getting this error: Bummer! The array does not contain the correct elements.
I did a var_dump and everything seems to be in order. Here is my code:
<?php
$colors = array("Red","Green","Blue");
//add modifications below this line
array_unshift($colors, "Yellow"); array_push($colors, "Black");
array_shift($colors); $colors[0] = "Magenta";
array_pop($colors); $colors[2] = "Cyan";
var_dump($colors);
11 Answers
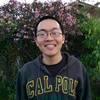
Samuel Jih
8,344 PointsGot it! It's because we added Yellow and Black, so now we need to target index 1 and 3! :)

Waldo Alvarado
16,322 PointsRemember, the index value of Red and Blue increased by one because you added Yellow to the beginning of the array.
$colors = array("Red","Green","Blue");
//add modifications below this line
array_unshift($colors, "Yellow");
array_push($colors, "Black");
$colors[1] = "Magenta";
$colors[3] = "Cyan";

Joy Manuel
6,464 PointsThis is starting to make sense. I was going as far as looking up splicing. Just need to remind myself to keep it simple.

Vijay Ramdass
13,275 PointsThank you for your reply. The unshift and push at the beginning for colors Black, and Yellow.
That is the answer I originally had, however with that I am getting this error:
Bummer! Change the element with the value "Red" to the value "Magenta"

Jeff Reeve4
8,535 PointsI'm on to the third part of the question where we need to remove the value green. I think unset($colors, 2); should do this but I keep getting the following error.
Oops! It looks like Task 1 is no longer passing

Vijay Ramdass
13,275 PointsClose! Try doing it this way:
Unset($array[key]);
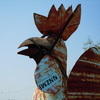
Will Anderson
28,683 PointsHere's my code that passes step 1 and 2. The 2 '$colors' lines are step 2. Note that the index has changed as we did add an element to the top in step 1. <?php
$colors = array("Red","Green","Blue"); //add modifications below this line array_unshift($colors, "Yellow"); array_push($colors, "Black"); $colors[1] = "Magenta"; $colors[3] = "Cyan";
?>

Jonathan Morton
7,942 Points$colors[1]="Magenta"; $colors[3]="Cyan";

Christian Andersson
8,712 PointsNot sure why you're doing all these shifts and unshifts, but here's the easiest way to do this:
$colors = array("Red","Green","Blue");
//add modifications below this line
$colors[0] = "Magenta";
$colors[2] = "Cyan";
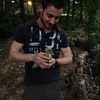
John Ireland
6,585 PointsThe first challenge has you adding things to the array, therefore changing the index. if you change that to
$colors[1] = 'Magenta'; $colors[3] = 'Cyan';
.....you're good to go.
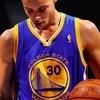
Bruce Migeri
2,675 PointsWell we need to be aware that the index values for "Red" has shifted from 0 to 1 and that of "Blue" from 2 to 3. This is because we have attached string "Yellow" to the front, index 0.

Jeff Reeve4
8,535 PointsThanks Vijay - schoolboy error that one!

Matthew Costigan
18,319 PointsHelp!
<?php
$colors = array("Red","Green","Blue"); array_push($colors, "Black"); array_unshift($colors, "Yellow");
//add modifications below this line $colors[0] = "Magenta"; $colors[2] = "Cyan";
Can't understand why this is not passing ! and getting the error Bummer! Change the element with the value "Red" to the value "Magenta"

Anne Donald
9,847 PointsThank you, I was struggling with this!
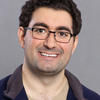
Jacob Khan
12,273 Points<?php
$colors = array("Red","Green","Blue");
//add modifications below this line
array_unshift($colors, 'Yellow');
array_push($colors, 'Black');
$colors[1] = "Magenta";
$colors[3] = "Cyan";
var_dump($colors);

Alan Sebastian
5,666 Points$colors[1] = "Magenta"; $colors[3] = "Cyan";

Masango Dewheretsoko
19,661 PointsHie Vjay Ramdas, here is the correct answer for both challenge 1 and 2 from Masango Dewheretsoko
<?php
$colors = array("Red","Green","Blue");
//add modifications below this line
array_unshift($colors,'Yellow'); array_push($colors,'Black'); $colors[1] = "Magenta"; $colors[3] = "Cyan"; ?>
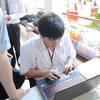
natapol chanpradab
3,190 Points$colors = array("Red","Green","Blue"); array_push($colors,'Black'); array_unshift($colors,'Yellow');
$replacements = array(1 => "Magenta", 3 => "Cyan"); $colors = array_replace($colors, $replacements);
Vijay Ramdass
13,275 PointsVijay Ramdass
13,275 PointsThank you!