Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial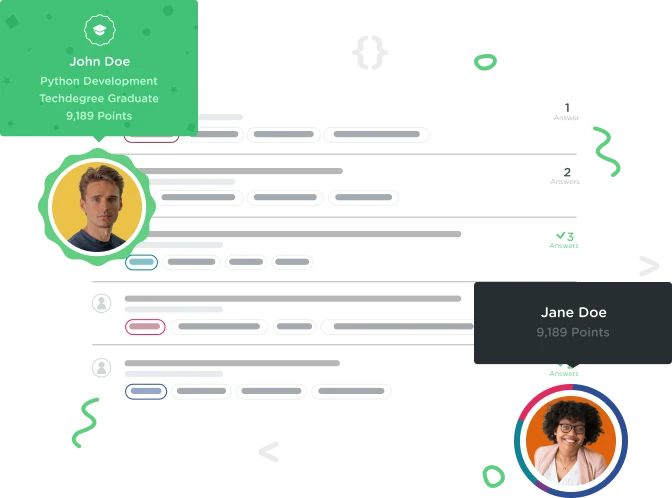

John Nixon
17,690 PointsPHP opinion
I've been building wordpress sites for a while and I'm trying to get more advanced and proficient with my php. I've written a simple little file that checks the variables of some social media options. What I want to know is if what I have is good or if there is a better way of getting my list of social media links.
<?php
$facebook = of_get_option('facebook');
$twitter = of_get_option('twitter');
$youtube = of_get_option('youtube');
$linkedIn = of_get_option('linkedin');
$instagram = of_get_option('instagram');
$socialMediaLinks = array(
);
if (!empty($facebook)){
$socialMediaLinks[facebook] = $facebook;
}
if (!empty($twitter)){
$socialMediaLinks[twitter] = $twitter;
}
if (!empty($youtube)){
$socialMediaLinks[youtube] = $youtube;
}
if (!empty($linkedIn)){
$socialMediaLinks[linkedIn] = $linkedIn;
}
if (!empty($instagram)){
$socialMediaLinks[instagram] = $instagram;
}
function socialMediaList($value, $key){
echo '<li class="'.$key.'"><a href="'.$value.'">'.$key.'</a></li>';
}
?>
<?php if (!empty($socialMediaLinks)){ ?>
<ul class="social-media">
<?php array_walk($socialMediaLinks, 'socialMediaList'); ?>
</ul>
<?php } ?>
'''
2 Answers

miguelcastro2
Courses Plus Student 6,573 PointsLooks good!
When I see a common pattern in my code that is usually a good sign I can refactor the code and optimize it by following the DRY principle. I might do something like this:
<?php
$socialMediaOpts = array('facebook', 'twitter', 'youtube', 'linkedin', 'instagram');
$socialMediaLinks = array();
foreach ($socialMediaOpts as $option) {
$linkArr = of_get_option($option);
if (!empty($linkArr)) {
$socialMediaLinks[$option] = $linkArr;
}
}
function socialMediaList($value, $key){
echo '<li class="'.$key.'"><a href="'.$value.'">'.$key.'</a></li>';
}
?>
<?php array_walk($socialMediaLinks, 'socialMediaList'); ?>

John Nixon
17,690 PointsSo I've found another php built in function in searching through this. array_filter(). Here is where i have the code at this point. Not sure if it would be better to continue using the function or just at this point switch it to a foreach loop.
<?php
$socialMediaLinks = array_filter(array(
'facebook' => of_get_option('facebook'),
'twitter' => of_get_option('twitter'),
'youtube' => of_get_option('youtube'),
'linkedIn' => of_get_option('linkedin'),
'instagram' => of_get_option('instagram')
));
function socialMediaList($value, $key){
echo '<li class="'.$key.'"><a href="'.$value.'" target="_blank">'.$key.'</a></li>';
}
?>
<?php if (!empty($socialMediaLinks)){ ?>
<ul class="social-media">
<?php array_walk($socialMediaLinks, 'socialMediaList'); ?>
</ul>
<?php } ?>