Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial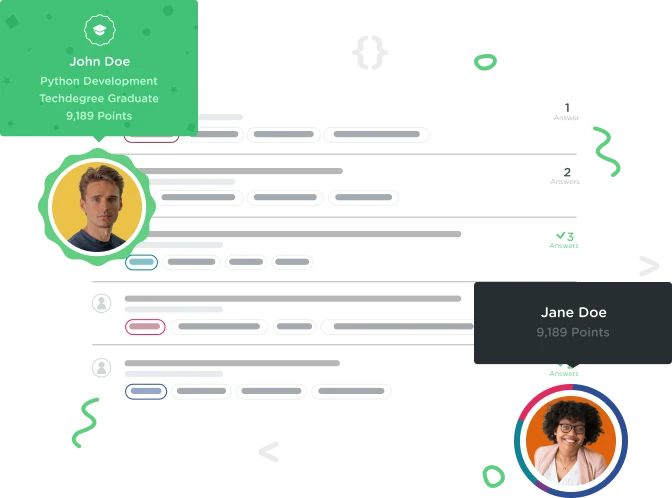

Keith Colleluori
9,577 PointsPHP OOP, Need help understanding or answering code challenge
I am having trouble with the 3rd of 3 on a PHP OOP coding challenge.
The question is as follows:
Return an array containing the public lot parameter of each house object whose public roof_color OR wall_color match the passed color parameter.
Perhaps I am having trouble trying to visualize how to get this done as an object, or perhaps I just don't know how to do it.
The leftover from the first two tasks is:
<?php
class Subdivision {
public $houses = array();
public function house($houses){
}
public function filterHouseColor($color){
}
}
?>
I have tried adding something like the following, but frankly I just don't feel like I understand what the question is asking from me and I'm tired of taking random shots at it!! Either help with the code or help understanding the question would be most appreciated.
$lots = array();
foreach ($this->houses as $house) {
if (in_array(strtolower($color)) {
$lot[]=$lots;
}
return $lot;
2 Answers
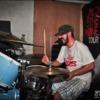
Mike Costa
Courses Plus Student 26,362 PointsSo the instructions of this challenge is as follows:
"Return an array containing the public lot parameter of each house object whose public roof_color OR wall_color match the passed color parameter."
You already have the skeleton of the challenge set up and based off of what we know from the instructions, we can add some comments to help guide us on the way to a solution.
<?php
class Subdivision {
public $houses = []; // an array to store house objects
public function filterHouseColor($color){
// return an array containing the public "lot" parameter of each "house object"
// "house object" has a "lot", "roof_color" and "wall_color"
}
}
?>
Based off of these hints from the question, we can then piece together this solution.
<?php
class Subdivision {
public $houses = [];
public function filterHouseColor($color){
// this will store the array of houses that match the criteria.
$houses_that_match = [];
// we know that "this" class has an array of "house objects", and array can be looped though, so let's loop through them
foreach($this->houses as $house){
// We need a condition to check if the color matches a houses "wall_color" or "roof_color"
if($house->roof_color == $color || $house->wall_color == $color){
// if we find a match, store this current house's "lot" in the array we created so we can return it
$houses_that_match[] = $house->lot;
}
}
return $houses_that_match;
}
}
?>
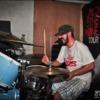
Mike Costa
Courses Plus Student 26,362 PointsFrom the question, "the public lot parameter of each house object whose public roof_color OR wall_color " it tells you that the house object has roof_color, lot and wall_color properties. From that sentence, you can assume the house object at a bare minimum is constructed like so:
<?php
class House {
public $lot;
public $roof_color;
public $wall_color;
}
When you need to store the "lot" parameter of the house object in an array if a certain condition matches, you are accessing the properties of the object it is attached to. So Subdivision has an array of "house objects", so you can think of it like so:
// for the sake of this example, lets give the house class a construct method
class House {
public $lot;
public $roof_color;
public $wall_color;
public function __construct($wall_color, $roof_color, $lot){
$this->wall_color = $wall_color;
$this->roof_color = $roof_color;
$this->lot = $lot;
}
}
$subdivision1 = new Subdivision();
$subdivision1->houses[] = new House('white', 'red', 42);
$subdivision1->houses[] = new House('blue', 'red', 38);
$subdivision2 = new Subdivision();
$subdivision2->houses[] = new House('gold', 'white', 52);
$subdivision2->houses[] = new House('black', 'yellow', 78);
The subdivision "houses" property now has 2 house objects stored in its array. For the filterHouseColor method, you're passing in a color, and then looping through the $houses attached to this specific subdivision.
// also for the sake of this example, I'm not storing the results of this function in a variable for later access
// subdivision 1
$subdivision1->filterHouseColor('white'); // returns the array [42]
$subdivision1->filterHouseColor('red'); // returns the array [42, 38]
$subdivision1->filterHouseColor('blue'); // returns the array [38]
// subdivision 2
$subdivision2->filterHouseColor('white'); // returns the array [52]
Keith Colleluori
9,577 PointsKeith Colleluori
9,577 PointsRight off the bat I am seeing I had an unnecessary house method.... That in itself probably contributed to my confusion.
Additionally I think what threw me regarding this exercise is the reference to the elements which are otherwise undeclared (lot, wall_color, and roof_color).
Most of it makes sense to me but I still don't know that I understand:
$houses_that_match[] = $house->lot;
Where is this "lot" coming from? I mean yea its in the question, but I am still having a hard time understanding how I would arrive at that line, even after seeing it as the correct solution.
I figured at some point I would be checking roof_color and wall_color against $color, so I can understand that part much easier.